Variables
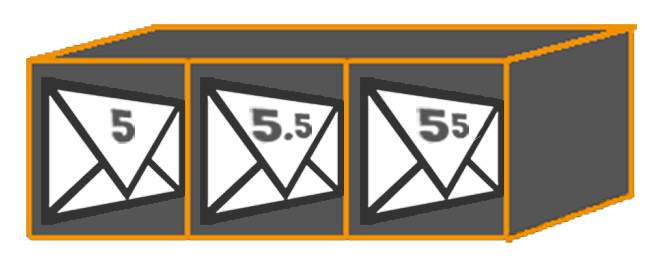
Prerequisite
This page assumes you have completed the DUE Script Intro lesson.
Intro
A variable is like a mailbox for computers. It is used to hold a value. In DUE Script, there are 26 predefined variables represented by the alphabet letters A to Z. A user can’t create variables with other names. In this example, we assign x with value 5.
x = 5
You can use comments to remind yourself of what the variable is used for since variables names can’t be made and all variables are global, meaning they can be accessed from anywhere in the code. We usually use letters that can relate to the use. For example, if using a variable to hold “area” we use the “a” variable. DUE Script does not care but this makes it easier for us to remember when you programs start gowning.
a = 5 # we use a to hold area
Print(a)
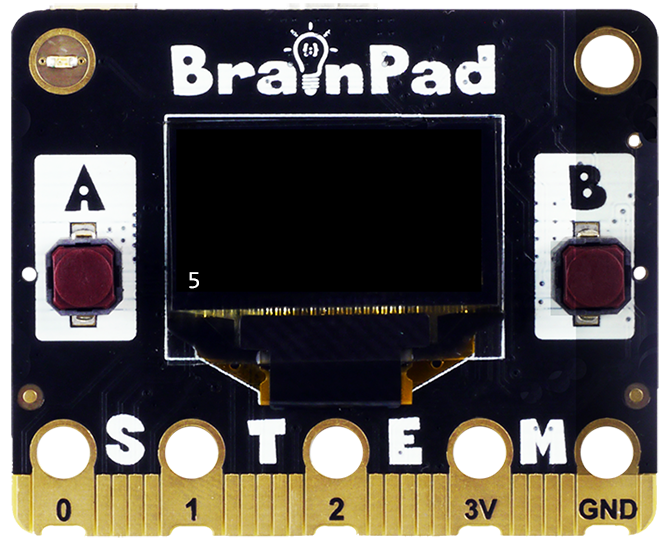
Variable Types
Variables in DUE Script are type-less. The 26 predefined variables are all numerical and can hold fractional values. Strings can’t be stored in variables.
Arrays
Arrays are a way to hold a list of variables. Similar to variables, 26 arrays are predefined with the alphabet letters. DUE Script can distinguish between variables and array when it sees the use of square brackets [ ].
Moreover, the size of the array is dynamic. The Dim command is used to allocate the needed memory and set the size of a specific array.
Dim a[5] # We need a to be able to hold 5 values
We can access and assign variables to specific spots in an array. We do this by including a number in brackets after the name, as shown below. This is called indexing. It is very important to note that indexes start at 0. An array of size 10 starts at index 0 and ends at index 9.
a[2] = 6 # set the third element in the array to 6
Arrays values can be assigned in bulk.
# Dim and assign
Dim a[5] = [8, 8, 0, 0, 0, 1, 1, 0]
# Dim and assign later
Dim b[3]
b=[1,22,333]
Initialization values can me on multi line but there is one simple restriction, where every line must end of comma.
Dim a[2+(8*8)] = [8,8,
0, 0, 0, 1, 1, 0, 0, 0,
0, 0, 1, 1, 1, 1, 0, 0,
0, 1, 1, 1, 1, 1, 1, 0,
1, 1, 0, 1, 1, 0, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1,
0, 0, 1, 0, 0, 1, 0, 0,
0, 1, 0, 1, 1, 0, 1, 0,
1, 0, 1, 0, 0, 1, 0, 1]
LcdClear(0)
LcdImgS(a,60,30,3,3,0)
LcdShow()
Hex and Binary
You may find places where using a hexadecimal (hex) or binary numbers are useful. Hex numbers are proceeded by 0x and binary numbers are proceeded by 0b. Can you guess what will the output be from these three lines?
Print(16)
Print(0x10)
Print(0b1000)
What’s Next?
Learn about Loops and how they are used to repeat tasks.
BrainStorm
We know that anything between double-quotes is a string. With that in mind, what is the difference between 53 and “53”? Can you guess what this code will print? Try it! While we may see them as the same thing, computers are not that smart! 53 is a value that can be stored in a variable. “53” is a string, which is an array! “53” is an array of size 2, first index contain the ASCII value of the letter 5 and the second index is holding the ASCII value of the letter 3. Computers see “AB” or “53” as test, no numerical values here.
There are places later where you will use ASCII values and to do so, you will use a single quote. ‘A’ is the ASCII value of A. Since DUE Script is not case sensitive, it will see everything as lower case. Meaning, ‘A’ and ‘a’ are both the same and both return the value 97. Try print(‘A’) and search the web for ASCII table.