Analog Watch
How do smart watches work? In this lesson you will learn how to build an analog-ish clock. This can run on BrainPad Pulse alone, but the results will be much cooler when combined with BrainPower.
Prerequisite
You must have basic understanding of coding and have completed the Drawing lesson.
The Face
The watch face is what you see behind the hands. We will build a simple face that consists of the numbers 1 to 12. We want those to be arranged in a circle. We also want the circle (oval) to have different width and height, to accommodate a non-square screens. We’ll use Sin() and Cos() to calculate/draw circles.
LcdClear(0)
For f=1 to 12
a = (f/6*3.14)-(3.14/2)
x = (64+38*Cos(a))
y = (28+28*Sin(a))
LcdPixel(1, x, y)
Next
LcdShow()
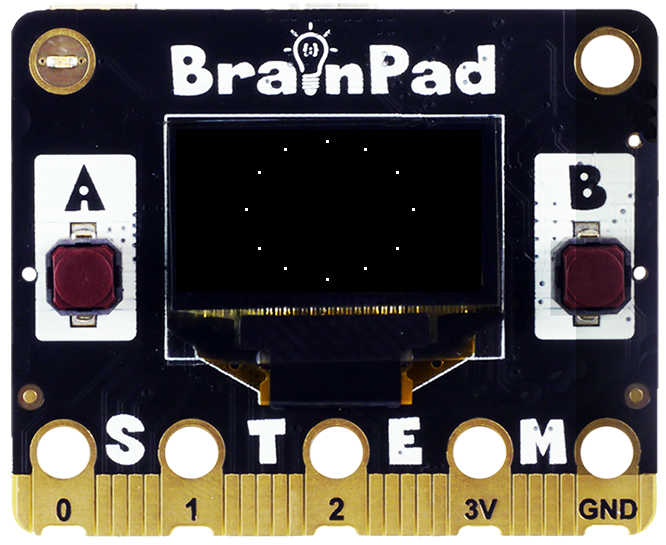
Face Redo
And we finally have 12 points surrounding the “face”. Let’s take this a bit further and put numbers instead of points. We can do this by changing our LcdPixel() function to LcdText() and use the ‘n’ variable in our For-Loop as the number. Use the Str(n) function to convert the variable to a string to display it.
LcdClear(0)
For f=1 to 12
a = (f/6*3.14)-(3.14/2)
x = (64+38*Cos(a))
y = (28+28*Sin(a))
LcdText(Str(f), 1, x, y)
Next
LcdShow()
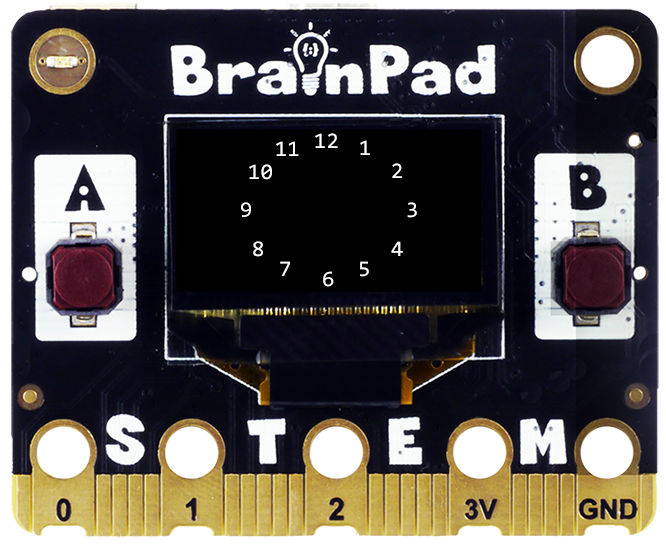
The Hands
The hand is simply a LcdLine() that starts at the middle of the watch and points to a location around the face. The hour hand uses 1 to 12 numbers, but the minute and second hands are from 1 to 60. We’ll create a subroutine for each hand on the clock.
#Draw Hour Hand
@Hour
u = 12 / 2
a = (h / u * 3.14) - (3.14/2)
e = 64 + 20 * Cos(a)
f = 32 + 10 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
#Draw Minute Hand
@Min
u = 60 / 2
a = (m / u * 3.14) - (3.14/2)
e = 64 + 28 * Cos(a)
f = 32 + 18 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
#Draw Second Hand
@Sec
u = 60 / 2
a = (s / u * 3.14) - (3.14/2)
e = 64 + 30 * Cos(a)
f = 32 + 20 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
Before we can run the code we need to create the variables to hold our hours, minutes, and seconds. Let’s say the current time is 3:25 and 40 seconds.
h = 3 #Hours
m = 25 #Minutes
s = 0 #Seconds
LcdClear(0)
Hour()
Min()
Sec()
Face()
LcdShow()
#Draw Watch Face
@Face
For f=1 to 12
a = (f/6*3.14)-(3.14/2)
x = (64+38*Cos(a))
y = (28+28*Sin(a))
LcdText(Str(f), 1, x, y)
Next
Return
#Draw Hour Hand
@Hour
u = 12 / 2
a = (h / u * 3.14) - (3.14/2)
e = 64 + 20 * Cos(a)
f = 32 + 10 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
#Draw Minute Hand
@Min
u = 60 / 2
a = (m / u * 3.14) - (3.14/2)
e = 64 + 28 * Cos(a)
f = 32 + 18 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
#Draw Second Hand
@Sec
u = 60 / 2
a = (s / u * 3.14) - (3.14/2)
e = 64 + 30 * Cos(a)
f = 32 + 20 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
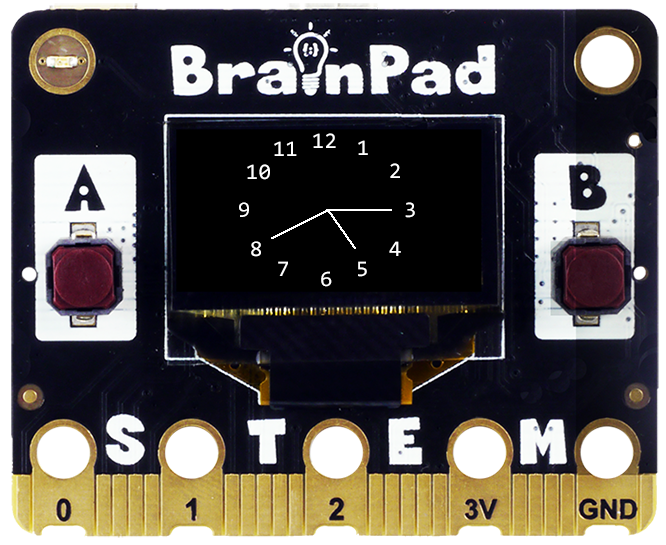
DUE does not have a time service. We will instead create a time counter. We now have generic code that can run on both.
We may have to adjust our Wait() function argument to keep more accurate time since we’re telling it to Wait(1000) milliseconds or 1 second and our code actually takes time to run.
h = 3 #Hours
m = 25 #Minutes
s = 0 #Seconds
@Loop
LcdClear(0)
Face()
Hour()
Min()
Sec()
s = s + 1
if s >= 60:
s = 0
m = m + 1
End
if m >=60:
m = 0
h = h + 1
End
if h > 12:
h = 1
End
Wait(1000)
LcdShow()
Goto Loop
#Draw Watch Face
@Face
For f=1 to 12
a = (f/6*3.14)-(3.14/2)
x = (64+38*Cos(a))
y = (28+28*Sin(a))
LcdText(Str(f), 1, x, y)
Next
Return
#Draw Hour Hand
@Hour
u = 12 / 2
a = (h / u * 3.14) - (3.14/2)
e = 64 + 20 * Cos(a)
f = 32 + 10 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
#Draw Minute Hand
@Min
u = 60 / 2
a = (m / u * 3.14) - (3.14/2)
e = 64 + 28 * Cos(a)
f = 32 + 18 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
#Draw Second Hand
@Sec
u = 60 / 2
a = (s / u * 3.14) - (3.14/2)
e = 64 + 30 * Cos(a)
f = 32 + 20 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
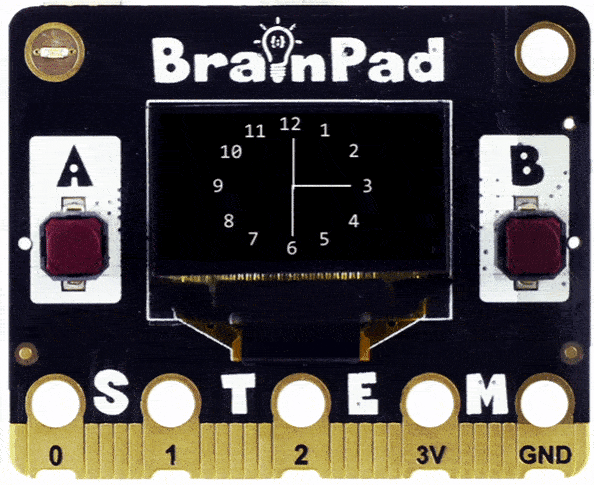
What’s Next?
Let’s use the buttons to set the hours and minutes on our new watch. This should be an easy addition given our current level of programming knowledge. First enable the A & B buttons.
BtnEnable('a', 1)
BtnEnable('b', 1)
Finally add if statements in side our @Loop to check each button and then increment the hours +1 when the A button is pressed, and increment the minutes + 1 when the B button is pressed.
h = 3 #Hours
m = 25 #Minutes
s = 0 #Seconds
BtnEnable('a', 1)
BtnEnable('b', 1)
@Loop
a=BtnDown('a')
b=BtnDown('b')
if a=1
m=m+1
End
if b=1
h=h+1
End
LcdClear(0)
Face()
Hour()
Min()
Sec()
s = s + 1
if s >= 60:
s = 0
m = m + 1
End
if m >=60:
m = 0
h = h + 1
End
if h > 12:
h = 1
End
Wait(1000)
LcdShow()
Goto Loop
#Draw Watch Face
@Face
For f=1 to 12
a = (f/6*3.14)-(3.14/2)
x = (64+38*Cos(a))
y = (28+28*Sin(a))
LcdText(Str(f), 1, x, y)
Next
Return
#Draw Hour Hand
@Hour
u = 12 / 2
a = (h / u * 3.14) - (3.14/2)
e = 64 + 20 * Cos(a)
f = 32 + 10 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
#Draw Minute Hand
@Min
u = 60 / 2
a = (m / u * 3.14) - (3.14/2)
e = 64 + 28 * Cos(a)
f = 32 + 18 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
#Draw Second Hand
@Sec
u = 60 / 2
a = (s / u * 3.14) - (3.14/2)
e = 64 + 30 * Cos(a)
f = 32 + 20 * Sin(a)
LcdLine(1,64, 32, e, f)
Return
BrainStorm
How does a computer keep track of time when it is off? It actually does not! Once power is down, everything is reset internally. However, there is a small circuit inside caller RTC (Real Time Clock) and this circuit is extremely low power and it has its own tiny battery. When the computer is off, the RTC will continue to run and continue to keep track of time. The moment the computer is powered up, it will read the current time from the RTC.