Countdown Timer
This lesson will create a countdown timer. This can remind you to check on the pizza in the oven or to get off the computer and take a little walk!
Prerequisite
You must have a basic understanding of coding and have completed the Drawing lesson.
The Plan
A good countdown timer will have a way to set the time and a start button. Button A will be used to add 10 seconds when it is pushed. Button B will start the countdown.
The Countdown
We need an easy way to see counter, so it will need large digits.
c = 12345
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
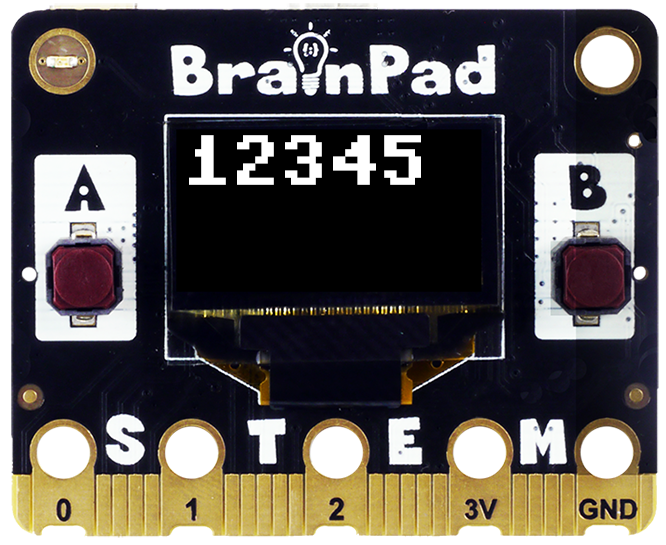
We can now add a loop to start counting down. We also need a one second delay so we are counting down once a second.
c = 12345
@Loop
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
c=c-1
Wait(1000)
Goto Loop
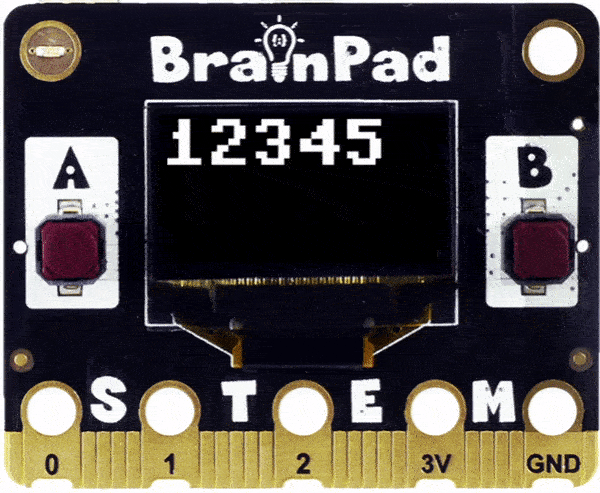
Instead of just starting immediately, we will create a loop that waits for the Button A to be pressed. In other words, keep looping as long as the button is not pressed. This loop will not do anything besides sit there waiting for the button to be pressed. Since we have nothing to do, we will Wait(0.01) in the loop.
Once button A is pressed, the loop will end and the next statement will be run, which is another loop; but this second loop is counting down.
c = 12345
BtnEnable('A',1)
BtnEnable('B',1)
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
@Start
a=BtnDown('A')
b=BtnDown('B')
if a=0
Goto Start
Wait(100)
End
@Loop
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
c=c-1
Wait(1000)
Goto Loop
Why did we add code to print the counter at the top of our program? Without placing the LcdTextS() line in our code, the screen will be blank if Button A is not pressed and you’ll think something is wrong. Without it, the loop will print the counter counting down when the program starts only after Button A to be pressed, and so nothing will show on the screen. Try removing that line and see for yourself!
We are now ready to check if Button B is pressed to increment the counter starting number by 10. This will happen within the loop where we check if Button A is pressed. To summarize, a loop is checking for a Button A press and within this loop we will check if Button B is pressed to increase the counter. Make sense?
We also want another thing to happen when Button B is pressed. That is, we want the new value to show on the screen every time we increase the counter.
c = 10
BtnEnable('A',1)
BtnEnable('B',1)
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
@Start
a=BtnDown('A')
b=BtnDown('B')
if a=0
if b=1
c=c+10
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
End
Goto Start
End
@Loop
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
c=c-1
Wait(1000)
Goto Loop
Since computers do not mind repeating tasks, we can simplify our code by making it print the counter in every loop, no matter if the counter changed or not. If the counter got increased, then the new value will show on the screen. And if it was not changed, then the old value will show over and over. This may seem redundant, but computers do not mind and the code looks simpler. It is up to you to decide which way is better for you.
c = 10
BtnEnable('A',1)
BtnEnable('B',1)
@Start
a=BtnDown('A')
b=BtnDown('B')
if a=0
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
if b=1
c=c+10
End
Goto Start
End
@Loop
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
c=c-1
Wait(1000)
Goto Loop
Looks like we have a countdown timer, but wait! What happens when the counter reaches zero? Well, we did not check for that and so the loop will continue into negative numbers!
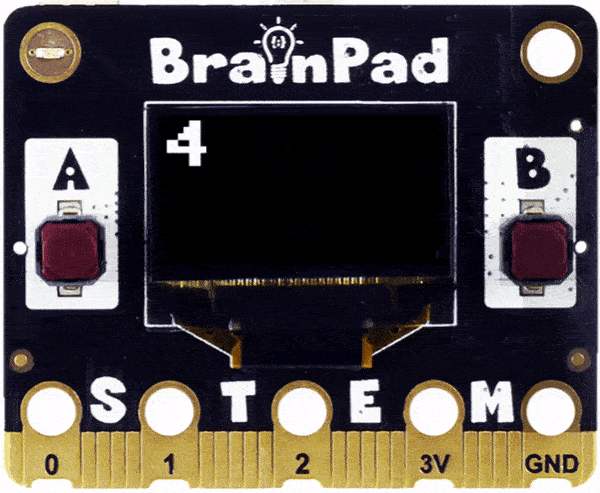
We can check for c=-1 in our @Loop and continue as we wish. In the below example, we will simply goto a @Done subroutine that shows “DONE!” and then exits the program
Do you see this coding style? It is a loop after a loop, and the ending loop takes the program to the next statement/loop. Notice how we positioned “DONE!” to show on the same line as the countdown? Try it without the LcdClear(0) line and see what happens. Not so “clear”, right?
c = 10
BtnEnable('A',1)
BtnEnable('B',1)
@Start
a=BtnDown('A')
b=BtnDown('B')
if a=0
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
if b=1
c=c+10
End
Goto Start
End
@Loop
LcdClear(0)
LcdTextS(Str(c), 1, 4, 4, 4, 4)
LcdShow()
c=c-1
if c=-1
Goto Done
End
Wait(1000)
Goto Loop
@Done
LcdClear(0)
LcdTextS("DONE!", 1, 4, 4, 4, 4)
LcdShow()
Exit
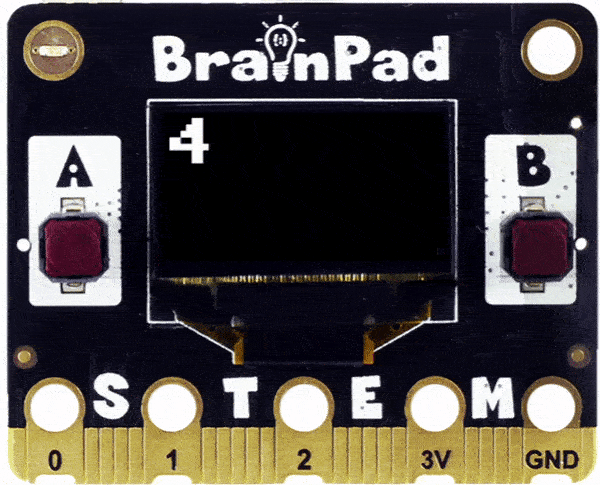
All is good now except we need to do something better than just showing “DONE!”
The Alarm
There are different ways to make an alarm noise, and the easiest way is to sweep through frequencies, from 50 to 5000. Keep the code above the same and add the this to our @Done subroutine, just before Exit
@Done
LcdClear(0)
LcdTextS("DONE!", 1, 4, 4, 4, 4)
LcdShow()
for f=50 to 5000 Step 100
Sound(f,1000,50)
if f>5000
f=50
End
Next
Exit
Using BrainPower, can we also vibrate when making noise? We just need to use DWrite(1,1) to set pin1 high.
@Done
DWrite(1,1)
LcdClear(0)
LcdTextS("DONE!", 1, 4, 4, 4, 4)
LcdShow()
for f=50 to 5000 Step 100
Sound(f,1000,50)
if f>5000
f=50
End
Next
Exit
What’s Next?
Give the screen a “face” and turn it into an Analog Watch.
BrainStorm
On the coding front, what do you think is missing from our countdown timer to be a complete product? How do we terminate the alarm? Can you check for a button press to stop the alarm? How do we restart and reuse the counter? Can we have our program loop back to the very top and repeat the entire code? Hint: Repeat = loop!