This is a mid to advanced level game that showcases the use of DUE to build Brix game!
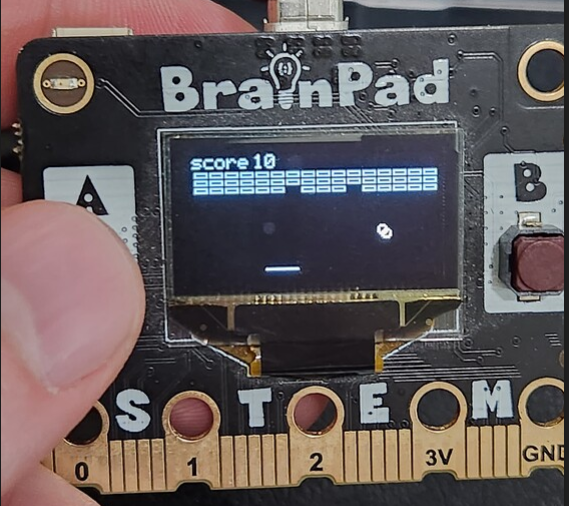
Prerequisite
You must have completed the Gaming Intro lesson.
Game Objective
The player will need to destroy all blocks.
Array’s Usefulness
DUE Script keeps things simple when it comes to variables. Instead of letting the user define variables and types, it simply ships with 26 variables! This can get a bit messy when writing larger programs. An alterative is to use arrays found in DUE Script. For example, we can use array b[ ] to hold the ball information. We could say b[0] is x, b[1] is y, b[2] is deltaX…etc. Moving the ball will look something like this.
b[0]=b[0]+b[2]
b[1]=b[1]+b[3]
Start vs Loop
It is a good practice in any program to have an initialization code and a main loop. This is especially useful when coding devices. A device’s program never ends. We will always have a loop that runs endlessly. Before entering the main loop, it is a good practice to have a start() method/subroutine that “Gets things ready”. It can initialize variable and get some hardware ready. For example, some connected sensors need to be configured before they are used. The initialization is done in start() and then then repeating code uses in the @Loop.
start()
@Loop
## add repeating code here
Goto Loop
@start
# Get things ready!
Return
Code can be broken further as needed. For example, a game can process collisions in a separate function and then does all drawings in a function.
Code Listing
start()
@loop
# Move ball
b[0]=b[0]+b[2]
b[1]=b[1]+b[3]
# Move paddle
if x>0 && dread('a',1)=0:x=x-2.3:end
if x<112 && dread('b',1)=0:x=x+2.3:end
# Check missed paddle
if b[1]>60:reset():l=l-1:end
# Check paddle collision
if b[1]>=60-r
if b[0]+r>=x && (b[0]-r)<=x+16
b[1]=60-r
b[2]=((b[0]-(x+8))/8)*2
b[3]=-b[3]
Beep(200,10,100)
end
end
# Check boundary collision
if b[0]<=r || b[0]>=127-r:b[2]=-b[2]:end
if b[1]<=0:b[3]=-b[3]:end
# Check brick collision
if b[1]<=22 && b[1]>=10
u = b[0]
v = b[1]-10
i = trunc(u/8) + trunc(v/3)*16
if i>=0 && i<48
if z[i]
b[3]=-b[3]
z[i]=0
Beep(400,10,100)
c=c-1
s=s+5
end
end
end
# Update screen
lcdclear(0)
u=0:v=0
for i in range(0,48)
u=(i%16)*8
v=10+trunc(i/16)*4
if z[i]
lcdrect(1,u+1,v-1,7,3)
end
next
if l = 3
lcdfill(1,115,2,3,3)
lcdfill(1,120,2,3,3)
lcdfill(1,125,2,3,3)
end
if l = 2
lcdfill(1,120,2,3,3)
lcdfill(1,125,2,3,3)
end
if l = 1
lcdfill(1,125,2,3,3)
end
if l = 0
over()
end
lcdline(1,x,60,x+16,60)
lcdcircle(1, b[0], b[1], r)
lcdtext("score",1,0,0)
lcdtext(str(s),1,32,0)
lcdshow()
if c=0
# Restart if we got all the bricks
start()
reset()
end
goto loop
@reset
# Reset ball
b[0]=64-r
b[1]=28
if b[0]>x
b[2]=-1
else
b[2]=1
end
lcdtext("Ready",1,52,30)
lcdshow();
wait(1000)
return
@start
c=48 # Brick count
dim z[c] # Bricks
dim b[4] # Ball x,y,dx,dy
r=2 # ball radius
d=r*2 # ball diameter
x=64-8 # Paddle X
w=16 # Paddle width
l=3 # Start with 3 lives
# Setup the bricks
for i in range(0,48):z[i]=1:next
# Setup ball
b[0]=64-r
b[1]=20
b[2]=1
b[3]=2
return
@over
lcdclear(0)
lcdtexts("Game Over", 1, 10, 10, 2, 2)
lcdtexts(Fmt("Score ",s), 1, 15, 30, 2, 2)
lcdshow()
wait(2000)
lcdclear(0)
start()
reset()
return
What is Next?
Modify the code to change how the game works, or completely make a new game.
BrainStorm
In the early computer days, things were very simple and resources were severely limited. Game creators had to be very creative to make exciting games with limited resources. Can you think of examples that you are familiar with? Tetris? Packman? Mario?