Flow Control
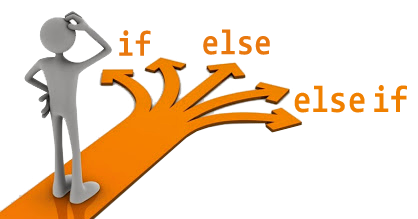
How do programs flow, and how do we control that flow?
Prerequisite
This page assumes you have completed the Coding Intro lesson.
Intro
With the basic flow control mechanisms introduced here, we can decide which path our program will take. Computers aren’t very smart and everything needs to be laid out, including the flow of our program. Can you imagine how complex a flow control could get when you have to think and program for every possible scenario?
If statement
The if statement should be easy to understand. It checks if a condition is true to run a piece of code. For example, we want to print “Too hot” if the temperature is more than 40 degrees (Celsius).
Python
if temperature > 40 :
BrainPad.System.Println("Too hot")
C#
if(temperature > 40){
BrainPad.System.Println("Too hot");
}
JS
if(temperature > 40){
await BrainPad.System.Println("Too hot");
}
The above code examples will not run since we do not have the temperature! But here is an example that we can run. It will print 0 to 9 repeatedly forever because we reset the count back to 0 whenever it reaches 10. Note how we used == instead of =. In coding, = is an assignment and not a check of equality. Use = to assign a value to a variable and == to check if a value is equal to another.
Python
count = 0
while True:
BrainPad.System.Println(count)
count = count + 1
if count == 10:
count = 0
C#
var count = 0;
while(true){
BrainPad.System.Println(count);
count = count + 1;
if(count == 10){
count = 0;
}
}
JS
let count = 0;
while(true){
await BrainPad.System.Println(count);
count = count + 1;
if(count == 10){
count = 0;
}
}
else
The else statement works hand in hand with the if statement. It is there to indicate the opposite of the if statement.
Python
temperature = 47
if temperature > 40 :
BrainPad.System.Println("Too hot")
else:
BrainPad.System.Println("Temp is good")
C#
var temperature = 47;
if(temperature > 40){
BrainPad.System.Println("Too hot");
}
else{
BrainPad.System.Println("Temp is good");
}
JS
var temperature = 47;
if(temperature > 40){
await BrainPad.System.Println("Too hot");
}
else{
await BrainPad.System.Println("Temp is good");
}
You can also have another if statement with else. In Python, this becomes elif.
What output would you expect if you kept the temperature at 47? What if you change it to 5 or 30? Change the temperature variable in the below example to different values and observe the printed text on the screen.
Python
temperature = 47
if temperature > 40:
BrainPad.System.Println("Too hot")
elif temperature < 10:
BrainPad.System.Println("Too Cold")
else:
BrainPad.System.Println("Temp is good")
C#
var temperature = 47;
if(temperature > 40){
BrainPad.System.Println("Too hot");
}
else if(temperature < 10){
BrainPad.System.Println("Too cold");
}
else{
BrainPad.System.Println("Temp is good");
}
JS
var temperature = 47;
if(temperature > 40){
await BrainPad.System.Println("Too hot");
}
else if(temperature < 10){
await BrainPad.System.Println("Too cold");
}
else{
await BrainPad.System.Println("Temp is good");
}
Switch Statement
The switch statement is unavailable in Python and we will not use it in the lessons, but here is an example. The switch statement below uses the variable count. Based on what the value of the count determines which line of code will run. The keyword break is where the code exits the switch statement after executing the section. If count doesn’t equal any of the cases then it will automatically execute the default section of code.
C#
var count = 5;
switch(count){
case 0:
BrainPad.System.Println("Zero");
break;
case 1:
BrainPad.System.Println("One");
break;
case 2:
BrainPad.System.Println("Two");
break;
case 3:
BrainPad.System.Println("Three");
break;
case 4:
BrainPad.System.Println("Four");
break;
case 5:
BrainPad.System.Println("Five");
break;
default:
BrainPad.System.Println("other");
break;
}
JS
let count = 5;
let word;
switch(count){
case 0:
word = "Zero";
break;
case 1:
word = "One";
break;
case 2:
word = "Two";
break;
case 3:
word = "Three";
break;
case 4:
word = "Four";
break;
case 5:
word = "Five";
break;
default:
word = "Zero";
break;
}
await BrainPad.System.Println(word);
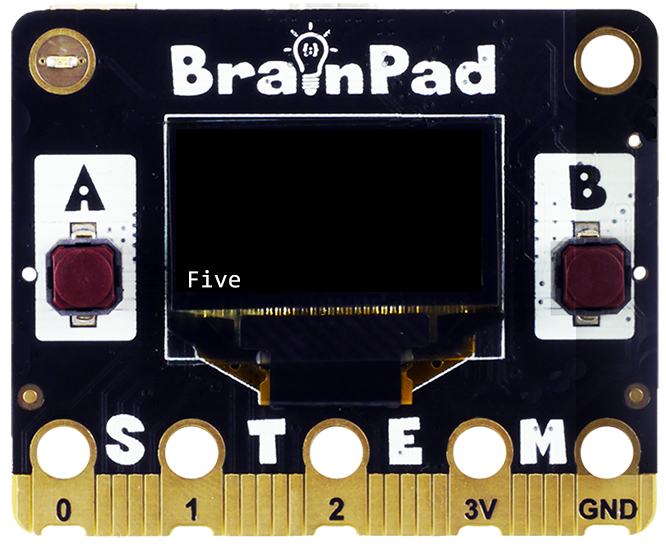
Change the value of the count, run the program, and view the results.
What’s Next?
You are nearly done with the basics of the language, but we still need to learn about operators.
BrainStorm
Is there any difference between humans and computers when it comes to “if”? Computers are very “black and white” with no grey in between. If we program a robot car to cross the intersecting when the traffic light is green, the robot car will do just that without taking in any other variables. Would you cross if there is a person/car in front of you? To us, this is automatic as our brains make millions of decisions around the clock. Teaching a machine to mimic what we do and factor it all in requires very sophisticated software. This is called artificial intelligence. And even then, how do you teach a machine to have feelings?