Math Art
This lesson will create an AI artist, and show how fun math can be, by creating creative circle (trig) artist! Do not worry, you can use this lesson even if you do not understand trigonometry.
Prerequisite
You must have basic understanding of coding and have completed the Drawing lesson.
A Circle
There are many ways to draw circles very effectively, but this lesson will rely on the basics and use Sin and Cos. All you need to know is that these return a point around a circle. You give them an angle around a circle and they return a point. They assume the circle has size (diameter) of 1, but we can multiply the results with the desired diameter.
Sin() and Cos() take angles in radians. We will convert to radians and place value in r variable.
A circle is 360 degrees and so our loop will go from 0 to 360 and draw the points.
LcdClear(0)
For a = 0 to 360
r = (3.14 / 180) * a
x = Cos(r) * 30
y = Sin(r) * 30
LcdPixel(1,x,y)
Next
LcdShow()
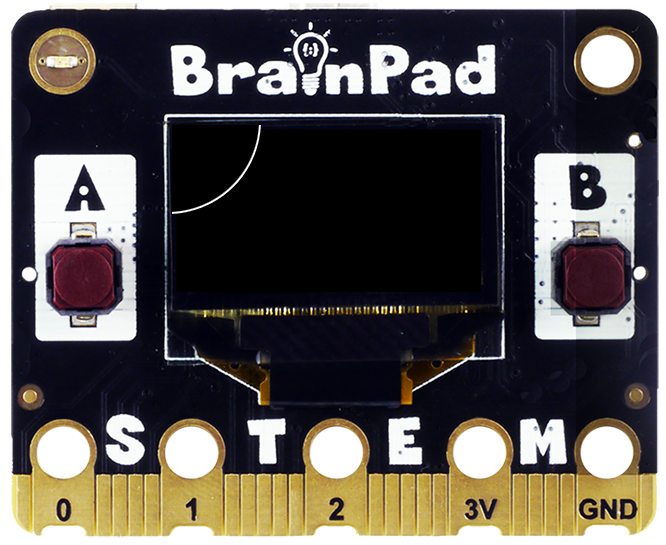
Oh look, we have a circle… sort of! The circle is positioned at 0,0 so we are only seeing a quarter of it. We can shift the circle to the middle of the screen.
LcdClear(0)
For a = 0 to 360
r = (3.14 / 180) * a
x = Cos(r) * 30
y = Sin(r) * 30
LcdPixel(1,64+x,32+y)
Next
LcdShow()
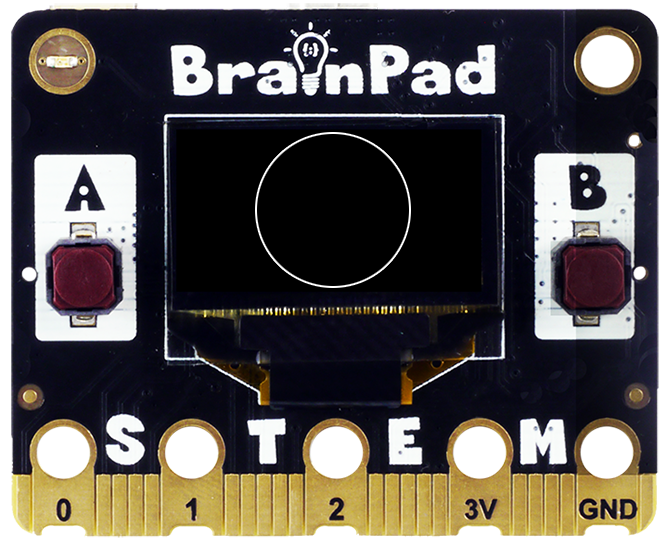
If you want to see each individual pixel being draw, move the LcdShow() inside the loop. This will run slower as the system needs to update the screen for every pixel. In fact, lets do that going forward. You can always move LcdShow() to the end to speed up the drawing.
LcdClear(0)
For a = 0 to 360
r = (3.14 / 180) * a
x = Cos(r) * 30
y = Sin(r) * 30
LcdPixel(1,64+x,32+y)
LcdShow()
Next
Trig Race
What happens if Sin() is going twice is fast as Cos()? We do this by multiplying the angle by 2. This is making one angle go twice as fast as the other.
LcdClear(0)
For a = 0 to 360
r = (3.14 / 180) * a
x = Cos(r) * 30
y = Sin(r*2) * 30
LcdPixel(1,64+x,32+y)
LcdShow()
Next
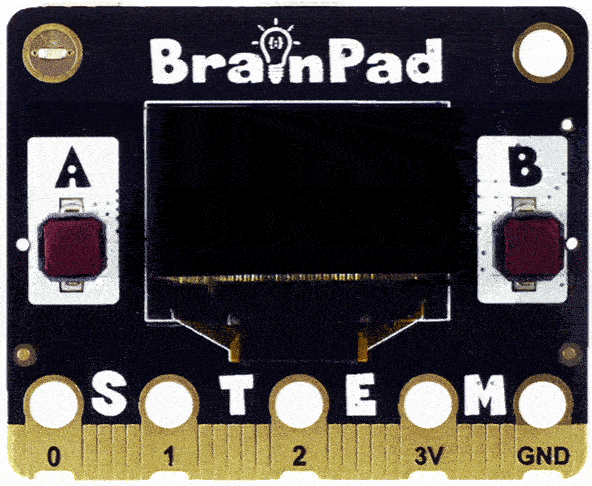
Do you see how the circle got folded in and now we have an infinity symbol. Change the code to 3 times faster. Do you see a three curves?
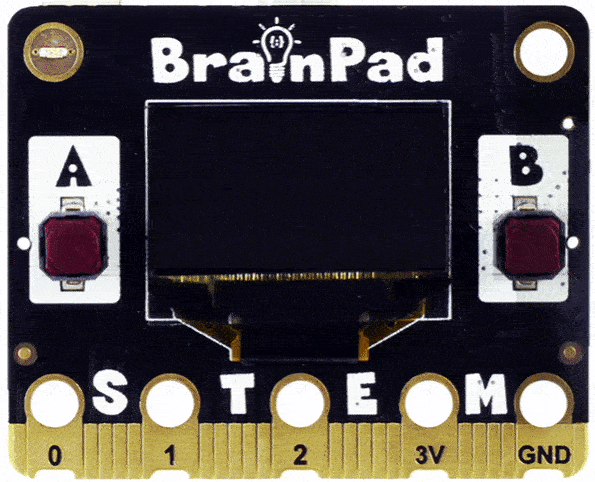
Can you guess what 4 or 5 would look like?
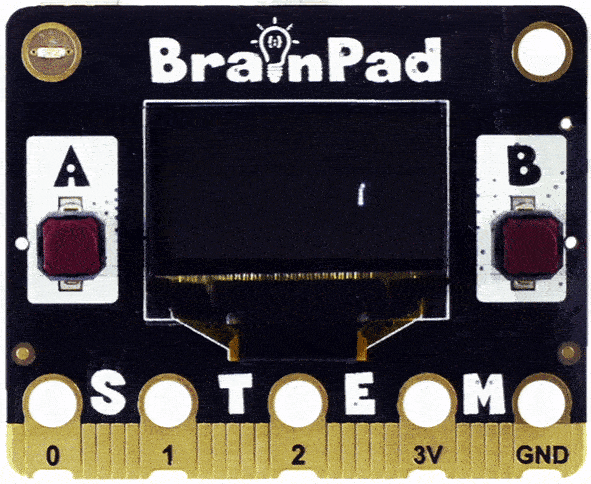
You may have guessed right, but what if you use 2.2?
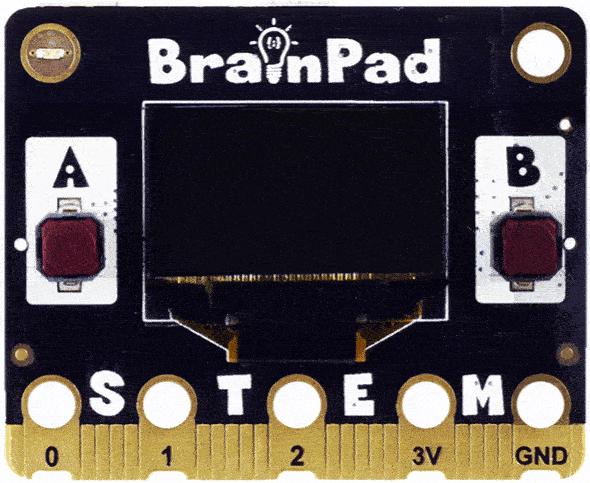
Looks like we are onto something, but the drawing did not complete. This is because the 2.0 will complete, but then the fraction 0.2 will not get a whole round. For 0.2 to become 1 (full round) you need to repeat our loop 5 times.
LcdClear(0)
For a = 0 to 360*5
r = (3.14 / 180) * a
x = Cos(r) * 30
y = Sin(r*2.2) * 30
LcdPixel(1,64+x,32+y)
LcdShow()
Next
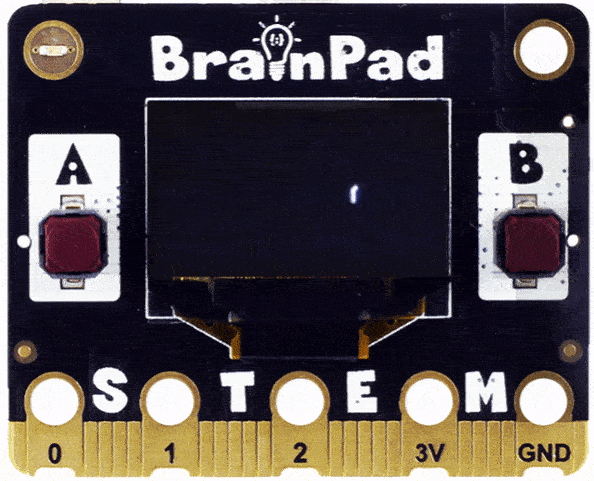
Circle of Circles
A circle can become all kinds of interesting shapes on its own, but what if we made a circle spiral along the circumference of another circle? The spiraling circle should go faster then the other circle it is going around. If not faster, then we will just have a larger circle. We will try 2 times faster and see what the results may look like.
LcdClear(0)
For a = 0 to 360
r = (3.14 / 180) * a
X = Cos(r) * 20
Y = Sin(r) * 20
c = Cos(r*2) * 10
d = Sin(r*2) * 10
LcdPixel(1, 64 + X + c, 32 + Y + d)
LcdShow()
Next
Is that a badly shaped heart? Change the multiplier of the spiral to 3, 4, 5… etc. Can you visualize what is happening?
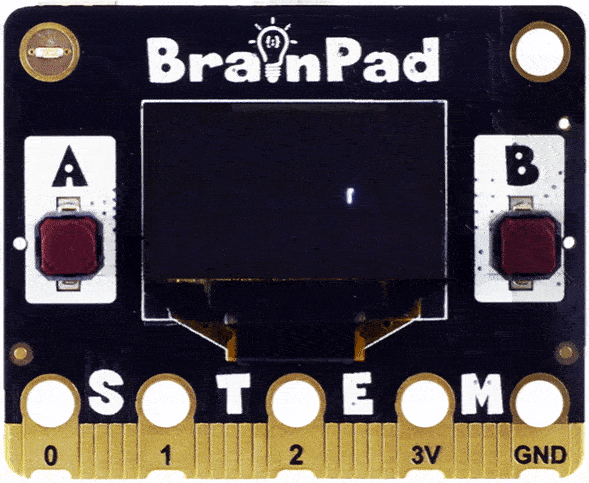
You can see what the circle is doing, but here is something impossible to visualize! What if we did 6 multiplier for c but then used 5 for d?
LcdClear(0)
For a = 0 to 360
r = (3.14 / 180) * a
X = Cos(r) * 20
Y = Sin(r) * 20
c = Cos(r*6) * 10
d = Sin(r*5) * 10
LcdPixel(1, 64 + X + c, 32 + Y + d)
LcdShow()
Next
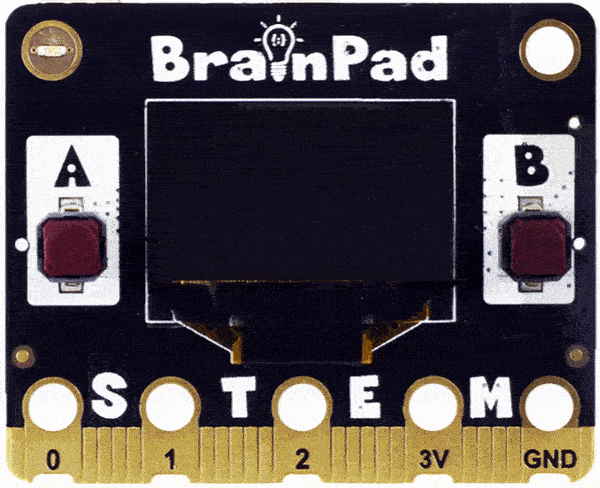
We bet you have never seen math this cool before! Ok, let’s do it the other way around. Keep the same multiplier for the spiral and change it for the circle being surrounded.
LcdClear(0)
For a = 0 to 360
r = (3.14 / 180) * a
X = Cos(r) * 20
Y = Sin(r*2) * 20
c = Cos(r*8) * 10
d = Sin(r*8) * 10
LcdPixel(1, 64 + X + c, 32 + Y + d)
LcdShow()
Next
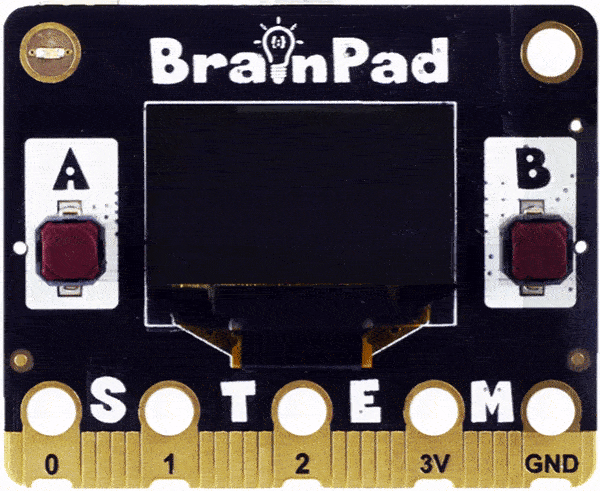
Continuous Art
We will build an artist that just generates trig math art forever using random numbers.
@loop
LcdClear(0)
v = Rnd(15)
w = Rnd(15)
LcdText(Fmt("(", v, ", ", w, ")"),1,0*8,0)
For a = 0 to 360
r = (3.14 / 180) * a
X = Cos(r) * 20
Y = Sin(r) * 20
c = Cos(r* v) * 10
d = Sin(r* w) * 10
LcdPixel(1, 64 + X + c, 32 + Y + d)
Next
LcdShow()
Goto loop
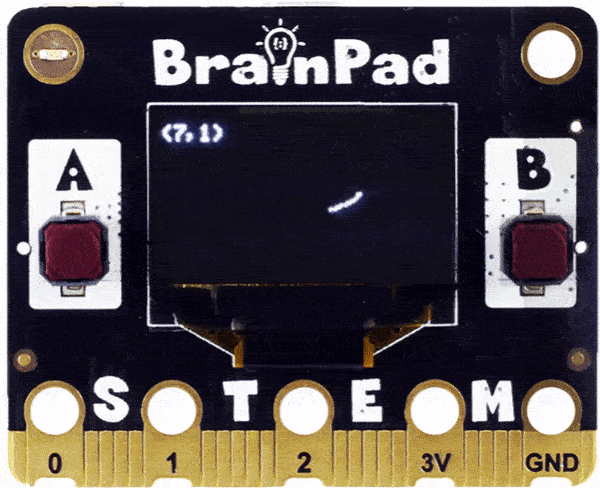
What’s Next?
Are you ready for some game making? Or control robots? See al of the DUE Script lessons.
BrainStorm
Who knew math can be so creative on its own?! Some of these drawing start as complete random but complete beautifully.