Built-in Functions
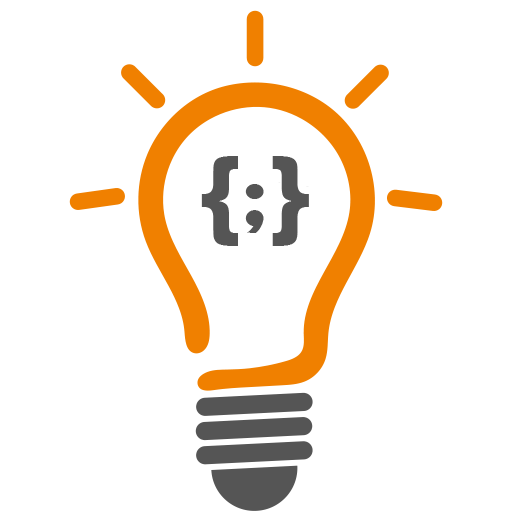
We are ready to cover some basic built-in functions to get us started. These functions are built inside both the BrainPad itself and in the programming language we’re using.
Prerequisite
This lesson assumes you’re already completed the Coding Intro lesson.
Intro
Built-in functions, sometimes referred to as API (Application Programming Interface), are functions that are pre-made and found internally in the system. Just like how we make a function for later use, someone created functions that are already found in the device that we can just use.
Device Specific
Some of these functions are provided by the specific devices’ libraries. Print() is one of them. The Print() function outputs whatever argument it is given to the screen.
Python
BrainPad.System.Println("Hello")
BrainPad.System.Println(5)
C#
BrainPad.System.Println("Hello");
BrainPad.System.Println(5);
JS
await BrainPad.System.Println("Hello");
await BrainPad.System.Println(5);
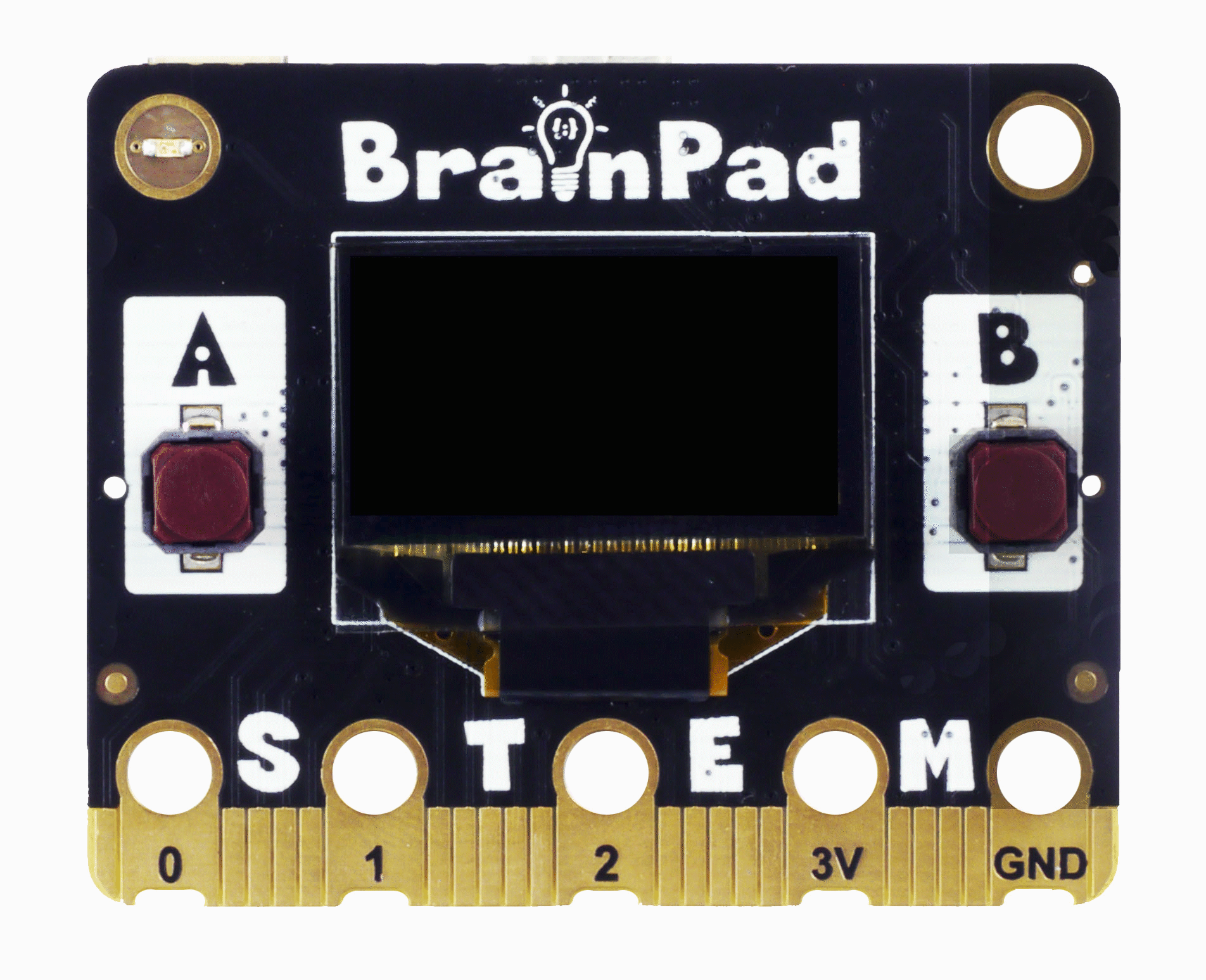
In the case of BrainPad Tick, the text will scroll across the LEDs. If the text being printed is a single character, then that one character will just show on the LEDs.
Another function is Wait(). Just as you may be thinking, Wait() forces the BrainPad to pause for some time. The following code will output 1 and then pause for one second, then output 2 and pauses for another second, and finally ends with an output of 3.
Python
BrainPad.System.Println(1)
BrainPad.System.Wait(1000)
BrainPad.System.Println(2)
BrainPad.System.Wait(1000)
BrainPad.System.Println(3)
C#
BrainPad.System.Println(1);
BrainPad.System.Wait(1000);
BrainPad.System.Println(2);
BrainPad.System.Wait(1000);
BrainPad.System.Println(3);
JS
await BrainPad.System.Println(1);
await BrainPad.System.Wait(1000);
await BrainPad.System.Println(2);
await BrainPad.System.Wait(1000);
await BrainPad.System.Println(3);
The complete list of device-specific functions is found on the Due Link website, for Python, .NET C#, and JS.
Language Built-ins
Some functions are built-in as part of the programming languages we’re using. While languages may have different definitions of those functions, they provide a similar outcome.
For example, one of these functions is a random number generator. We will show how it is used in C#, Python, and JS.
Python
import random
myRandomNumber = random.randint(0,100)
BrainPad.System.Println(myRandomNumber)
myRandomNumber = random.randint(0,100)
BrainPad.System.Println(myRandomNumber)
C#
var rnd = new System.Random();
var myRandomNumber = rnd.Next(100);
BrainPad.System.Println(myRandomNumber);
myRandomNumber = rnd.Next(100);
BrainPad.System.Println(myRandomNumber);
JS
let myRandomNumber = Math.floor(Math.random()*100);
await BrainPad.System.Println(myRandomNumber);
myRandomNumber = Math.floor(Math.random()*100);
await BrainPad.System.Println(myRandomNumber);
In C#, a Random object comes from the System namespace, needing System.Random(). Python needs an import of the random library. In JavaScript, random numbers can be generated using Math.random(). From there, the use is almost identical. C# Next() and Python randint() return the next random number.
The argument in the above example (the 100) tells the function to give us a random number that is no more than 100.
Can you use those random numbers to plot points or shapes on the screen at random locations? Just keep the shapes within the screen by limiting the random number to a max of the screen size. Don’t know how to draw yet? Well, that is next!
Python
import random
BrainPad.Display.Clear(0)
while True:
x = random.randint(0,127)
y = random.randint(0,64)
BrainPad.Display.SetPixel(1, x, y)
BrainPad.Display.Show()
C#
var rnd = new System.Random();
BrainPad.Display.Clear(0);
while(true){
var x = rnd.Next(127);
var y = rnd.Next(64);
BrainPad.Display.SetPixel(1,x, y);
BrainPad.Display.Show();
}
JS
BrainPad.Display.Clear(0);
while(true){
var x = Math.random()*127;
var y = Math.random()*64;
await BrainPad.Display.SetPixel(1,x, y);
await BrainPad.Display.Show();
}
What’s Next?
You now know enough coding and you deserve to start with some fun topics. The next step is to learn about the drawing function.
BrainStorm
Do animals and humans have built-in functions at birth? Why do some newborn animals get up as soon as they are born and start following their mom? Why does a parent is willing to risk their life to protect their children? Is this something they learned or it is built in our brains?