This page uses MicroPython, which is no longer supported, consider using the official Python instead.
Python System Setup
This lesson will show you how to setup your machine and the BrainPad to work with MicroPython.
Prerequisite
This lesson is the very starting point. You only need a computer and a BrainPad! If your computer and BrainPad are already setup, skip this lesson and go to Python Intro Lesson.
BrainPad Setup
We now need to load the BrainPad with MicroPython firmware. If you have multiple BrainPads, you will need to perform this setup for each one, but only once.
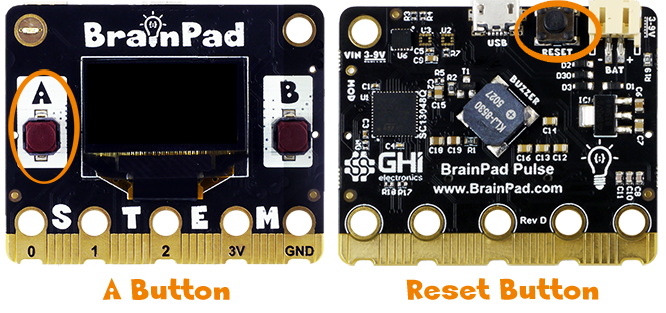
- Put the BrainPad into LOADER MODE: Connect your BrainPad to the computer using the included USB cable. To put the BrainPad into LOADER MODE, press and hold the A button, then press and release RESET while still holding the A button. You will hear a sound from your PC of the BrainPad reconnecting but you will not see anything on teh screen. The BrainPad is now in LOADER MODE.
- Visit the loader website: https://loader.brainpad.com/
- Install Firmware: Follow the steps on the loader website.
You have just completed the System Setup!
After successfully loading the firmware, the BrainPad Pulse will display the following screen. On the BrainPad Tick, the word Python will scroll across the LEDs. If the screen is still blank, try hitting only the Reset button.
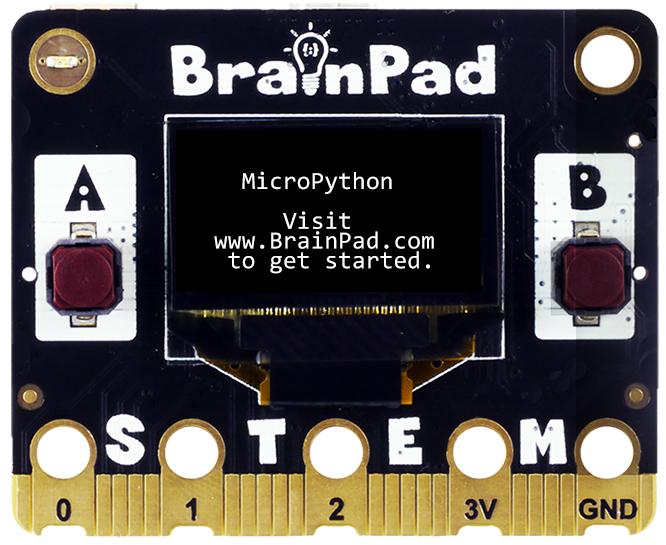
Prepping the Editor
We have chosen Thonny as an editor for coding BrainPad using MicroPython. While this maybe optional, we highly recommend it, and the lessons assume an editor is installed. Go ahead and install Thonny editor, which is available for Window, Mac and Linux. Using Chromebook should be possible, but that is beyond this lesson and not officially supported.
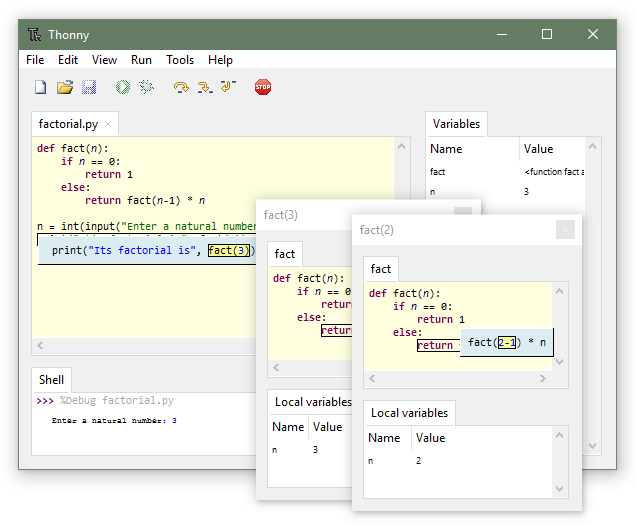
When installation is done, go ahead and start Thonny.
We now need to direct the editor to what COM port the BrainPad uses. First plug in the BrainPad that is running MicroPython. This was covered earlier. Then, from the top menu Run ->Select Interpreter…
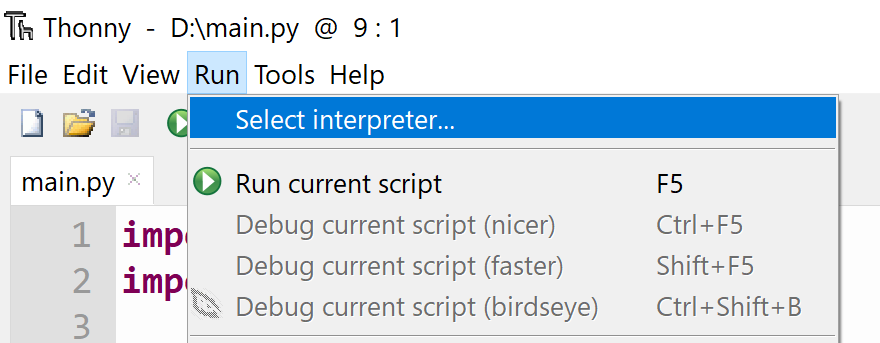
From here, select MicroPython (generic) interpreter. This will now show an option on the same window to select the Port. You need to select the correct USB Serial device COM port number that corresponds to your device. You will likely have only one to select from. If you have more than one, you can disconnect the BrainPad and see which COM port disappears.
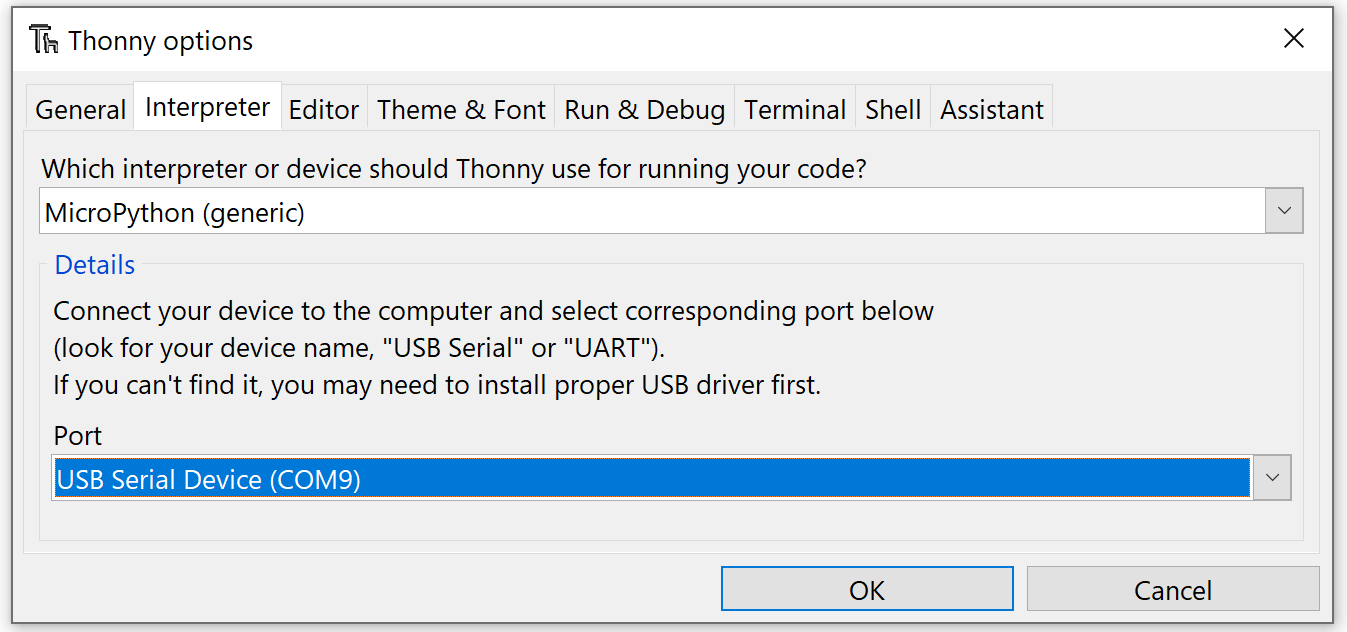
Loading Hello World
Every Python program you create MUST HAVE from BrainPad import * on the top line. Lessons going forward won’t include this line, so just remember it has to be there.
Now let’s print something on the screen. Try to copy/paste the code below instead of typing it.
from BrainPad import *
Print("Hello World")
We can now click the GREEN Arrow Run icon or hit the F5 key. Since this is a new program, we’ll be prompted to save the file.
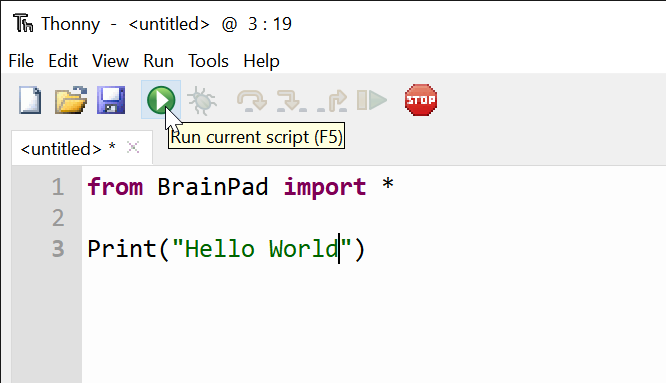
Make sure you select This computer when saving the file and NOT the device itself.
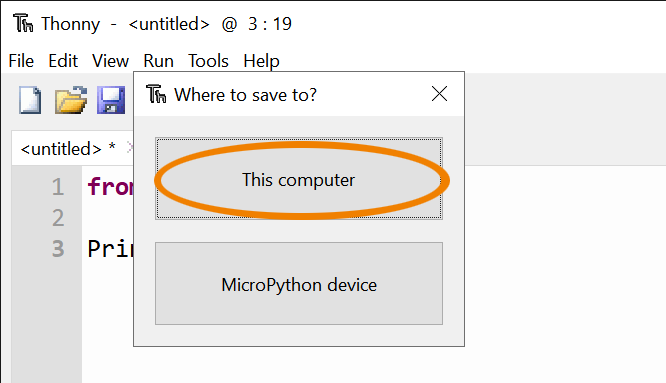
Navigate to somewhere on your computer, like Desktop or any folder you created to hold your MicroPython programs. You can name the file anything you like, then click and Save.
This will send the script to the device and execute the code, which will show Hello World on the screen.
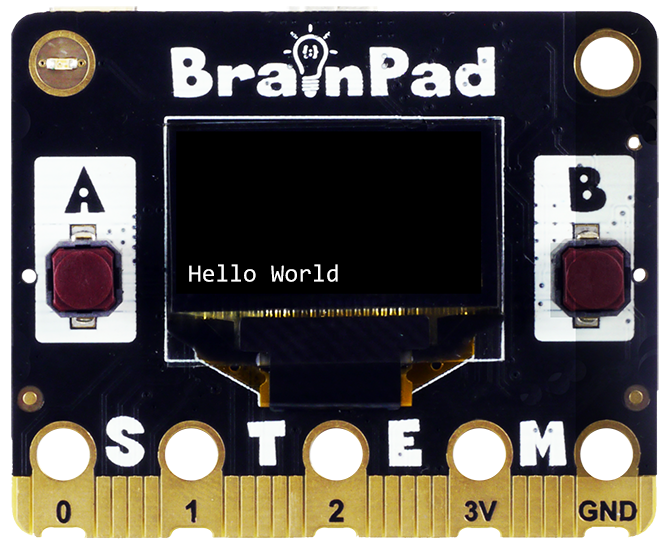
Your program got loaded onto the device using the cable. Keep on eye on the messages you see in the “Shell” window.

A program syntax error (typed wrong) or a program transfer error will show in this window. A transfer error can happen often and it is solved by clicking Run again. If Run is greyed out, then you can click Stop first and then run.
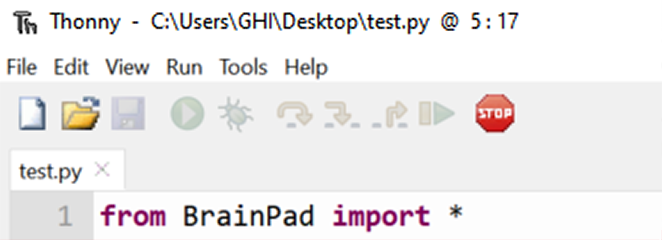
Note that sometimes you may build a program that runs forever. In this case, the program will not finish and you must click Stop. Here is a program that runs forever. You do not need to understand it yet, just try it for now.
from BrainPad import *
count = 0
while True:
count = count + 1
Print(count)
Persisting Programs
Time to save your program on BrainPad! Note that the program loaded will only run the one time you run it. If you reset the board, the program will not be there. Fear not, there is a way to keep (persist) the program onto the BrainPad.
If you want to bring back the BrainPad to its original state, you can reload it with the firmware just like you did in the BrainPad Setup section earlier.
When the BrainPad that is loaded with MicroPython is connected to a computer, the computer will detect a COM port that we used to load our program. It will also load a memory driver, just like a USB memory stick. In that memory stick, there is a file called main.py. This is the file that will automatically run when the the board powers up.
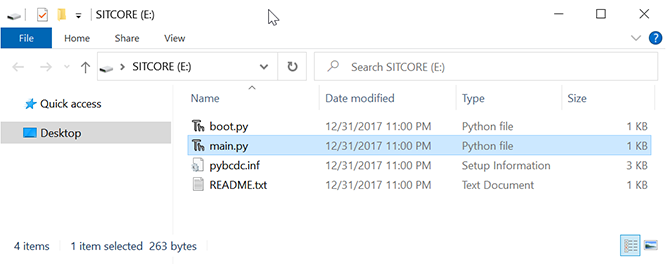
Open the Thonny editor and click file open. The location of the main.py file should open. If it doesn’t, then navigate to it’s location. Remember it acts just like a USB memory stick.
Once open, you’ll see the code that is currently saved on the BrainPad. Next, delete all the code EXCEPT from BrainPad import *. Remember we ALWAYS need this line.
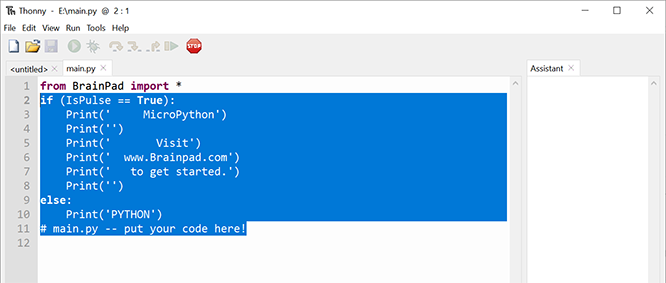
Now, add the new code and click run.
Print("This runs")
Print("Even when reset")
It is important to note that an activity LED will turn on when saving to a file on the internal BrainPad storage (the main.py file). Do NOT reset or disconnect the BrainPad when the LED comes on while saving the file. The activity LED is the small LED on the front left corner on BrainPad Pulse. On BrainPad Tick, it is the top right LED on the front.
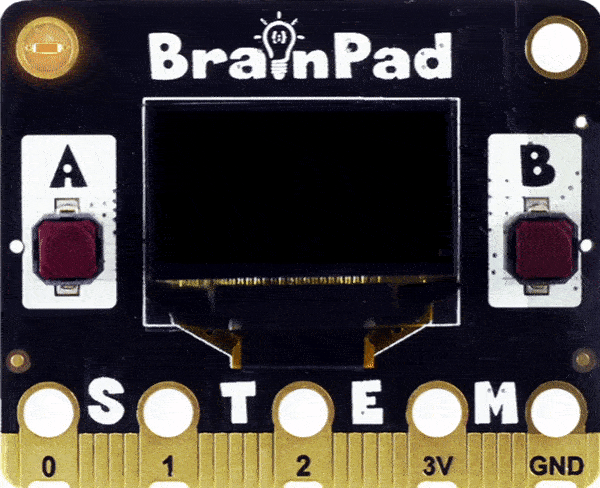
What is Next?
Now that you have a working project, let’s continue learning some more Python, or MicroPython to be exact! Check out the Python Intro Lesson to move to the next phase and start coding.