Sprites
Sprites are the objects on the screen, like player, enemy, and food! This lesson will show how to add and move sprites on the screen.
Prerequisite
You must have basic understanding of coding and have completed the Drawing lesson.
Drawing
A sprite is just an image placed at a specific location on the screen. We later need to add user control and handle collisions. The Drawing lesson is a prerequisite and already covers this section.
The first piece is the artwork of the alien. Then the second part converts the art to an image. We will set the scale here to 2 horizontal and 2 vertical. We want a decent size alien!
var alienArt =
" X X " +
" X X X" +
" XXXXXX X" +
" XXXXXXXXX" +
"XXX XX XXX" +
"X XXXXXX " +
"X X X " +
" X X ";
var alien = CreateImage(10, 8, alienArt, 2, 2, 0);
Image(alien, 0, 0);
Show();
alienArt = (
" X X " +
" X X X" +
" XXXXXX X" +
" XXXXXXXXX" +
"XXX XX XXX" +
"X XXXXXX " +
"X X X " +
" X X " )
alien = CreateImage(10,8, alienArt,2,2,0);
Image(alien, 0, 0)
Show()
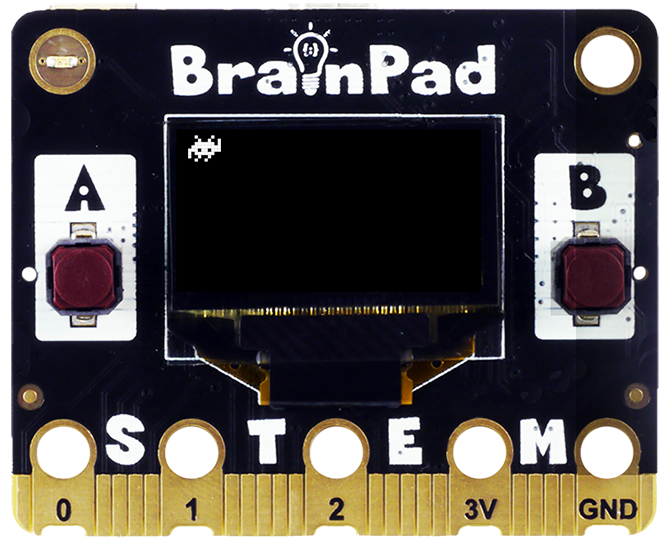
To simplify things for later, we will create a function that draws the alien at a specific location.
var alienArt =
" X X " +
" X X X" +
" XXXXXX X" +
" XXXXXXXXX" +
"XXX XX XXX" +
"X XXXXXX " +
"X X X " +
" X X ";
var alien = CreateImage(10, 8, alienArt, 2, 2, 0);
void DrawAlien(int x, int y) {
Image(alien, x, y);
}
DrawAlien(10, 10);
Show();
alienArt = (
" X X " +
" X X X" +
" XXXXXX X" +
" XXXXXXXXX" +
"XXX XX XXX" +
"X XXXXXX " +
"X X X " +
" X X " )
alien = CreateImage(10,8, alienArt,2,2,0);
def DrawAlien(x, y):
Image(alien, x, y)
DrawAlien(10,10)
Show()
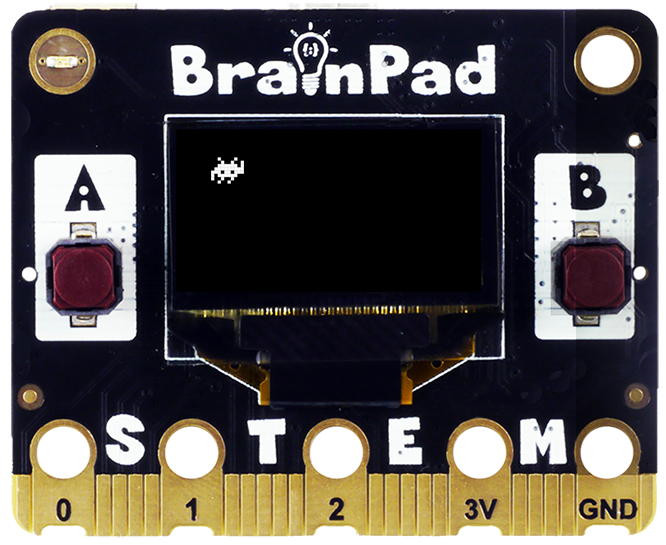
Going forward, anytime you need an alien on the display, you just need to call DrawAlien(). You an actually call it multiple times to have multiple aliens.
DrawAlien(10, 10);
DrawAlien(70, 10);
Show();
DrawAlien(10,10)
DrawAlien(70,10)
Show()
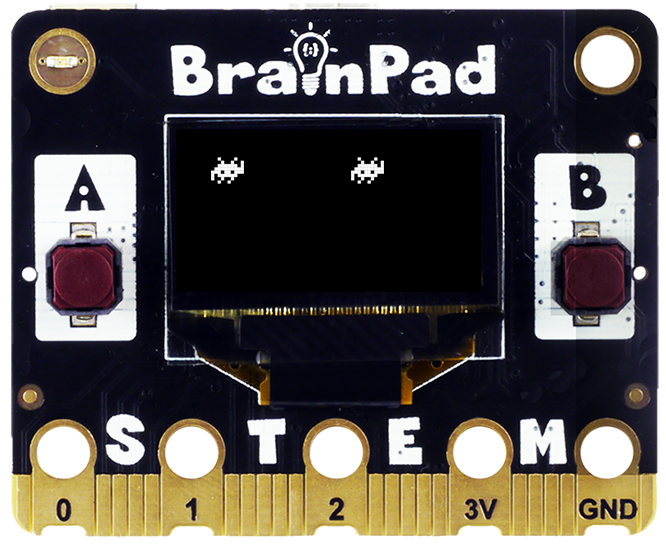
Animate
Let’s focus on the one alien but we want to make it dance. The alien can be animated by creating 2 frames that change the alien’s movement. Our specific art has an alien with one arm up and the second down. Instead of making another piece of art to invert the look, we will use the last argument in the CreateImage() which sets the transform. Looking at the Drawing lesson we can see that flip horizontal is 1.
We will call the 2 images alienLeft and alienRight. Notice the 1 in the last argument.
var alienLeft = CreateImage(10, 8, alienArt, 2, 2, 0);
var alienRight = CreateImage(10, 8, alienArt, 2, 2, 1);
alienLeft = CreateImage(10, 8, alienArt, 2, 2, 0)
alienRight = CreateImage(10, 8, alienArt, 2, 2, 1)
Now, the DrawAlien() function should draw the next frame every time it is called. If we have many frames, we would have a counter to keep track of the frames. In this beginner lesson we will have only 2 and we will do either or. To accomplish this, we will use isLeft variable to remind the DrawAlien() function of which image to draw, left or right.
var isLeft = true;
void DrawAlien(int x, int y) {
if (isLeft) {
Image(alienLeft, x, y);
isLeft = false;
}
else {
Image(alienRight, x, y);
isLeft = true;
}
}
isLeft = true
def DrawAlien(x, y):
if isLeft:
Image(alienLeft, x, y)
isLeft = False
else:
Image(alienRight, x, y)
isLeft = True
We can now start making our game loop. The first thing is clear the screen. Then draw the alien and Show() it. We will add a small delay of 0.03sec (30ms).
while (true)
{
Clear();
DrawAlien(10, 10);
Show();
Wait(0.03);
}
add
Ok that was cool! But lets make it even more fun and make the alien climb down the screen. We want the alien to start above the screen. The top of the screen is 0 so lets start the alien at -30. We also want the alien to go beyond the end of the screen. We will make it go all the way to 70. Then whenever it reaches 70 we would send it back to -30.
var alienY = -30;
while (true)
{
Clear();
DrawAlien(10, alienY);
Show();
alienY = alienY + 10;
if (alienY > 70)
{
alienY = -30;
}
Wait(0.03);
}
add
This is the complete code for entire program.
using static BrainPad.Controller;
using static BrainPad.Display;
var alienArt =
" X X " +
" X X X" +
" XXXXXX X" +
" XXXXXXXXX" +
"XXX XX XXX" +
"X XXXXXX " +
"X X X " +
" X X ";
var alienLeft = CreateImage(10, 8, alienArt, 2, 2, 0);
var alienRight = CreateImage(10, 8, alienArt, 2, 2, 1);
var isLeft = true;
void DrawAlien(int x, int y)
{
if (isLeft){
Image(alienLeft, x, y);
isLeft = false;
}
else{
Image(alienRight, x, y);
isLeft = true;
}
}
var alienY = -30;
while (true){
Clear();
DrawAlien(10, alienY);
Show();
alienY = alienY + 10;
if (alienY > 70){
alienY = -30;
}
Wait(0.03);
}
add
What is Next?
Sprites have moved on their own but we now want to move them using buttons, using the Controller.
BrainStorm
Artificial Intelligence is coding something to make it seem like it is thinking. How can we make our alien smarter? Can it climb down from a different location? If we use a random number to decide where the alien will come down we can make it seem like the the alien is doing something different.
Here is the game loop:
var alienY = -30;
var rnd = new System.Random();
var alienX = rnd.Next(100);
while (true)
{
Clear();
DrawAlien(alienX, alienY);
Show();
alienY = alienY + 10;
if (alienY > 70)
{
alienY = -30;
alienX = rnd.Next(100);
}
Wait(0.03);
}
add