Python is a popular scripting language. It is well-capable and user-friendly.
.NET C# is a powerful language that stems from C, which is the mother of all languages. In the coding world, C is the global language that is the base for all other languages. Learning C# is a great learning point for anyone wanting to pursue lower-level coding or go deep into low-level devices.
JavaScript is a powerful language that stems from C and Java. It is a very popular language among web developers because all modern web browsers can run JavaScript code.
Prerequisite
This lesson assumes you have completed the system setup as shown on the main .NET C# Page, Python page or JavaScript page.
Objective
This lesson is a quick introduction to C#, Python, and JS. You can ignore the language you are not interested in learning but seeing them all is a good way to understand the differences between coding languages.
The goal at the end of this lesson is to write a program that counts from 0 to 7. And then we’ll modify the program to print 0 to 7 but skip number 4.
Case Sensitive
C#, Python, and JS are case-sensitive like most coding languages, but what does that mean?! This means the language cares greatly about how things are spelled. For example, pRint() and Print() are not the same.
Comments
A comment is a group of words or sentences we can add to our code that doesn’t affect how it runs. The computer will ignore the comment and run the code only. We use comments to tell us what’s happening in the code we’ve written or how something is done so others can understand the code we’re creating. C# and JS use // to start comments, whereas Python uses # to start a comment.
Comments can also be useful for testing different sections of code by commenting and uncommenting lines and viewing the results.
Python
# This is a comment.
# Comments are useful to remind us of what our code is doing.
# The computer will ignore comments completely.
print("Hello")
C#
// This is a comment.
// Comments are useful to remind us of what our code is doing.
// The computer will ignore comments completely.
Console.WriteLine("Hello");
JS
// This is a comment.
// Comments are useful to remind us of what our code is doing.
// The computer will ignore comments completely.
console.log("Hello");
C# and JS also support a block of comments that start with /* and end with */. We will only use // throughout the lessons.
Print Output
Going forward, we will use a special Print() PrintLn() method that does two things, print to the output window and also to the display if available.
Python
from DUELink.DUELinkController import DUELinkController
# Connect to BrainPad
availablePort = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(availablePort)
# BrainPad is ready
BrainPad.System.Println("Hello")
C#
using GHIElectronics.DUELink;
// Connect to BrainPad
var availablePort = DUELinkController.GetConnectionPort();
var BrainPad = new DUELinkController(availablePort);
// BrainPad is ready
BrainPad.System.Println("Hello");
JS
await BrainPad.System.Println("Hello");
If you are using BrainPad Edge and have a supported display connected, you can add this line to configure the system to use an external display. You need the appropriate display address. More on this in the drawing lesson.
Python
BrainPad.Display.Config(1,address)
JS
await BrainPad.Display.Config(1,address)
Going forward, we will only show the code that uses BrainPad and not the initial code that sets up BrainPad since this code will always be the same. This is the code you need to add on your own:
Python
from DUELink.DUELinkController import DUELinkController
# Connect to BrainPad
availablePort = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(availablePort)
# BrainPad is ready
C#
using GHIElectronics.DUELink;
// Connect to BrainPad
var availablePort = DUELinkController.GetConnectionPort();
var BrainPad = new DUELinkController(availablePort);
// BrainPad is ready
JS
import {SerialUSB} from './serialusb.js';
import * as due from './duelink.js';
let BrainPad = new due.DUELinkController(new SerialUSB());
await BrainPad.Connect();
The following code will assume you have added the code above.
Python
BrainPad.System.Println("Hello")
C#
BrainPad.System.Println("Hello");
JS
await BrainPad.System.Println("Hello");
Variables
A variable is a spot in the computer’s memory to help the program in memorizing something. We can give the variable any name, but the name can’t contain spaces, can’t start with a number, and can’t contain a symbol. Say we have a variable to keep track of a count and the count now is 5.
Python
count = 5
BrainPad.System.Println(count)
C#
var count = 5;
BrainPad.System.Println(count);
JS
let count = 5;
await BrainPad.System.Println(count);
Can you guess why PrintLn() showed the value of count, which is 5, instead of printing the word count?
Let’s make a program that takes two numbers and then adds them up. The two numbers will be stored in variables a and b.
Python
a = 5
b = 4
BrainPad.System.Println("The sum of a and b is:")
BrainPad.System.Println(a+b)
C#
var a = 5;
var b = 4;
BrainPad.System.Println("The sum of a and b is:");
BrainPad.System.Println(a+b);
JS
let a = 5;
let b = 4;
await BrainPad.System.Println("The sum of a and b is:");
await BrainPad.System.Println(a+b);
More on variables in other lessons.
Python Space vs Tab
Python uses indentation, or white space, to know when blocks start and end. This makes the white space at the beginning of the line very important. That white space can be 4 spaces or a tab. This is where things can get tricky. While it is okay to use either or, it is not okay to mix between spaces and tabs in the same blocks. But since a tab is considered white space and a space is also considered white space, a person looking at the code will not see the difference.
When using an editor, like Thonny, the editor will highlight tabs for the user to see better.
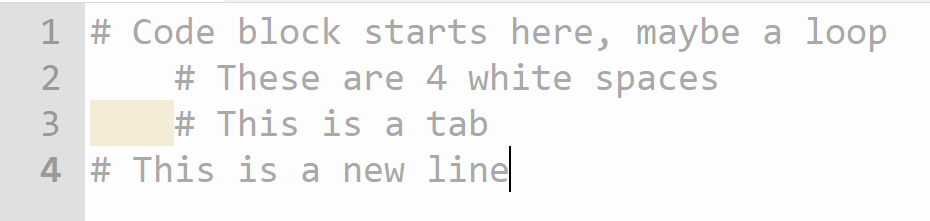
Another benefit of using an editor like Thonny is that it knows about blocks and helps with adding white space. Since using 4 spaces is recommended over using tabs, pressing the tab key on the keyboard will result in 4 spaces, not a tab in Thonny.
If you do not understand this section, just remember that you do not want to see any white space that is highlighted with darker areas. If you do, delete that “tab”; and then the next time you hit the tab key, Thonny will just add 4 spaces. You can also hit the space key 4 times!
For demonstration purposes, we will use the → symbol to show spaces. However, when you write the code, you will add a space for each → you see in the example code. The space added (indentation) before the lines inside the block is very important as this is how Python knows where blocks start and where they end. Each line with the same indentation is a continuation of the block. The last line with no indentation is the end of the block.
count = 0
while count<8:
→→→→BrainPad.System.PrintLn(count)
→→→→# Still in the block
→→→→count = count + 1
# block end
count = 0
while count<8:
BrainPad.System.PrintLn(count)
# Still in the block
count = count + 1
# block end
In summary, a block is at the indented line(s). An indent is a tab or 4 spaces.
C# White Space
White space does not mean anything to C#, making it optional. White space does make the code easier to spot though. The following two code examples are both identical and are both completely valid. Which one would you rather use?
var count = 0; while(count<
8){
BrainPad.System.Println(count
); count =
count + 1;}
var count = 0;
while(count<8){
BrainPad.System.Println(count)
count = count + 1;
}
JS White Space
White space does not mean anything to JavaScript, making it optional. White space does make the code easier to spot though. The following two code examples are both identical and are both completely valid. Which one would you rather use?
let count = 0; while(count<
8){
await BrainPad.System.Println(count
); count =
count + 1;}
var count = 0;
while(count<8){
BrainPad.System.Println(count)
count = count + 1;
}
Code Blocks
Python relies on spaces to know when blocks start and end. C# and JS are the opposite and completely ignore white space. It relies on semicolons and curly brackets to know where code starts and ends and where blocks start and end.
Python
This is a line of code
block
This is a line in a block
C#
This is a line of code;
block{
This is a line in a block;
}
JS
This is a line of code;
block {
This is a line in a block;
}
Loops
So far, all of our programs have executed a small program and then terminated. However, most programs will continue to run, handling specific tasks repeatedly. This happens in a loop that executes a specific block over and over.
We will use a while loop that will run the code inside its block as long as a condition is true, “while” a condition is true.
In this example, the while loop will run as long as the variable count is less than 8. Inside our block, we increment the count by 1 in every loop. We do so by taking the count value, adding 1 to it, and then assigning the result back into count. So, if the count was 3 before that line, we add one to it making it 4 and then assign it back to the same variable itself.
Python
count = 0
while count<8:
BrainPad.System.Println(count)
count = count + 1
C#
var count = 0;
while (count<8){
BrainPad.System.Println(count);
count = count + 1;
}
JS
let count = 0;
while(count<8){
await BrainPad.System.Println(count)
count = count + 1;
}
More on loops in other lessons.
Operators
Operators are used for assigning values, like when we use the = symbol, and for evaluating/comparing two values, like when we use the > symbol. There are other operators to help us mathematically, like the + symbol, and others to help with logic checks.
More on Operators in other lessons.
Flow Control
The while loop used earlier automatically checked for a condition and kept looping as long as that condition was true, which was the count was less than 8 (count<8). But what if we want to check for something without using a while loop? The answer is exactly what you may have guessed, an if statement! You simply have a block, like a loop, but in this case, the block will run only once if a condition is true.
This code will print the count if the count does not equal 4. In most programming languages, “not” is the! symbol. And then != means “does not equal”.
Python
if count != 4:
BrainPad.System.Println(count)
C#
if (count != 4){
BrainPad.System.Println(count);
}
JS
if (count != 4){
await BrainPad.System.Println(count);
}
Let’s now combine the earlier while loop with the if statement, say we want to print numbers 0 to 7, but this time we want to skip 4! Meaning, we will Print() the number if the count does not equal 4.
Python
count = 0
while count<8:
if count != 4:
BrainPad.System.Println(count)
count = count + 1
C#
var count = 0;
while (count<8){
if (count != 4){
BrainPad.System.Println(count);
}
count = count + 1;
}
JS
let count = 0;
while (count<8){
if (count != 4){
await BrainPad.System.Println(count);
}
count = count + 1;
}
Something new happened in the code. We have a nested block. This is where a block goes inside a block. Note how the if statement is inside the while loop. This is called nesting. It is important to note how Python requires 2 indentations for the code inside the if statement.
The results? All numbers show except for 4.
More on flow control in other lessons.
Functions
Functions are tasks a computer has learned to execute. When someone asks you to sleep, you won’t just lie down in place and fall asleep. The “sleep” function (task) will internally handle many tasks, like going to the bedroom, turning lights off, lying in bed, shutting eyes…etc. However, instead of commanding all the above separately, you simply combine it all into “sleep”.
You can create your functions that handle multiple tasks, and you can also use some of the built-in functions that we have pre-created for you. Our favorite is BrainPad.System.PrintLn(). By the way, we will always add () to indicate a function.
Python
BrainPad.System.Println("Hello World!")
C#
BrainPad.System.Println("Hello World!");
JS
await BrainPad.System.Println("Hello World!");
Go ahead and try this code. You can change it to print your name, and even add multiple lines.
Python
BrainPad.System.Println("Hello, my name is:")
BrainPad.System.Println("BrainPad")
C#
BrainPad.System.Println("Hello, my name is:");
BrainPad.System.Println("BrainPad");
JS
await BrainPad.System.Println("Hello, my name is:");
await BrainPad.System.Println("BrainPad");
BrainPad.System.PrintLn() can also print numbers or the results of a mathematical calculation. The first line will print 55 and the second will print 10.
Python
BrainPad.System.Println(55)
BrainPad.System.Println(5+5)
C#
BrainPad.System.Println(55);
BrainPad.System.Println(5+5);
JS
await BrainPad.System.Println(55);
await BrainPad.System.Println(5+5);
What do you think this code will produce?
Python
BrainPad.System.Println("55")
BrainPad.System.Println("5"+"5")
C#
BrainPad.System.Println("55");
BrainPad.System.Println("5"+"5");
JS
await BrainPad.System.Println("55");
await BrainPad.System.Println("5"+"5");
C#, Python, and JS are object-oriented languages. This has a great advantage as functions live inside an object. That function defines how a task is handled for that object. In our examples, we have an object named BrainPad, yet it has another object in it called System. The object System handles the “system” function for the BrainPad to provide several functions. Two of these functions are Print() and PrintLn().
The IntelliSense feature in Visual Studio gives you a list of available options as soon as a period is entered.
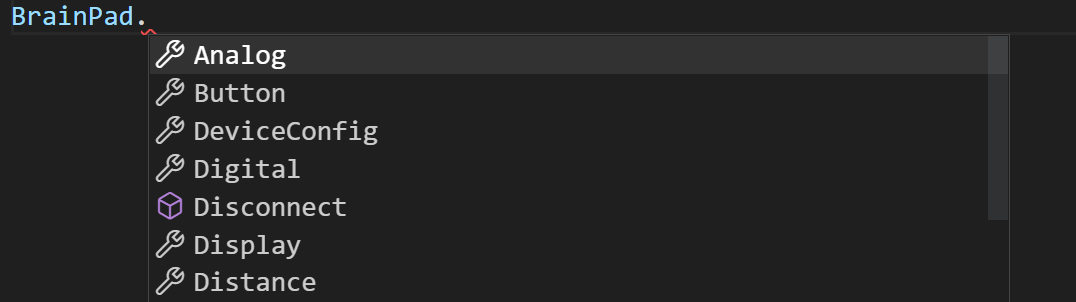
Same for the system:
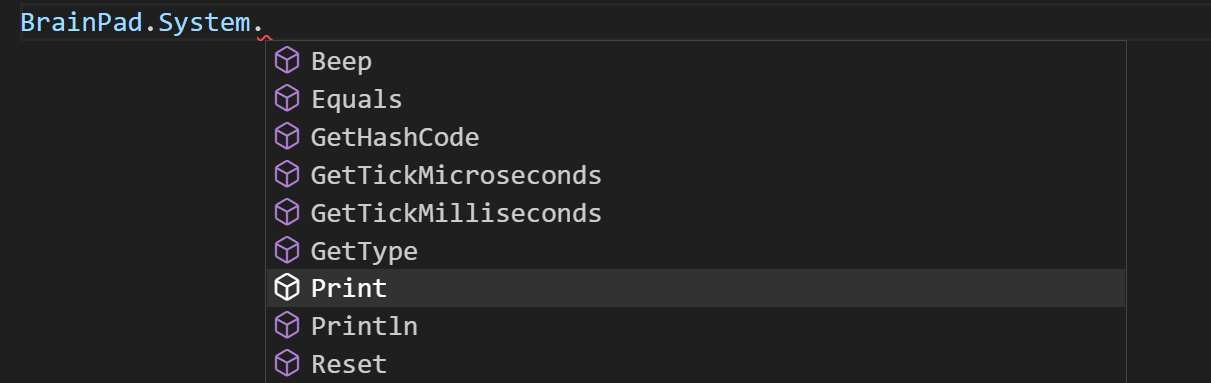
More on functions/methods in other lessons.
BrainStorm
Computers are not as smart as we may assume. They run instructions step-by-step. But if so, how does a computer play music while surfing the web and checking emails? They do so by running billions of instructions per second. It feels like things are happening simultaneously, but it’s just a very fast simple machine.
How do computers compare to our brains? Do you think we process one instruction at a time? How about feelings? Computers always have an answer, but how come we are sometimes unsure? How do we make decisions that we feel confident about, but then we regret those decisions later? Would a computer regret a decision? What is “regret” to a computer if all it knows is a yes or no?