This is an old page and it is no longer supported.
Video games have been an important part of computer systems from their early days. We will start by making the simplest possible video game. This lesson will build a game that is the same as the one in the Gaming Intro lesson, but this lesson changes it to work with BrainPad Tick.
Prerequisite
You must have basic understanding of coding and have completed the Drawing lesson.
Game Objective
The player will need to catch falling eggs in a basket. Since this lesson is for the BrainPad Tick, which has a 5×5 LED matrix, the eggs are just a point (pixel) and the basket is also just a point.
Basket Drawing
The player, which is a basket in this case, is just a Point().
As a reminder, we will add the top lines that bring in the drawing libraries. Those lines will be excluded in the examples shown after.
using static BrainPad.Controller;
using static BrainPad.Display;
Point(2,3,White);
Show();
from BrainPad import *
Point(2,3,White)
Show()
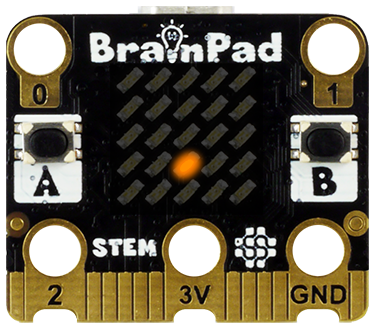
Basket Control
ButtonA and ButtonB will be used to control the player (basket). There are complete lessons on In & Out control, but for now, all you need to know is that there is a Button() element that In() can read. When a button is pressed, a 1 is returned, 0 when not pressed.
We will create a variable to keep track of the basket X position. When ButtonA is pressed, the basket is moved to the left decreasing the X position. When ButtonB is pressed the basket is moved to the right by increasing the X position.
To keep this code separate and easier to manage, we will place it in its own function.
var basketX = 2;
var btnA = Button(ButtonA, 0.1);
var btnB = Button(ButtonB, 0.1);
void ProcessBasket() {
if (In(btnA) == 1){
basketX = basketX - 1;
}
if (In(btnB) == 1) {
basketX = basketX + 1;
}
}
basketX = 2
btnA = Button(ButtonA,0.1)
btnB = Button(ButtonB,0.1)
def ProcessBasket():
global basketX
global btnA
global btnB
if In(btnA) == 1:
basketX = basketX - 1
if In(btnB) == 1:
basketX = basketX + 1
With the basket process in place, we can now create the game loop to process and draw the the “basket”.
while (true) {
Clear();
Point(basketX, 4, White);
Show();
ProcessBasket();
}
while True:
Clear()
Point(basketX, 4, White)
Show()
ProcessBasket()
Since the game is very simple and only processing a few LEDs, it will run too fast. We will add Wait(0.1) to the loop to slow it down.
while (true) {
Clear();
Point(basketX, 4, White);
Show();
ProcessBasket();
Wait(0.1);
}
while True:
Clear()
Point(basketX, 4, White)
Show()
ProcessBasket()
Wait(0.1)
The entire code listing is here in case you have missed something.
var basketX = 2;
var btnA = Button(ButtonA, 0.1);
var btnB = Button(ButtonB, 0.1);
void ProcessBasket() {
if (In(btnA) == 1) {
basketX = basketX - 1;
}
if (In(btnB) == 1) {
basketX = basketX + 1;
}
}
while (true) {
Clear();
Point(basketX, 4, White);
Show();
ProcessBasket();
Wait(0.1);
}
basketX = 2
btnA = Button(ButtonA,0.1)
btnB = Button(ButtonB,0.1)
def ProcessBasket():
global basketX
global btnA
global btnB
if In(btnA) == 1:
basketX = basketX - 1
if In(btnB) == 1:
basketX = basketX + 1
while True:
Clear()
Point(basketX, 4, White)
Show()
ProcessBasket()
Wait(0.1)
Try what we have so far. Did you notice how the basket can leave the screen if you keep pushing one of the buttons? Any ideas on how we can keep the basket from leaving the screen?
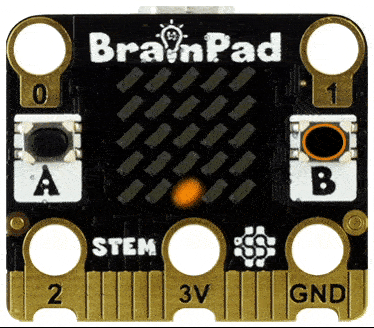
Stay on Screen
Keeping the basket on the screen is somewhat easy. We will check the basket position and only change its position if the buttons are pressed and also if the basket is not leaving the screen. Notice how we used “and” in the previous sentence. The code will also be exactly just that.
var basketX = 2;
var btnA = Button(ButtonA, 0.1);
var btnB = Button(ButtonB, 0.1);
void ProcessBasket() {
if (In(btnA) == 1 && basketX > 0) {
basketX = basketX - 1;
}
if (In(btnB) == 1 && basketX < 4) {
basketX = basketX + 1;
}
}
basketX = 2
btnA = Button(ButtonA,0.1)
btnB = Button(ButtonB,0.1)
def ProcessBasket():
global basketX
global btnA
global btnB
if In(btnA) == 1 and basketX > 0:
basketX = basketX - 1
if In(btnB) == 1 and basketX < 4:
basketX = basketX + 1
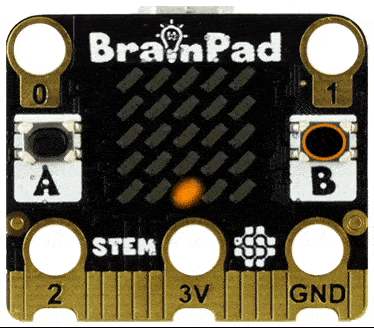
Falling Eggs
We’ll use a Point() as our egg, with its location being eggY and eggX.
var eggY = 0;
var eggX = 2;
Point(eggX, eggY, White);
eggY = 0
eggX = 2
Point(eggX, eggY, White)
The eggs will always start on top of the screen and fall down. The “down movement” means the Y axis location will increase. Remember, the top of the screen is 0. eggY variable will keep track of the ball position falling down. For the X axis position, we will use eggX variable. Let us just set it and keep it at 20 for now.
void ProcessEgg() {
eggY = eggY + 1;
}
def ProcessEgg():
global eggY
eggY = eggY + 1
Update the main loop and try the game. The “egg” will fall down and that is about it. To see it again, you need to rerun your program or hit the reset button on the back.
while (true) {
Clear();
Point(basketX, 4, White);
Circle(eggX, eggY, White);
Show();
ProcessBasket();
ProcessEgg();
Wait(0.1);
}
while True:
Clear()
Point(basketX, 4, White)
Point(eggX, eggY, White)
Show()
ProcessBasket()
ProcessEgg()
Wait(0.1)
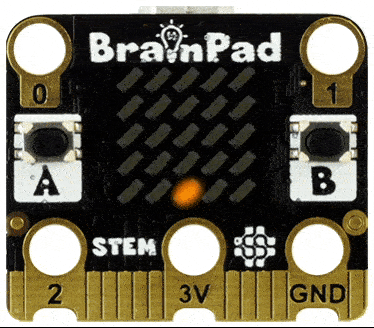
A small change is needed to send the egg back to the top. Whenever the egg’s eggY reaches the bottom, we would set its position back to the top. Note how “global” was added to the eggY variable in Python. This variable is defined outside ProcessEgg() (defined globally) and we want ProcessEgg() to use that same variable instead of using its own version.
var eggY = 0;
var eggX = 2;
void ProcessEgg() {
eggY = eggY + 1;
if (eggY > 4) {
eggY = 0;
}
}
eggY = 0
eggX = 2
def ProcessEgg():
global eggY
eggY = eggY + 1
if eggY>4:
eggY = 0
If you try what we have so far, you will notice that the egg is falling too quickly. We can slow things down by increasing our Wait(0.1), but that is already at 100ms, meaning at 10 frames per second. We do not want that to be slower. To slow down the egg without slowing down the game, we would increase eggY by a number smaller than 1, say 0.25 to make it 4 times slower. Doing so will cause an error in C# because the language expected an integer (not fractions), but then we have it 0.25. We need to specify that the variable eggY is a double. This can be done by changing var to double to just use 0.0 instead of 0.
var eggY = 0.0;
var eggX = 20;
void ProcessEgg() {
eggY = eggY + 0.25;
if (eggY > 4) {
eggY = 0;
}
}
eggY = 0.0
eggX = 20
def ProcessEgg():
global eggY
eggY = eggY + 0.25
if eggY > 4:
eggY = 0
Now, we can try it and see if the results are what we need.
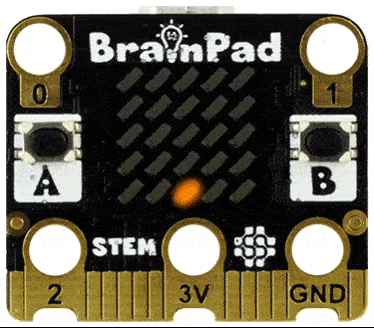
That is starting to look like a game! We now need to change where the egg falls down from. We want it coming down from a random location. Thankfully, the random is a built-in feature in most programming languages. If you’re not sure how random works, there is more information inside the Built-in functions lesson. When using Python, don’t forget to add the line ‘import random’ at the top of our code.
var eggY = 0.0;
var eggX = 2;
var rnd = new System.Random();
void ProcessEgg() {
eggY = eggY + 0.25;
if (eggY > 4) {
eggY = 0;
eggX = rnd.Next(4);
}
}
import random
eggY = 0.0
eggX = 20
def ProcessEgg():
global eggY
eggY = eggY + 0.25
if eggY > 4:
eggY = 0
eggX = random.randint(0,4)
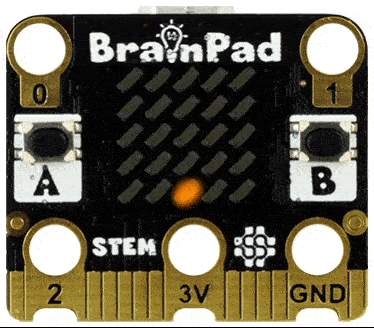
Keeping Score
The basket is moving and the egg is falling, but where is the score? This is done by comparing the position of the egg to the basket. We only need to do this when the egg reaches the bottom. If the egg is within the basket, we will increase the score by one.
var eggY = 0.0;
var eggX = 2;
var rnd = new System.Random();
var score = 0;
void ProcessEgg()
{
eggY = eggY + 0.25;
if (eggY > 4)
{
if(eggX == basketX)
{
score = score + 1;
}
eggY = 0;
eggX = rnd.Next(4);
}
}
eggY = 0
eggX = 2
score = 0
def ProcessEgg():
global eggY
global eggX
global basketX
global score
eggY = eggY + 0.25;
if eggY > 4:
if eggX == basketX:
score = score + 1
eggY = 0
eggX = random.randint(0,4)
We finally can show off our score! We will take over the entire screen (LEDs), show the score for one second, then go back to playing the game. If there is a miss, we will show X on the LEDs.
var eggY = 0.0;
var eggX = 2;
var rnd = new System.Random();
var score = 0;
void ProcessEgg() {
eggY = eggY + 0.25;
if (eggY > 4) {
if (eggX == basketX) {
score = score + 1;
Clear();
Text(score, 0, 0);
Show();
Wait(1);
}
else {
Clear();
Text("X", 0, 0);
Show();
Wait(1);
}
eggY = 0;
eggX = rnd.Next(4);
}
}
eggY = 0
eggX = 2
score = 0
def ProcessEgg():
global eggY
global eggX
global basketX
global score
eggY = eggY + 0.25
if eggY > 4:
if eggX == basketX:
score = score + 1
Clear()
Text(score,0,0)
Show()
Wait(1)
else:
Clear()
Text("X", 0, 0)
Show()
Wait(1)
eggY = 0
eggX = random.randint(0,4)
Final Results
A playable game with under 50 lines of code! Not bad considering commercial games are usually thousands or millions of lines of code! Here is the complete code with all the parts we’ve shown above:
using static BrainPad.Controller;
using static BrainPad.Display;
var basketX = 2;
var btnA = Button(ButtonA, 0.1);
var btnB = Button(ButtonB, 0.1);
void ProcessBasket() {
if (In(btnA) == 1 && basketX > 0) {
basketX = basketX - 1;
}
if (In(btnB) == 1 && basketX < 4) {
basketX = basketX + 1;
}
}
var eggY = 0.0;
var eggX = 2;
var rnd = new System.Random();
var score = 0;
void ProcessEgg() {
eggY = eggY + 0.25;
if (eggY > 4) {
if (eggX == basketX) {
score = score + 1;
Clear();
Text(score, 0, 0);
Show();
Wait(1);
}
else {
Clear();
Text("X", 0, 0);
Show();
Wait(1);
}
eggY = 0;
eggX = rnd.Next(4);
}
}
while (true) {
Clear();
Point(basketX, 4, White) ;
Point(eggX,eggY,White) ;
Show();
ProcessBasket();
ProcessEgg();
Wait(0.1);
}
from BrainPad import *
import random
basketX = 2
btnA = Button(ButtonA,0.1)
btnB = Button(ButtonB,0.1)
def ProcessBasket():
global basketX
global btnA
global btnB
if In(btnA) == 1 and basketX > 0:
basketX = basketX - 1
if In(btnB) == 1 and basketX < 4:
basketX = basketX + 1
eggY = 0
eggX = 2
score = 0
def ProcessEgg():
global eggY
global eggX
global basketX
global score
eggY = eggY + 0.25
if eggY > 4:
if eggX == basketX:
score = score + 1
Clear()
Text(score,0,0)
Show()
Wait(1)
else:
Clear()
Text("X", 0, 0)
Show()
Wait(1)
eggY = 0
eggX = random.randint(0,4)
while True:
Clear()
Point(basketX, 4, White)
Point(eggX, eggY, White)
Show()
ProcessBasket()
ProcessEgg()
Wait(0.1)
What’s Next?
Modify the code to change how the game works, or completely make a new game. Visit the Lesson Plan page to see what other lessons are there.
BrainStorm
In the early computer days, things were very simple and resources were severely limited. Game creators had to be very creative to make exciting games with limited resources. Can you think of examples that you are familiar with? Tetris? Packman? Mario?