BrainSense advanced Dashboard
In this lesson, we aim to advance the representation and visualization of our sensor data through the colorful graphic BrainPad Rave LCD screen.
Prerequisite
It’s essential to learn the programming of all sensors and modules in the BrainSense kit, outlined comprehensively in the BrainSense modules. Alternatively, you can refer to the BrainSense Demo for a complete walkthrough.
Drawing Dynamic Bar Chart
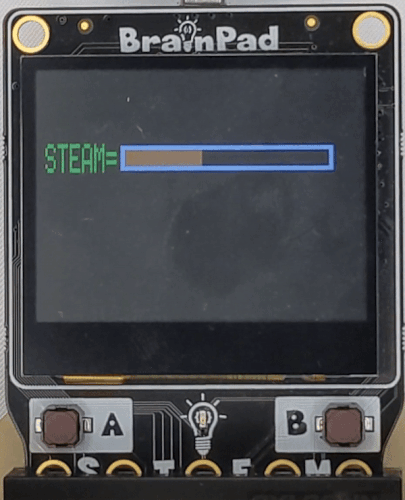
The bar design should comprise three rectangles: the first, a constant and solid representation outlining the overall shape of the bar; the second, representing the background of the bar when it’s empty; and the third, situated within the boundaries of the first and positioned above the second, dynamically adjusts in width based on sensor values. With values ranging from 0 to 100, the width of the third rectangle precisely mirrors the sensor value within this range.
Let’s take the Steam sensor, for instance, and begin by rendering its name as a label with a green color on the BrainPad Rave LCD:
Python
from DUELink.DUELinkController import DUELinkController
available_port = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(available_port)
# Initialize sensor pins
steam_pin = 5
# Function to update LCD display
def rave_lcd(steam_sensor_value):
y=35
x=5
BrainPad.Display.DrawTextScale("STEAM= ",0x00ff00, x, y,1,2)
# Main loop
while True:
BrainPad.Display.Clear(0)
steam_sensor_value = BrainPad.Analog.Read(steam_pin)
rave_lcd(steam_sensor_value)
BrainPad.Display.Show()
C#
using GHIElectronics.DUELink;
namespace BrainSense
{
class Program
{
public static class Globals
{
// Initialize sensor pins
public static int steam_pin = 5;
}
// Main loop
static void Main(string[] args)
{
DUELinkController BrainPad = new DUELinkController(DUELinkController.GetConnectionPort());
while (true)
{
BrainPad.Display.Clear(0);
double steam_sensor_value = BrainPad.Analog.Read(Globals.steam_pin); steam_sensor_value = (double)System.Math.Round(steam_sensor_value, 2);
RaveLCD(steam_sensor_value, BrainPad);
BrainPad.Display.Show();
}
}
// Function to update LCD display - rave_lcd
static void RaveLCD(double steam_sensor_value, DUELinkController BrainPad)
{
int y = 35;
int x = 5;
BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
}
}
}
JS
import { SerialUSB } from './serialusb.js';
import * as due from './duelink.js';
let BrainPad = new due.DUELinkController(new SerialUSB());
await BrainPad.Connect();
// Initialize sensor pins
const steam_pin = 5;
// Function to update LCD display - rave_lcd
async function rave_lcd(steam_sensor_value) {
let y = 35;
let x = 5;
await BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
}
// Main loop
async function mainLoop() {
while (true) {
await BrainPad.Display.Clear(0);
let steam_sensor_value = await BrainPad.Analog.Read(steam_pin);
await rave_lcd(steam_sensor_value);
await BrainPad.Display.Show();
}
}
// Call the main loop function
mainLoop();
Following this, we proceed to draw the two filled rectangles—the white boundaries and the black background—forming the foundational structure of the bar by LcdFill(color, x, y, width, height)
.
Python
from DUELink.DUELinkController import DUELinkController
available_port = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(available_port)
# Initialize sensor pins
steam_pin = 5
# Function to update LCD display
def rave_lcd(steam_sensor_value):
y=35
x=5
BrainPad.Display.DrawTextScale("STEAM= ",0x00ff00, x, y,1,2)
width = 103
height = 12
y = 36
x = 42
BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height)
BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4)
# Main loop
while True:
BrainPad.Display.Clear(0)
steam_sensor_value = BrainPad.Analog.Read(steam_pin)
rave_lcd(steam_sensor_value)
BrainPad.Display.Show()
C#
using GHIElectronics.DUELink;
namespace BrainSense
{
class Program
{
public static class Globals
{
// Initialize sensor pins
public static int steam_pin = 5;
}
// Main loop
static void Main(string[] args)
{
DUELinkController BrainPad = new DUELinkController(DUELinkController.GetConnectionPort());
while (true)
{
BrainPad.Display.Clear(0);
double steam_sensor_value = BrainPad.Analog.Read(Globals.steam_pin); steam_sensor_value = (double)System.Math.Round(steam_sensor_value, 2);
RaveLCD(steam_sensor_value, BrainPad);
BrainPad.Display.Show();
}
}
// Function to update LCD display - rave_lcd
static void RaveLCD(double steam_sensor_value, DUELinkController BrainPad)
{
int y = 35;
int x = 5;
BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
int width = 103;
int height = 12;
y = 36;
x = 42;
// drawing the white rectangles
BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height);
BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4);
}
}
}
JS
import { SerialUSB } from './serialusb.js';
import * as due from './duelink.js';
let BrainPad = new due.DUELinkController(new SerialUSB());
await BrainPad.Connect();
// Initialize sensor pins
const steam_pin = 5;
// Function to update LCD display - rave_lcd
async function rave_lcd(steam_sensor_value) {
let y = 35;
let x = 5;
await BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
let width = 103;
let height = 12;
y = 36;
x = 42;
// Drawing the white rectangle
await BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height);
await BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4);
}
// Main loop
async function mainLoop() {
while (true) {
await BrainPad.Display.Clear(0);
let steam_sensor_value = await BrainPad.Analog.Read(steam_pin);
await rave_lcd(steam_sensor_value);
await BrainPad.Display.Show();
}
}
// Call the main loop function
mainLoop();
Lastly, we draw the third rectangle, representing the sensor value as the width of the rectangle within the range of 0 to 100.
We are positioned above the black rectangle, surrounded by the white boundaries, providing a visual representation of the sensor value on the BrainPad Rave LCD.
Python
from DUELink.DUELinkController import DUELinkController
available_port = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(available_port)
# Initialize sensor pins
steam_pin = 5
# Function to update LCD display
def rave_lcd(steam_sensor_value):
y=35
x=5
BrainPad.Display.DrawTextScale("STEAM= ",0x00ff00, x, y,1,2)
width = 103
height = 12
y = 36
x = 42
# drawing the white rectangles
BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height)
BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4)
x = 44
y = 38
height = 8
# drawing the bars based on sensors values
BrainPad.Display.DrawFillRect(0xf08000, x, y, steam_sensor_value, height)
# Main loop
while True:
BrainPad.Display.Clear(0)
steam_sensor_value = BrainPad.Analog.Read(steam_pin)
rave_lcd(steam_sensor_value)
BrainPad.Display.Show()
C#
using GHIElectronics.DUELink;
namespace BrainSense
{
class Program
{
public static class Globals
{
// Initialize sensor pins
public static int steam_pin = 5;
}
// Main loop
static void Main(string[] args)
{
DUELinkController BrainPad = new DUELinkController(DUELinkController.GetConnectionPort());
while (true)
{
BrainPad.Display.Clear(0);
double steam_sensor_value = BrainPad.Analog.Read(Globals.steam_pin); steam_sensor_value = (double)System.Math.Round(steam_sensor_value, 2);
RaveLCD(steam_sensor_value, BrainPad);
BrainPad.Display.Show();
}
}
// Function to update LCD display - rave_lcd
static void RaveLCD(double steam_sensor_value, DUELinkController BrainPad)
{
int y = 35;
int x = 5;
BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
int width = 103;
int height = 12;
y = 36;
x = 42;
// drawing the white rectangles
BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height);
BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4);
x = 44;
y = 38;
height = 8;
// drawing the bars based on sensors values
BrainPad.Display.DrawFillRect(0xf08000, x, y, (int)steam_sensor_value, height);
}
}
}
JS
import { SerialUSB } from './serialusb.js';
import * as due from './duelink.js';
let BrainPad = new due.DUELinkController(new SerialUSB());
await BrainPad.Connect();
// Initialize sensor pins
const pir_pin = 3;
const flame_pin = 4;
const steam_pin = 5;
const temp_humid_pin = 6;
const photo_pin = 0;
const soil_pin = 1;
// Function to update LCD display - rave_lcd
async function rave_lcd(steam_sensor_value) {
let y = 35;
let x = 5;
await BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
let width = 103;
let height = 12;
y = 36;
x = 42;
// Drawing the white rectangle
await BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height);
await BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4);
x = 44;
y = 38;
height = 8;
// Drawing the bars based on sensor values
await BrainPad.Display.DrawFillRect(0xf08000, x, y, steam_sensor_value, height);
}
// Main loop
async function mainLoop() {
while (true) {
await BrainPad.Display.Clear(0);
let steam_sensor_value = await BrainPad.Analog.Read(steam_pin);
await rave_lcd(steam_sensor_value);
await BrainPad.Display.Show();
}
}
// Call the main loop function
mainLoop();
Finally, let’s extend the design to accommodate three dynamic bars for the Steam, Light, and Soil Moisture sensors. Additionally, we can display the Temperature and Humidity sensor values as numeric data at the bottom of the screen on the BrainPad Rave LCD.
This comprehensive visualization will offer a quick and insightful overview of multiple sensor readings simultaneously.
Python
from DUELink.DUELinkController import DUELinkController
available_port = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(available_port)
# Initialize sensor pins
pir_pin = 3
flame_pin = 4
steam_pin = 5
temp_humid_pin = 6
photo_pin = 0
soil_pin = 1
# Initialize actuator pins
servo_pin = 10
fan_pin = 11
led_pin = 12
horn_pin = 13
# Function to update LCD display
def rave_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value,
humidity_sensor_value):
BrainPad.Display.DrawTextScale("BrainSense", 0xf08000, 20, 10, 2, 2)
y = 35
x = 5
BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2)
BrainPad.Display.DrawTextScale("LIGHT= ", 0x00ff00, x, y + 20, 1, 2)
BrainPad.Display.DrawTextScale("SOIL= ", 0x00ff00, x, y + 40, 1, 2)
BrainPad.Display.DrawText("Celsius= ", 0xf0ff00, x + 40, y + 60)
BrainPad.Display.DrawText(float(temperature_sensor_value), 0xf0ff00, x + 100, y + 60)
BrainPad.Display.DrawText("Humidity= ", 0x00fff0, x + 40, y + 70)
BrainPad.Display.DrawText(float(humidity_sensor_value), 0x00fff0, x + 100, y + 70)
width = 103
height = 12
y = 36
x = 42
# drawing the white rectangles
for n in range(0, 3):
BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height)
BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4)
y = y + 20
x = 44
y = 38
height = 8
# drawing the bars based on sensors values
BrainPad.Display.DrawFillRect(0xf08000, x, y, steam_sensor_value, height)
BrainPad.Display.DrawFillRect(0xf08000, x, y + 20, light_sensor_value, height)
BrainPad.Display.DrawFillRect(0xf08000, x, y + 40, soilMoisture_sensor_value, height)
# Main loop
while True:
BrainPad.Display.Clear(0)
motion_sensor_value = BrainPad.Digital.Read(pir_pin, 1)
steam_sensor_value = BrainPad.Analog.Read(steam_pin)
flame_sensor_value = BrainPad.Digital.Read(flame_pin, 1)
light_sensor_value = BrainPad.Analog.Read(photo_pin)
soilMoisture_sensor_value = BrainPad.Analog.Read(soil_pin)
temperature_sensor_value = BrainPad.Temperature.Read(temp_humid_pin, 11)
humidity_sensor_value = BrainPad.Humidity.Read(temp_humid_pin, 11)
rave_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value,
humidity_sensor_value)
BrainPad.Display.Show()
C#
using GHIElectronics.DUELink;
namespace BrainSense
{
class Program
{
public static class Globals
{
// Initialize sensor pins
public static int pir_pin = 3;
public static int flame_pin = 4;
public static int steam_pin = 5;
public static int temp_humid_pin = 6;
public static int photo_pin = 0;
public static int soil_pin = 1;
// Initialize actuator pins
public static int servo_pin = 10;
public static int fan_pin = 11;
public static int led_pin = 12;
public static int horn_pin = 13;
}
// Main loop
static void Main(string[] args)
{
DUELinkController BrainPad = new DUELinkController(DUELinkController.GetConnectionPort());
while (true)
{
BrainPad.Display.Clear(0);
bool motion_sensor_value = BrainPad.Digital.Read(Globals.pir_pin, 1);
double steam_sensor_value = BrainPad.Analog.Read(Globals.steam_pin); steam_sensor_value = (double)System.Math.Round(steam_sensor_value, 2);
bool flame_sensor_value = BrainPad.Digital.Read(Globals.flame_pin, 1);
double light_sensor_value = BrainPad.Analog.Read(Globals.photo_pin); light_sensor_value = (double)System.Math.Round(light_sensor_value, 2);
double soilMoisture_sensor_value = BrainPad.Analog.Read(Globals.soil_pin); soilMoisture_sensor_value = (double)System.Math.Round(soilMoisture_sensor_value, 2);
double temperature_sensor_value = BrainPad.Temperature.Read(Globals.temp_humid_pin, 11); temperature_sensor_value = (double)System.Math.Round(temperature_sensor_value, 2);
double humidity_sensor_value = BrainPad.Humidity.Read(Globals.temp_humid_pin, 11); humidity_sensor_value = (double)System.Math.Round(humidity_sensor_value, 2);
RaveLCD(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value, BrainPad);
BrainPad.Display.Show();
}
}
// Function to update LCD display - rave_lcd
static void RaveLCD(double steam_sensor_value, double light_sensor_value, double soilMoisture_sensor_value, double temperature_sensor_value, double humidity_sensor_value, DUELinkController BrainPad)
{
BrainPad.Display.DrawTextScale("BrainSense", 0xf08000, 20, 10, 2, 2);
int y = 35;
int x = 5;
BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
BrainPad.Display.DrawTextScale("LIGHT= ", 0x00ff00, x, y + 20, 1, 2);
BrainPad.Display.DrawTextScale("SOIL= ", 0x00ff00, x, y + 40, 1, 2);
BrainPad.Display.DrawText("Celsius= ", 0xf0ff00, x + 40, y + 60);
BrainPad.Display.DrawText(temperature_sensor_value.ToString(), 0xf0ff00, x + 100, y + 60);
BrainPad.Display.DrawText("Humidity= ", 0x00fff0, x + 40, y + 70);
BrainPad.Display.DrawText(humidity_sensor_value.ToString(), 0x00fff0, x + 100, y + 70);
int width = 103;
int height = 12;
y = 36;
x = 42;
// drawing the white rectangles
for (int n = 0; n < 3; n++)
{
BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height);
BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4);
y = y + 20;
}
x = 44;
y = 38;
height = 8;
// drawing the bars based on sensors values
BrainPad.Display.DrawFillRect(0xf08000, x, y, (int)steam_sensor_value, height);
BrainPad.Display.DrawFillRect(0xf08000, x, y + 20, (int)light_sensor_value, height);
BrainPad.Display.DrawFillRect(0xf08000, x, y + 40, (int)soilMoisture_sensor_value, height);
}
}
}
JS
import { SerialUSB } from './serialusb.js';
import * as due from './duelink.js';
let BrainPad = new due.DUELinkController(new SerialUSB());
await BrainPad.Connect();
// Initialize sensor pins
const pir_pin = 3;
const flame_pin = 4;
const steam_pin = 5;
const temp_humid_pin = 6;
const photo_pin = 0;
const soil_pin = 1;
// Function to update LCD display - rave_lcd
async function rave_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value) {
await BrainPad.Display.DrawTextScale("BrainSense", 0xf08000, 20, 10, 2, 2);
let y = 35;
let x = 5;
await BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
await BrainPad.Display.DrawTextScale("LIGHT= ", 0x00ff00, x, y + 20, 1, 2);
await BrainPad.Display.DrawTextScale("SOIL= ", 0x00ff00, x, y + 40, 1, 2);
await BrainPad.Display.DrawText("Celsius= ", 0xf0ff00, x + 40, y + 60);
await BrainPad.Display.DrawText(temperature_sensor_value.toString(), 0xf0ff00, x + 100, y + 60);
await BrainPad.Display.DrawText("Humidity= ", 0x00fff0, x + 40, y + 70);
await BrainPad.Display.DrawText(humidity_sensor_value.toString(), 0x00fff0, x + 100, y + 70);
let width = 103;
let height = 12;
y = 36;
x = 42;
// Drawing the white rectangles
for (let n = 0; n < 3; n++) {
await BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height);
await BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4);
y += 20;
}
x = 44;
y = 38;
height = 8;
// Drawing the bars based on sensor values
await BrainPad.Display.DrawFillRect(0xf08000, x, y, steam_sensor_value, height);
await BrainPad.Display.DrawFillRect(0xf08000, x, y + 20, light_sensor_value, height);
await BrainPad.Display.DrawFillRect(0xf08000, x, y + 40, soilMoisture_sensor_value, height);
}
// Main loop
async function mainLoop() {
while (true) {
await BrainPad.Display.Clear(0);
let steam_sensor_value = await BrainPad.Analog.Read(steam_pin);
let light_sensor_value = await BrainPad.Analog.Read(photo_pin);
let soilMoisture_sensor_value = await BrainPad.Analog.Read(soil_pin);
let temperature_sensor_value = await BrainPad.Temperature.Read(temp_humid_pin, 11);
let humidity_sensor_value = await BrainPad.Humidity.Read(temp_humid_pin, 11);
await rave_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value);
await BrainPad.Display.Show();
}
}
// Call the main loop function
mainLoop();
Smart Home Full Demo with Dynamic Dashboard
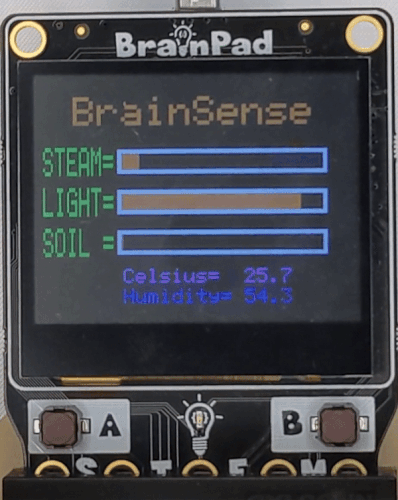
Let’s revisit the previous lesson where we wrote a full demo of BrainSense with BrainPad Pulse and we need to seamlessly transition it to the BrainPad Rave. We’ll integrate the new dashboard featuring dynamic bars.
Since we employed Screen instructions as Subroutines, akin to methods and functions, we can write both subroutines for Pulse and Rave actions. In the main loop, we’ll call the appropriate method (line 41) based on the microcomputer, ensuring compatibility with both BrainSense devices.
Python
from DUELink.DUELinkController import DUELinkController
available_port = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(available_port)
# Initialize sensor pins
pir_pin = 3
flame_pin = 4
steam_pin = 5
temp_humid_pin = 6
photo_pin = 0
soil_pin = 1
# Initialize actuator pins
servo_pin = 10
fan_pin = 11
led_pin = 12
horn_pin = 13
# Function for movement based on PIR sensor
def move(motion_sensor_value):
if not motion_sensor_value:
BrainPad.Servo.Set(servo_pin, 0)
else:
BrainPad.Servo.Set(servo_pin, 90)
# Function for steam sensor action
def steam(steam_sensor_value):
if steam_sensor_value > 70:
BrainPad.Analog.Write(fan_pin, 50)
for i in range(0, 4):
BrainPad.Neo.SetColor(i, 0x0000FF)
BrainPad.Neo.Show(led_pin, 4)
# Function for flame sensor action
def flame(flame_sensor_value):
if flame_sensor_value == 0:
BrainPad.Servo.Set(servo_pin, 90)
for n in range(400, 501, 5):
BrainPad.System.Beep(horn_pin, n, 20)
BrainPad.Neo.SetColor(0, 0xFF0000)
BrainPad.Neo.SetColor(1, 0x0000FF)
BrainPad.Neo.SetColor(2, 0xFF0000)
BrainPad.Neo.SetColor(3, 0x0000FF)
BrainPad.Neo.Show(led_pin, 4)
BrainPad.Neo.Clear()
BrainPad.Neo.Show(led_pin, 4)
# Function for light sensor action
def light(light_sensor_value):
if light_sensor_value <= 85:
for i in range(0, 4):
BrainPad.Neo.SetColor(i, 0xFFC800)
# Function for soil moisture sensor action
def soil(soilMoisture_sensor_value):
if soilMoisture_sensor_value < 10:
for n in range(100, 151, 50):
BrainPad.System.Beep(horn_pin, n, 20)
BrainPad.Neo.SetColor(0, 0xFF00FF)
BrainPad.Neo.SetColor(1, 0x00FF00)
BrainPad.Neo.SetColor(2, 0xFF00FF)
BrainPad.Neo.SetColor(3, 0x00FF00)
BrainPad.Neo.Show(led_pin, 4)
BrainPad.Neo.Clear()
BrainPad.Neo.Show(led_pin, 4)
# Function to update LCD display
def rave_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value):
BrainPad.Display.DrawTextScale("BrainSense",0xf08000,20,10,2,2)
y=35
x=5
BrainPad.Display.DrawTextScale("STEAM= ",0x00ff00, x, y,1,2)
BrainPad.Display.DrawTextScale("LIGHT= ", 0x00ff00, x, y+20,1,2)
BrainPad.Display.DrawTextScale("SOIL= ", 0x00ff00, x, y+40,1,2)
BrainPad.Display.DrawText("Celsius= ", 0xf0ff00, x+40, y+60)
BrainPad.Display.DrawText(float(temperature_sensor_value), 0xf0ff00, x + 100, y+60)
BrainPad.Display.DrawText("Humidity= ", 0x00fff0, x+40, y+70)
BrainPad.Display.DrawText(float(humidity_sensor_value), 0x00fff0, x + 100,y+70)
width = 103
height = 12
y = 36
x = 42
# drawing the white rectangles
for n in range(0,3):
BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height)
BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4)
y = y + 20
x = 44
y = 38
height = 8
# drawing the bars based on sensors values
BrainPad.Display.DrawFillRect(0xf08000, x, y, steam_sensor_value, height)
BrainPad.Display.DrawFillRect(0xf08000, x, y + 20, light_sensor_value, height)
BrainPad.Display.DrawFillRect(0xf08000, x, y + 40, soilMoisture_sensor_value, height)
# Function to main LCD display
def pulse_lcd(steam_sensor_value,light_sensor_value,soilMoisture_sensor_value,temperature_sensor_value,humidity_sensor_value):
BrainPad.Display.DrawTextScale("BrainSense",1,3,0,2,1)
y=15
x=5
BrainPad.Display.DrawText("STEAM= ",1,x,y)
BrainPad.Display.DrawText(int(steam_sensor_value),1,x+60,y)
BrainPad.Display.DrawText("LIGHT= ", 1, x, y+10)
BrainPad.Display.DrawText(int(light_sensor_value), 1, x + 60, y+10)
BrainPad.Display.DrawText("SOIL= ", 1, x, y+20)
BrainPad.Display.DrawText(int(soilMoisture_sensor_value), 1, x + 60, y+20)
BrainPad.Display.DrawText("Celsius= ", 1, x, y+30)
BrainPad.Display.DrawText(float(temperature_sensor_value), 1, x + 60, y+30)
BrainPad.Display.DrawText("Humidity= ", 1, x, y+40)
BrainPad.Display.DrawText(float(humidity_sensor_value), 1, x + 60, y+40)
# Implement LCD text display
# Main loop
while True:
BrainPad.Display.Clear(0)
BrainPad.Neo.Clear()
motion_sensor_value = BrainPad.Digital.Read(pir_pin,1)
steam_sensor_value = BrainPad.Analog.Read(steam_pin)
flame_sensor_value = BrainPad.Digital.Read(flame_pin,1)
light_sensor_value = BrainPad.Analog.Read(photo_pin)
soilMoisture_sensor_value = BrainPad.Analog.Read(soil_pin)
temperature_sensor_value = BrainPad.Temperature.Read(temp_humid_pin, 11)
humidity_sensor_value = BrainPad.Humidity.Read(temp_humid_pin, 11)
BrainPad.Analog.Write(fan_pin, 0)
rave_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value)
#pulse_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value)
move(motion_sensor_value)
steam(steam_sensor_value)
flame(flame_sensor_value)
light(light_sensor_value)
soil(soilMoisture_sensor_value)
BrainPad.Display.Show()
BrainPad.Neo.Show(led_pin,4)
C#
using GHIElectronics.DUELink;
namespace BrainSense
{
class Program
{
public static class Globals
{
// Initialize sensor pins
public static int pir_pin = 3;
public static int flame_pin = 4;
public static int steam_pin = 5;
public static int temp_humid_pin = 6;
public static int photo_pin = 0;
public static int soil_pin = 1;
// Initialize actuator pins
public static int servo_pin = 10;
public static int fan_pin = 11;
public static int led_pin = 12;
public static int horn_pin = 13;
}
// Main loop
static void Main(string[] args)
{
DUELinkController BrainPad = new DUELinkController(DUELinkController.GetConnectionPort());
while (true)
{
BrainPad.Display.Clear(0);
BrainPad.Neo.Clear();
bool motion_sensor_value = BrainPad.Digital.Read(Globals.pir_pin, 1);
double steam_sensor_value = BrainPad.Analog.Read(Globals.steam_pin); steam_sensor_value = (double)System.Math.Round(steam_sensor_value, 2);
bool flame_sensor_value = BrainPad.Digital.Read(Globals.flame_pin, 1);
double light_sensor_value = BrainPad.Analog.Read(Globals.photo_pin); light_sensor_value = (double)System.Math.Round(light_sensor_value, 2);
double soilMoisture_sensor_value = BrainPad.Analog.Read(Globals.soil_pin); soilMoisture_sensor_value = (double)System.Math.Round(soilMoisture_sensor_value, 2);
double temperature_sensor_value = BrainPad.Temperature.Read(Globals.temp_humid_pin, 11); temperature_sensor_value = (double)System.Math.Round(temperature_sensor_value, 2);
double humidity_sensor_value = BrainPad.Humidity.Read(Globals.temp_humid_pin, 11); humidity_sensor_value = (double)System.Math.Round(humidity_sensor_value, 2);
BrainPad.Analog.Write(Globals.fan_pin, 0);
RaveLCD(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value, BrainPad);
// PulseLCD(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value, BrainPad);
Move(motion_sensor_value, BrainPad);
Steam(steam_sensor_value, BrainPad);
Flame(flame_sensor_value, BrainPad);
Light(light_sensor_value, BrainPad);
Soil(soilMoisture_sensor_value, BrainPad);
BrainPad.Display.Show();
BrainPad.Neo.Show(Globals.led_pin, 4);
}
}
// Function for movement based on PIR sensor
static void Move(bool motion_sensor_value, DUELinkController BrainPad)
{
if (!motion_sensor_value)
{
BrainPad.Servo.Set(Globals.servo_pin, 0);
}
else
{
BrainPad.Servo.Set(Globals.servo_pin, 90);
}
}
// Function for steam sensor action
static void Steam(double steam_sensor_values, DUELinkController BrainPad)
{
if (steam_sensor_values > 70)
{
BrainPad.Analog.Write(Globals.fan_pin, 50);
for (int i = 0; i < 4; i++)
{
BrainPad.Neo.SetColor(i, 0x0000FF);
}
BrainPad.Neo.Show(12, 4);
}
}
// Function for flame sensor action
static void Flame(bool flame_sensor_value, DUELinkController BrainPad)
{
if (!flame_sensor_value)
{
BrainPad.Servo.Set(Globals.servo_pin, 90);
for (int n = 400; n <= 500; n += 5)
{
BrainPad.System.Beep(Globals.horn_pin, (uint)n, 20);
BrainPad.Neo.SetColor(0, 0xFF0000);
BrainPad.Neo.SetColor(1, 0x0000FF);
BrainPad.Neo.SetColor(2, 0xFF0000);
BrainPad.Neo.SetColor(3, 0x0000FF);
BrainPad.Neo.Show(Globals.led_pin, 4);
BrainPad.Neo.Clear();
BrainPad.Neo.Show(Globals.led_pin, 4);
}
}
}
// Function for light sensor action
static void Light(double light_sensor_value, DUELinkController BrainPad)
{
if (light_sensor_value <= 85)
{
for (int i = 0; i < 4; i++)
{
BrainPad.Neo.SetColor(i, 0xFFC800);
}
}
}
// Function for soil moisture sensor action
static void Soil(double soilMoisture_sensor_value, DUELinkController BrainPad)
{
if (soilMoisture_sensor_value < 10)
{
for (int n = 100; n <= 150; n += 50)
{
BrainPad.System.Beep(Globals.horn_pin, (uint)(int)n, 20);
BrainPad.Neo.SetColor(0, 0xFF00FF);
BrainPad.Neo.SetColor(1, 0x00FF00);
BrainPad.Neo.SetColor(2, 0xFF00FF);
BrainPad.Neo.SetColor(3, 0x00FF00);
BrainPad.Neo.Show(Globals.led_pin, 4);
BrainPad.Neo.Clear();
BrainPad.Neo.Show(Globals.led_pin, 4);
}
}
}
// Function to update LCD display - rave_lcd
static void RaveLCD(double steam_sensor_value, double light_sensor_value, double soilMoisture_sensor_value, double temperature_sensor_value, double humidity_sensor_value, DUELinkController BrainPad)
{
BrainPad.Display.DrawTextScale("BrainSense", 0xf08000, 20, 10, 2, 2);
int y = 35;
int x = 5;
BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
BrainPad.Display.DrawTextScale("LIGHT= ", 0x00ff00, x, y + 20, 1, 2);
BrainPad.Display.DrawTextScale("SOIL= ", 0x00ff00, x, y + 40, 1, 2);
BrainPad.Display.DrawText("Celsius= ", 0xf0ff00, x + 40, y + 60);
BrainPad.Display.DrawText(temperature_sensor_value.ToString(), 0xf0ff00, x + 100, y + 60);
BrainPad.Display.DrawText("Humidity= ", 0x00fff0, x + 40, y + 70);
BrainPad.Display.DrawText(humidity_sensor_value.ToString(), 0x00fff0, x + 100, y + 70);
int width = 103;
int height = 12;
y = 36;
x = 42;
// drawing the white rectangles
for (int n = 0; n < 3; n++)
{
BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height);
BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4);
y = y + 20;
}
x = 44;
y = 38;
height = 8;
// drawing the bars based on sensors values
BrainPad.Display.DrawFillRect(0xf08000, x, y, (int)steam_sensor_value, height);
BrainPad.Display.DrawFillRect(0xf08000, x, y + 20, (int)light_sensor_value, height);
BrainPad.Display.DrawFillRect(0xf08000, x, y + 40, (int)soilMoisture_sensor_value, height);
}
// Function to update LCD display - pulse_lcd
static void PulseLCD(double steam_sensor_value, double light_sensor_value, double soilMoisture_sensor_value, double temperature_sensor_value, double humidity_sensor_value, DUELinkController BrainPad)
{
BrainPad.Display.DrawTextScale("BrainSense", 1, 3, 0, 2, 1);
int y = 15;
int x = 5;
BrainPad.Display.DrawText("STEAM= ", 1, x, y);
BrainPad.Display.DrawText(steam_sensor_value.ToString(), 1, x + 60, y);
BrainPad.Display.DrawText("LIGHT= ", 1, x, y + 10);
BrainPad.Display.DrawText(light_sensor_value.ToString(), 1, x + 60, y + 10);
BrainPad.Display.DrawText("SOIL= ", 1, x, y + 20);
BrainPad.Display.DrawText(soilMoisture_sensor_value.ToString(), 1, x + 60, y + 20);
BrainPad.Display.DrawText("Celsius= ", 1, x, y + 30);
BrainPad.Display.DrawText(temperature_sensor_value.ToString(), 1, x + 60, y + 30);
BrainPad.Display.DrawText("Humidity= ", 1, x, y + 40);
BrainPad.Display.DrawText(humidity_sensor_value.ToString(), 1, x + 60, y + 40);
}
}
}
JS
import { SerialUSB } from './serialusb.js';
import * as due from './duelink.js';
let BrainPad = new due.DUELinkController(new SerialUSB());
await BrainPad.Connect();
// Initialize sensor pins
const pir_pin = 3;
const flame_pin = 4;
const steam_pin = 5;
const temp_humid_pin = 6;
const photo_pin = 0;
const soil_pin = 1;
// Initialize actuator pins
const servo_pin = 10;
const fan_pin = 11;
const led_pin = 12;
const horn_pin = 13;
// Function for movement based on PIR sensor
async function move(motion_sensor_value) {
if (!motion_sensor_value)
await BrainPad.Servo.Set(servo_pin, 0);
else
await BrainPad.Servo.Set(servo_pin, 90);
}
// Function for steam sensor action
async function steam(steam_sensor_value) {
if (steam_sensor_value >= 70) {
BrainPad.Analog.Write(fan_pin, 50);
for (let i = 0; i < 4; i++) {
await BrainPad.Neo.SetColor(i, 0x0000FF);
}
await BrainPad.Neo.Show(led_pin, 4);
}
}
// Function for flame sensor action
async function flame(flame_sensor_value) {
if (!flame_sensor_value) {
await BrainPad.Servo.Set(servo_pin, 90);
for (let n = 400; n <= 500; n += 5) {
await BrainPad.System.Beep(horn_pin, n, 20);
await BrainPad.Neo.SetColor(0, 0xFF0000)
await BrainPad.Neo.SetColor(1, 0x0000FF)
await BrainPad.Neo.SetColor(2, 0xFF0000)
await BrainPad.Neo.SetColor(3, 0x0000FF)
await BrainPad.Neo.Show(led_pin, 4);
await BrainPad.Neo.Clear();
await BrainPad.Neo.Show(led_pin, 4);
}
}
}
// Function for light sensor action
async function light(light_sensor_value) {
if (light_sensor_value <= 85) {
for (let i = 0; i < 4; i++) {
await BrainPad.Neo.SetColor(i, 0xFFC800);
}
await BrainPad.Neo.Show(led_pin, 4);
}
}
// Function for soil moisture sensor action
async function soil(soilMoisture_sensor_value) {
if (soilMoisture_sensor_value < 10) {
for (let n = 100; n <= 150; n += 50) {
await BrainPad.System.Beep(horn_pin, n, 20);
await BrainPad.Neo.SetColor(0, 0xFF00FF)
await BrainPad.Neo.SetColor(1, 0x00FF00)
await BrainPad.Neo.SetColor(2, 0xFF00FF)
await BrainPad.Neo.SetColor(3, 0x00FF00)
await BrainPad.Neo.Show(led_pin, 4);
await BrainPad.Neo.Clear();
await BrainPad.Neo.Show(led_pin, 4);
}
}
}
// Function to update LCD display - rave_lcd
async function rave_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value) {
await BrainPad.Display.DrawTextScale("BrainSense", 0xf08000, 20, 10, 2, 2);
let y = 35;
let x = 5;
await BrainPad.Display.DrawTextScale("STEAM= ", 0x00ff00, x, y, 1, 2);
await BrainPad.Display.DrawTextScale("LIGHT= ", 0x00ff00, x, y + 20, 1, 2);
await BrainPad.Display.DrawTextScale("SOIL= ", 0x00ff00, x, y + 40, 1, 2);
await BrainPad.Display.DrawText("Celsius= ", 0xf0ff00, x + 40, y + 60);
await BrainPad.Display.DrawText(temperature_sensor_value.toString(), 0xf0ff00, x + 100, y + 60);
await BrainPad.Display.DrawText("Humidity= ", 0x00fff0, x + 40, y + 70);
await BrainPad.Display.DrawText(humidity_sensor_value.toString(), 0x00fff0, x + 100, y + 70);
let width = 103;
let height = 12;
y = 36;
x = 42;
// Drawing the white rectangles
for (let n = 0; n < 3; n++) {
await BrainPad.Display.DrawFillRect(0xffffff, x, y, width, height);
await BrainPad.Display.DrawFillRect(0x000000, x + 2, y + 2, width - 4, height - 4);
y += 20;
}
x = 44;
y = 38;
height = 8;
// Drawing the bars based on sensor values
await BrainPad.Display.DrawFillRect(0xf08000, x, y, steam_sensor_value, height);
await BrainPad.Display.DrawFillRect(0xf08000, x, y + 20, light_sensor_value, height);
await BrainPad.Display.DrawFillRect(0xf08000, x, y + 40, soilMoisture_sensor_value, height);
}
// Function to update LCD display - pulse_lcd
async function pulse_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value) {
await BrainPad.Display.DrawTextScale("BrainSense", 1, 3, 0, 2, 1)
let y = 15
let x = 5
await BrainPad.Display.DrawText("STEAM= ", 1, x, y)
await BrainPad.Display.DrawText(steam_sensor_value.toString(), 1, x + 60, y)
await BrainPad.Display.DrawText("LIGHT= ", 1, x, y + 10)
await BrainPad.Display.DrawText(light_sensor_value.toString(), 1, x + 60, y + 10)
await BrainPad.Display.DrawText("SOIL= ", 1, x, y + 20)
await BrainPad.Display.DrawText(soilMoisture_sensor_value.toString(), 1, x + 60, y + 20)
await BrainPad.Display.DrawText("Celsius= ", 1, x, y + 30)
await BrainPad.Display.DrawText(temperature_sensor_value.toString(), 1, x + 60, y + 30)
await BrainPad.Display.DrawText("Humidity= ", 1, x, y + 40)
await BrainPad.Display.DrawText(humidity_sensor_value.toString(), 1, x + 60, y + 40)
}
// Main loop
async function mainLoop() {
while (true) {
await BrainPad.Display.Clear(0);
await BrainPad.Neo.Clear();
let motion_sensor_value = await BrainPad.Digital.Read(pir_pin, 1);
let steam_sensor_value = await BrainPad.Analog.Read(steam_pin);
let flame_sensor_value = await BrainPad.Digital.Read(flame_pin, 1);
let light_sensor_value = await BrainPad.Analog.Read(photo_pin);
let soilMoisture_sensor_value = await BrainPad.Analog.Read(soil_pin);
let temperature_sensor_value = await BrainPad.Temperature.Read(temp_humid_pin, 11);
let humidity_sensor_value = await BrainPad.Humidity.Read(temp_humid_pin, 11);
await BrainPad.Analog.Write(fan_pin, 0);
await rave_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value);
//await pulse_lcd(steam_sensor_value, light_sensor_value, soilMoisture_sensor_value, temperature_sensor_value, humidity_sensor_value);
await move(motion_sensor_value);
await steam(steam_sensor_value);
await flame(flame_sensor_value);
await light(light_sensor_value);
await soil(soilMoisture_sensor_value);
await BrainPad.Display.Show();
await BrainPad.Neo.Show(led_pin, 4);
}
}
// Call the main loop function
mainLoop();
What’s Next?
let’s modify our data visualization to present the bars in a vertical orientation. This adjustment will provide a different visual perspective, allowing for a representation of the sensor data on the BrainPad Rave LCD.
BrainStorm
Certainly! With the touch capability of the BrainPad Rave LCD using the Touch API, we can design a dynamic and interactive dashboard for our smart home. Users can intuitively control and visualize data by incorporating touch-based controls.
For example, tapping on a specific area could toggle a device, change a setting, or provide more detailed information about a sensor. This interactive approach adds a layer of user-friendly functionality, enhancing the overall experience of managing and monitoring the smart home environment.