BrainBot Flashy
The BrainBot is equipped with two headlights and two taillights. The headlights work in tandem together and can’t be controlled individually. The taillights, however, work independently.
Prerequisite
This lesson assumes you’ve already assembled your BrainBot, followed the BrainBot Intro and know what NuGets, Using or Import statements we need, and you’ve made your BrainBot Dance.
Headlight()
To use the headlights we use the Headlight() function, which takes one argument. What color we want to use?
Headlight(color)
First let’s select the color using one of the eight built-in colors: White, Yellow, Cyan, Green, Magenta, Red, Blue, or Black. We can pass these colors directly inside the Headlight() function argument. Black turns off the headlights.
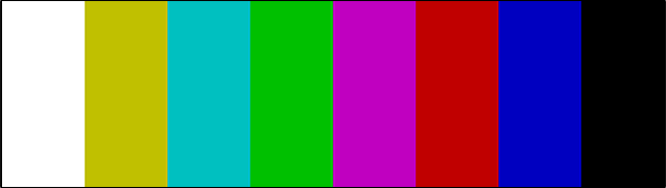
Let’s turn the headlights red.
Headlight(Red);
Headlight(Red)
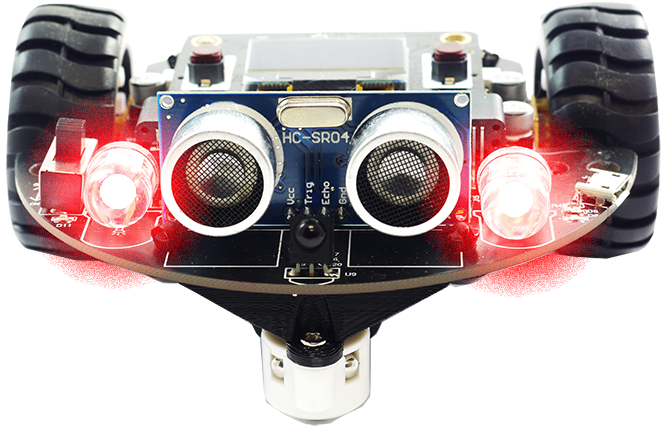
We can use a loop to make the headlights flash between red & blue.
while(true){
Headlight(Red);
Wait(0.5);
Headlight(Blue);
Wait(0.5);
}
while True:
Headlight(Red)
Wait(0.5)
Headlight(Blue)
Wait(0.5)
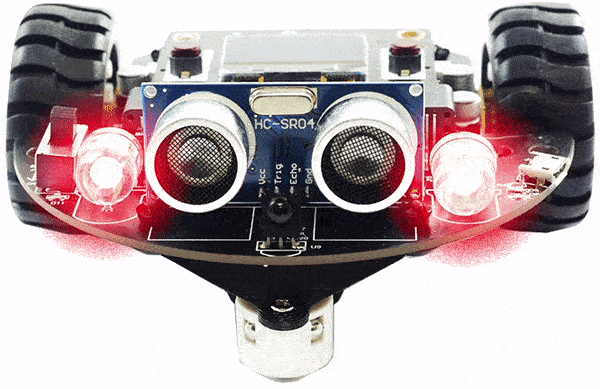
Custom Colors
The headlight uses a combination of 3 LEDs to create any color we would like. We just need to find the HEX value of the color we’d like to use. If we search the Internet for ‘color picker‘, we’ll find a tool that gives us the HEX value we need. We just need add 0x in place of the #. This is how code knows we’re using a HEX value. We can learn more about HEX values and Color in the Drawing lessons under Color().
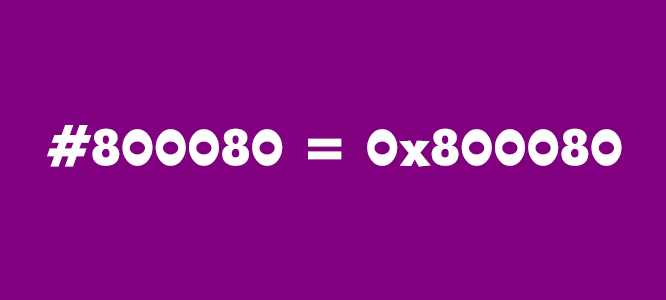
Let’s make the headlights purple using the HEX value.
Headlight(0x800080);
Headlight(0x800080)
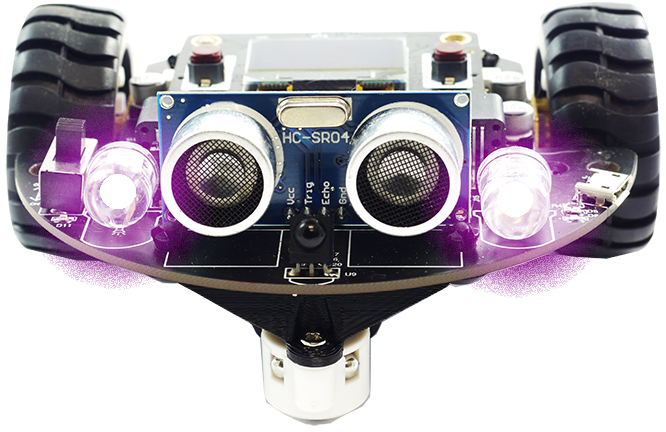
Taillights()
The BrainBot has two independent taillights. We can control them individually within one single Taillight() function. The Taillight() function takes two arguments; the right and left color we’d like to use. Just like with Headlight(), we can use built-in colors or the HEX value.
Taillight(color,color)
Let’s Flash the taillights like we did the headlights inside a loop.
while(true) {
Taillight(Blue,Red);
Wait(0.5);
Taillight(Red,Blue);
Wait(0.5);
}
while True:
Taillight(Blue,Red)
Wait(0.5)
Taillight(Red, Blue)
Wait(0.5)
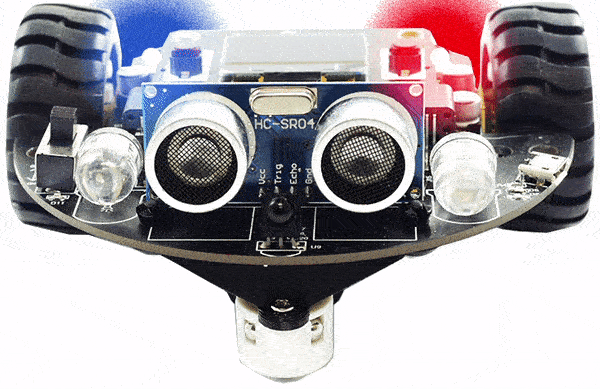
What’s Next?
Let’s move on to BrainBot Collision to help our robot avoid hitting everything in it’s path.
BrainStorm
Why are colors represented in HEX? Since every color is a single byte, the range for each color is 0 to 255. If you didn’t know, a byte is 8 bit and 2 to the power of 8 is 255. What happens when we use a number higher than 255? And how do we show the value? 255255255? All this is representation to us humans. The computer internally is all binary (zeros and ones). A HEX number is easier for us to see and manage. A number of 5577DD is HEX is easy to see that it is 3 bytes (each byte is always 2 digits) and in the case of RGB color, we can easily see that green is 77 in HEX.