BrainGamer Pong
Pong was one of the first video games ever created back in 1972. We’ll build the game step-by-step and use the BrainGamer for its ANALOG Rocker & DIGITAL Buttons to control the paddles.
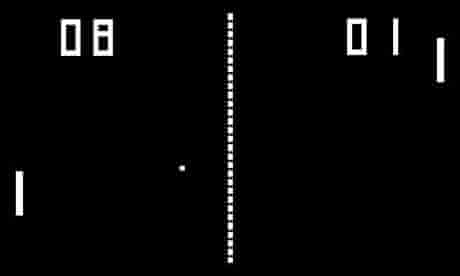
Prerequisites
You must have a basic understanding of coding and read the BrainGamer Intro and have completed the Drawing lesson.
Creating the players
We’ll make this game so 2 people can play, one player will use the Rocker Switch and the other player can use the Buttons on the BrainGamer. We’ll use the LcdFill() function to make both players. Since the paddles only move up and down, we’ll need one variable for each player to track this position. We can’t use L and R because they are used in the driver. So, let’s use A and B instead. Let’s create an @Start subroutine that we can call later to reset our game when the game is over.
Place the FillRect() functions inside a while loop so we can check the position every time the program loops. Let’s also LcdClear() the screen at the top of the while loop and LcdShow() the screen at the bottom.
@Start
a = 30 #Left Player Position
b = 30 #Right Player Position
@Loop
LcdClear(0)
LcdFill(1,10, a, 2, 10) #Draw Left Paddle
LcdFill(1,120, b, 2, 10) #Draw Right Paddle
LcdShow()
Wait(10)
Goto Loop
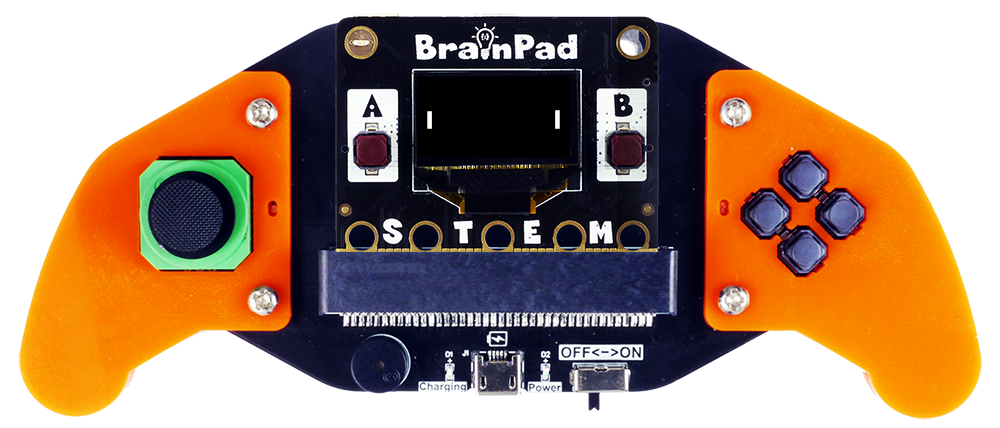
Creating the Net
Let’s create the playing field. To start we’ll need to create a net. We can do this by using the Line() function inside of a loop. We’ll also change the value of the net by adding 5 to it. This creates spaces in the net so it looks more like the original game.
@Start
a = 30 #Left Player Position
b = 30 #Right Player Position
@Loop
LcdClear(0)
LcdFill(1, 10, a, 2, 10) #Draw Left Paddle
LcdFill(1, 120, b, 2, 10) #Draw Right Paddle
For n = 0 to 64 step 10 #Draw Net
LcdLine(1,64,n,64,n+5)
Next
LcdShow()
Wait(10)
Goto Loop
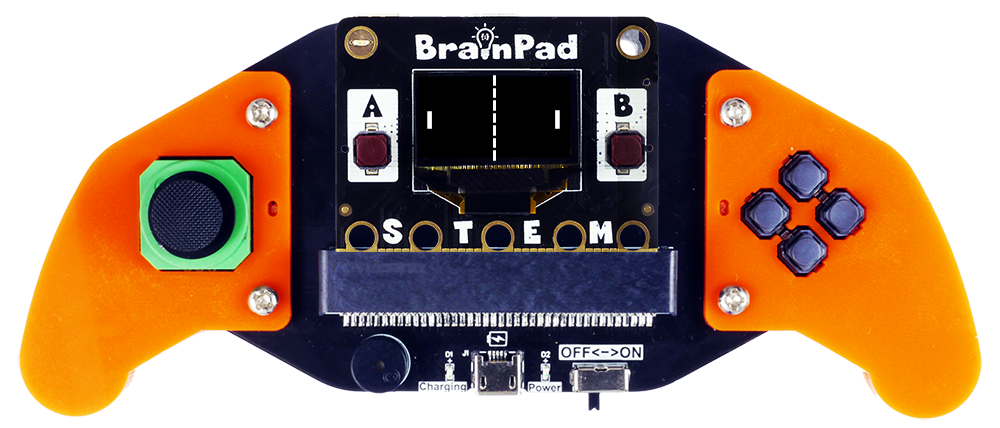
Moving the Players
Let’s start by moving the right player first. We’ll use the DIGITAL buttons on the BrainGamer for this. We will also add code to keep the paddle on the screen. Place this code just about where we created the right paddle using LcdFill().
Keys() #Check Key Press
if u = 0:b = b - 4:End #Move Right Paddle Up
if d = 0:b = b + 4:End #Move Right Paddle Down
if b < 5:b = 5:End #Right Paddle Hits Top
if b > 50:b = 50:End #Right Paddle Hits Bottom
LcdFill(1,120,b,2,10) #Draw Right Paddle
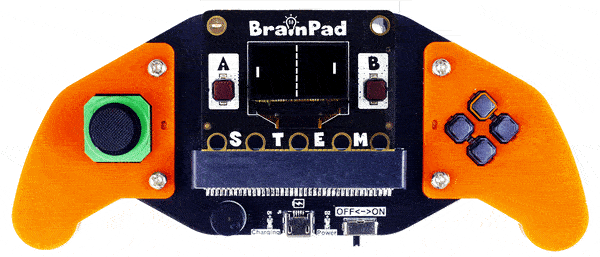
To move left player we’ll use the ANALOG Rocker switch on the BrainGamer. Place this code just above
Rocker() #Check Rocker Move
if y < 40:a = a - 4:End #Move Left Paddle Up
if y > 60:a = a + 4:End #Move Left Paddle Down
if a < 5:a = 5:End #Left Paddle Hits Top
if a > 50:a = 50:End #Left Paddle Hits Bottom
LcdFill(1, 10, a, 2, 10) #Draw Left Paddle
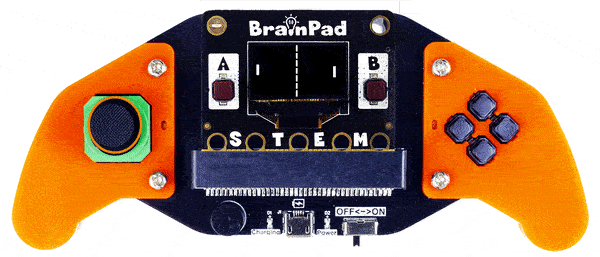
When we play our game, it will be DIGITAL (buttons) vs ANALOG (rocker). Which do you like better? If you’d like to know more about Digital or Analog, check out their respective lessons.
Here is the complete code we have created so far.
@Start
a = 30 #Left Player Position
b = 30 #Right Player Position
@Loop
LcdClear(0)
Rocker() #Check Rocker Move
if y < 40:a = a - 4:End #Move Left Paddle Up
if y > 60:a = a + 4:End #Move Left Paddle Down
if a < 5:a = 5:End #Left Paddle Hits Top
if a > 50:a = 50:End #Left Paddle Hits Bottom
LcdFill(1, 10, a, 2, 10) #Draw Left Paddle
Keys() #Check Key Press
if u = 0:b = b - 4:End #Move Right Paddle Up
if d = 0:b = b + 4:End #Move Right Paddle Down
if b < 5:b = 5:End #Right Paddle Hits Top
if b > 50:b = 50:End #Right Paddle Hits Bottom
LcdFill(1,120,b,2,10) #Draw Right Paddle
For n = 0 to 64 step 10 #Draw Net
LcdLine(1,64,n,64,n+5)
Next
LcdShow()
Wait(10)
Goto Loop
#####################################
### BrainGamer Driver ###
### YOUR CODE GOES ABOVE THIS BOX ###
#####################################
### variables used by driver ###
### x,y,u,d,l,r ###
#####################################
Exit
### Rocker ###
# Reads the rocker position into x and y
@Rocker
x = ARead(4)
y = ARead(3)
Return
### Activate Vibrator ###
@Von
DWrite(8,0)
Return
### Deactivate Vibrator ###
@Voff
DWrite(8,1)
Return
### Read Keypad ####
# Reads the 4 buttons into U,D,L,R
@Keys
u = DRead(14,1)
d = DRead(15,1)
l = DRead(13,1)
r = DRead(16,1)
Return
Adding a ball
We’ve got our players moving and we created a net. We can’t play without a ball, so let’s add that now. First we need variables to keep track of the coordinates and speed of the ball.
We will use G/H/I/K for:
- G: Ball x
- H: Ball x direction
- I: Ball y
- J: Ball y direction
Now that we’ve decided on the variables to track our ball, we can add some code to move it and draw it.
Add this code to the very top to of the code. This will initialize the variables with useful values.
g = 10 #Ball x
h = 2.3 #Ball x direction
i = 10 #Ball y
j = 2.6 #Ball y direction
Add the following inside the loop, right below LcdClear().
g = g + h #Move Ball X
i = i + j #Move Ball Y
LcdFill(1, g, i, 4, 4) #Draw Ball
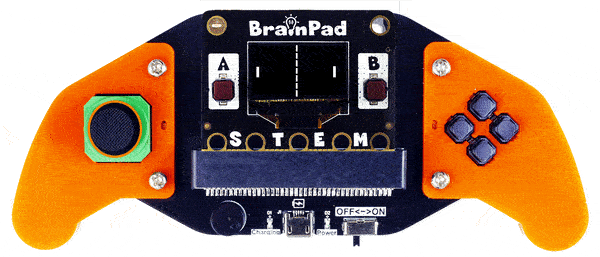
Bouncing off the Walls
Our ball moves, except it moves right off the screen! We need to add an if statement to detect when the ball hits the top or bottom wall and then bounces off it.
g = g + h #Move Ball X
i = i + j #Move Ball Y
If i < 5 || i> 55: #Bounce off top or bottom walls
j= j* -1
End
LcdFill(1, g, i, 4, 4) #Draw Ball
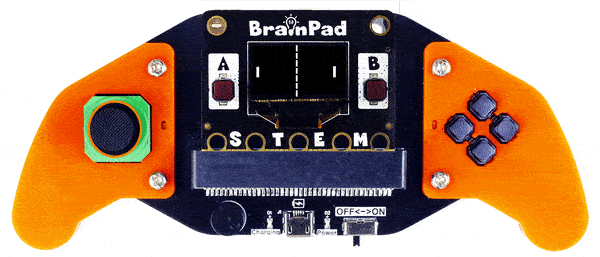
Collision Detection
Now our ball bounces off the walls. Next, we must add some code to detect when the ball hits a player’s paddle. Using an if statement, let’s detect the player2 side first. We only want to check when the ball is near the paddle. So we create an if statement to check ballPosX position, then we’ll use another if statement inside that to see if the ball hits the player2 paddle.
Let’s also add an else condition to our second if statement to do something if the ball is missed. Remember that the comments added here will not interfere with the code.
if g > 118:
if i >= (b - 1) && i <= (b + 12)
#Add code here when player hits ball
h = h* -1
else
#Add code here when player misses
g = 15 #Reset the Ball position
End
End
Before we add what happens when we miss the ball, let’s add the code to detect hitting the player1 paddle just below the player2 code we just created. Let’s also add:
if g > 118:
if i >= (b - 1) && i <= (b + 12)
#Add code here when player hits ball
h = h* -1
else
#Add code here when player misses
g = 15 #Reset the Ball position
End
End
if g < 12:
if i >= (a - 1) && i <= (a + 12)
#Add code here when player misses
h = h* -1
else
#Add code here when player misses
g = 115 #Reset the Ball position
End
End
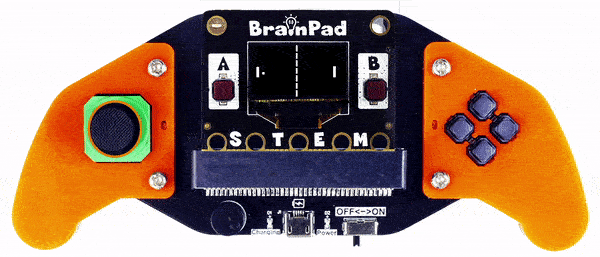
Now that we have the basic functionality we can add scoring to the game. We’ll come back and increase the score based on which player misses the ball, but first let’s create those variables. Place the following with the other variables near the top of our code that we created earlier.
s = 0 #Player1 Score
t = 0 #Player2 Score
We have the variables for score, now let’s show them on the screen. Add LcdText() function code just above the LcdShow() function at the bottom of our @Loop.
LcdText(str(s),1, 50, 5)
LcdText(str(t),1, 74, 5)
LCDShow()
Wait(10)
Let’s increase the score of a player when the other player misses. We’ll do this inside the else condition in the if statement we created to detect collision, where we placed our Add Code comments. Let’s also add a sound using Beep() so we know when a player scores, and vibrate the BrainGamer when they miss by using VOn() and VOff() to control the vibration motor.
if g > 118:
if i >= (b - 1) && i <= (b + 12)
#Add code here when player hits ball
h = h* -1
Beep('P',256,100)
else
#Add code here when player misses
s = s + 1
g = 15
Von()#Vibrate BrainGamer
Wait(100)
Voff()#Turn off Vibrate
End
End
if g < 12:
if i >= (a - 1) && i <= (a + 12)
#Add code here when player hits ball
h = h* -1
Beep('P',256,100)
else
#Add code here when player misses
t=t+1
g=115
Von()#Vibrate BrainGamer
Wait(100)
Voff()#Turn off Vibrate
End
End
Winning the Game
Now let’s add some code to find a winner. If any player reaches 5, they win. We’ll create a variable called W , place it near the top with our other variables and set it to 0. We’ll set it to 1 when someone has won the game. Then we’ll use that variable to tell us when to play a sound. Add this code just above our Show() and Wait() functions at the bottom of the while loop.
w = 0 #Check for winner
if s = 5
LcdClear(0)
LcdTextS("Player 1",1,5,0,2,2)
LcdTextS("WINS!",1,25,20,2,2)
LcdShow()
w=1
Wait(3000)
End
if t = 5
LcdClear(0)
LcdTextS("Player 2",1,5,0,2,2)
LcdTextS("WINS!",1,25,20,2,2)
LcdShow()
w=1
Wait(3000)
End
Finally, let’s use the W variable to tell us when to play a victory tune. We can add this at the very bottom of the @Loop subroutine. At the end of the if-statement, we’ll send the program back to Start() to reset our game.
if w = 1 #If winner play sound and restart
Beep('P',256,100)
Beep('P',200,100)
Beep('P',256,100)
Wait(2000)
Goto Start
End
Final Results
Congratulations, you’ve coded Pong…the classic first video game ever created!
@Start
a = 30 #Left Player Position
b = 30 #Right Player Position
g = 10 #Ball x
h = 2.3 #Ball x direction
i = 10 #Ball y
j = 2.6 #Ball y direction
s = 0 #Player1 Score
t = 0 #Player2 Score
w = 0 #Check Win
@Loop
LcdClear(0)
g = g + h #Move Ball X
i = i + j #Move Ball Y
if i < 5 || i> 55: #Bounce off top or bottom walls
j = j* -1
End
LcdFill(1, g, i, 4, 4) #Draw Ball
if g > 118:
if i >= (b - 1) && i <= (b + 12)
#Add code here when player hits ball
h = h* -1
Beep('P',256,100)
else
#Add code here when player misses
s = s + 1
g = 15
Von()#Vibrate BrainGamer
Wait(100)
Voff()#Turn off Vibrate
End
End
if g < 12:
if i >= (a - 1) && i <= (a + 12)
#Add code here when player hits ball
h = h* -1
Beep('P',256,100)
else
#Add code here when player misses
t=t+1
g=115
Von()#Vibrate BrainGamer
Wait(100)
Voff()#Turn off Vibrate
End
End
Rocker() #Check Rocker Move
if y < 40:a = a - 4:End #Move Left Paddle Up
if y > 60:a = a + 4:End #Move Left Paddle Down
if a < 5:a = 5:End #Left Paddle Hits Top
if a > 50:a = 50:End #Left Paddle Hits Bottom
LcdFill(1, 10, a, 2, 10) #Draw Left Paddle
Keys() #Check Key Press
if u = 0:b = b - 4:End #Move Right Paddle Up
if d = 0:b = b + 4:End #Move Right Paddle Down
if b < 5:b = 5:End #Right Paddle Hits Top
if b > 50:b = 50:End #Right Paddle Hits Bottom
LcdFill(1,120,b,2,10) #Draw Right Paddle
For n = 0 to 64 step 10 #Draw Net
LcdLine(1,64,n,64,n+5)
Next
if s = 5
LcdClear(0)
LcdTextS("Player 1",1,5,0,2,2)
LcdTextS("WINS!",1,25,20,2,2)
LcdShow()
w=1
Wait(3000)
End
if t = 5
LcdClear(0)
LcdTextS("Player 2",1,5,0,2,2)
LcdTextS("WINS!",1,25,20,2,2)
LcdShow()
w=1
Wait(3000)
End
if w = 1 #If winner play sound and restart
Beep('P',256,100)
Beep('P',200,100)
Beep('P',256,100)
Wait(2000)
Goto Start
End
LcdText(str(s),1, 50, 5);
LcdText(str(t),1, 74, 5);
LcdShow()
Wait(10)
Goto Loop
#####################################
### BrainGamer Driver ###
#####################################
### variables used by driver ###
### x,y,u,d,l,r ###
#####################################
Exit
### Rocker ###
# Reads the rocker position into x and y
@Rocker
x = ARead(4)
y = ARead(3)
Return
### Activate Vibrator ###
@Von
DWrite(8,0)
Return
### Deactivate Vibrator ###
@Voff
DWrite(8,1)
Return
### Read Keypad ####
# Reads the 4 buttons into U,D,L,R
@Keys
u = DRead(14,1)
d = DRead(15,1)
l = DRead(13,1)
r = DRead(16,1)
Return
What’s Next?
Can you give the game Artificial Intelligence and let one of the player be the BrainPad?
BrainStorm
Typically, Video Games require a lot of processing power. Any basic modern computer can surf the web and send emails but modern video games require very sophisticated computers, with special graphics card. We find it silly that you need a basic computer to do work but then you need an advanced computer to play!