Functions
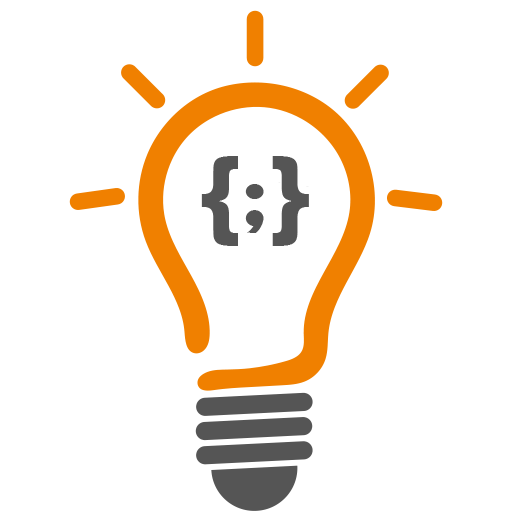
In this lesson we will learn about functions and how to pass arguments and return values. C# and Python both offer functions but differ slightly in how they are created.
Prerequisite
This page assumes you have completed the variables lesson.
Intro
Functions are blocks of code we create that can be used over and over inside our code. Sometimes they are also referred to as methods. Take Sleep() for example; how would you execute the Sleep() command? Would you just sleep in-place or are there steps you would take? The Sleep() function would contain other tasks involved with preparing to sleep, like BrushTeeth(), WashFace(), and PutOnPajamas(). Executing (also referred to as calling) Sleep() inside our program will automatically run the tasks we have put inside.
Subroutines
Functions are not supported in DUE Script. Instead, it uses subroutines. This is similar to BASIC style coding. A subroutine starts with an @ symbol followed by a label name, and ends with a Return.
@Sleep
BrushTeeth()
WashFace()
PutOnPajamas()
Return
Subroutines are function-like but are not functions; however, for the sake of simplifying lessons, we may refer to subroutines as functions.
Invoking Subroutines
To invoke (call) a subroutine, just use its label name with ().
Sleep()
Note that the built in functions in DUE Script are proper functions that take arguments and return values. Users can only create subroutines, which do not take arguments and do not return values. Any of the global variables can be used anywhere in the code, including subroutines.
Let’s make a subroutine/function that converts Fahrenheit to Celsius. We’ll call it FtoC(). The needed formula is Celsius = (Fahrenheit – 32) * 5 / 9.
Note how DUE Script will continue to run the next commands, even thought those after a label. We will be adding Exit command to terminate the program before it reaches the FtoC() subroutine.
f = 72.4
FtoC()
Print(c)
Exit
# Takes f as input - Fahrenheit
# Return results in c - Celsius
@FtoC
c = (f - 32) * 5 / 9
Return
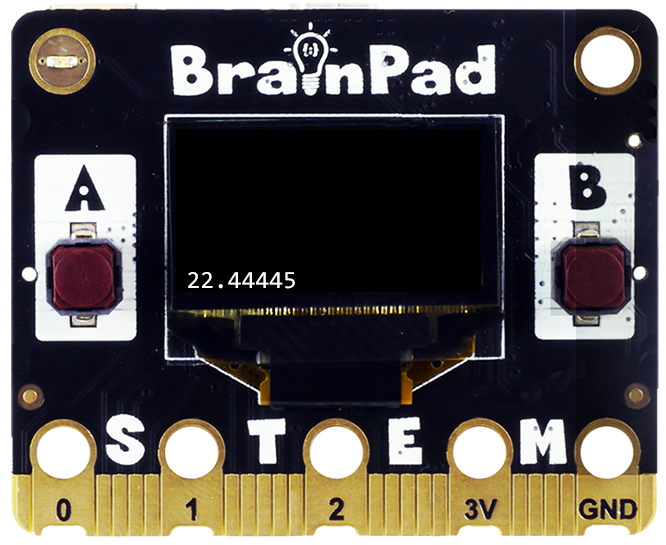
What’s Next?
Trying creating more than one function. Try calling a function from inside another function. From here you can start learning about all of the functions that are built-in functions.
BrainStorm
Can you explain why using functions might be useful when coding? Can you think of other ways to use functions?