Loops
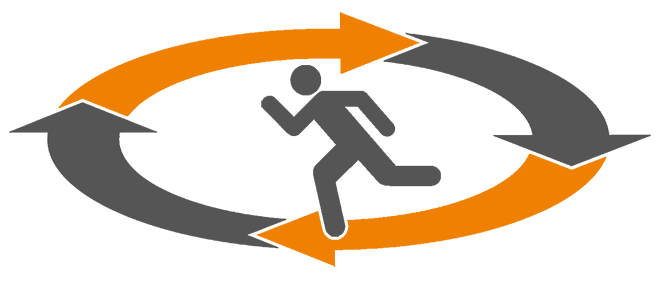
Prerequisite
This page assumes you have completed the variables lesson.
Intro
Loops are handy tools in programming because they allow repetitive tasks to run over and over until a condition is met. That condition can be a count, to loop/run for a specific number of times.
For Loop
A for loop counts through a range. The syntax for the for-loop can be done in two ways. This first way is similar to BASIC.
For i=0 to 4
PrintLn(i)
Next
PrintLn("Loop is over")
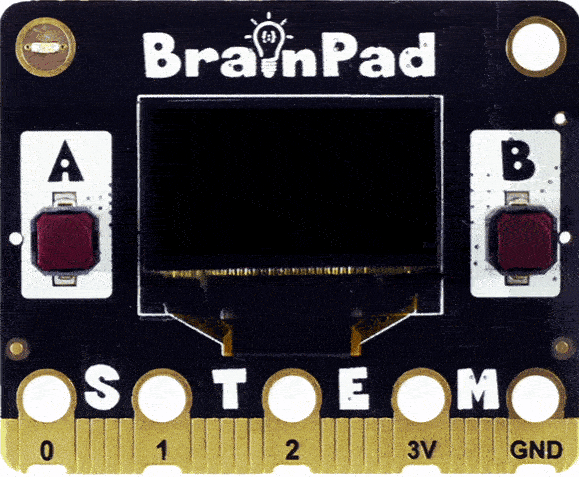
It it is possible to change the increment in each loop from 1 to something else. This code will count 30, 40, 50, 60, 70, 80, 90, 100.
For i=30 to 100 step 10
PrintLn(i)
Next
What if you want to count backwards? This where step should be negative. Note that the range needs to also be from large to small instead small to large.
For i=100 to 30 step -10
PrintLn(i)
Next
For-loops can also be done similarly to Python, using range().
For i in range(0, 4)
PrintLn(i)
Next
The code above looks very similar to the earlier code but it will count 0 to 3 instead of 0 to 4. There is a very important difference between BASIC-Like and Python-Like. The BASIC-like is inclusive of the second value, Python-Like is not.
Step is also possible in range, also similar to python.
For i in range(30, 100, 10)
Println(i)
Next
Goto
Goto statement is used to shift code execution to a predefined label. Labels start with @ symbol and are limited to 6 characters. This code runs endlessly and prints an ever incrementing number.
@Loop
x=x+1
PrintLn(x)
Wait(1000)
Goto Loop
If your program never ends and it cause some system stress, it is possible to create an unresponsive board. Reloading the board with the firmware will wipe everything out and bring the board back to life. For example, removing the Wait() from the code above will because the board to stop responding to the console because it is too busy “printing”!
What’s Next?
Loops are great, but to get the full power of coding we need to check and run code based on a specific condition to handle the program’s Flow Control.
BrainStorm
Loops help computers in repeating tasks. To print 1 to 3, we can just input three lines, but how do we count to 100?
Can you create a loop that prints all even numbers up to 100?
For i= 0 to 100 step 2
PrintLn(i)
Next