Variables
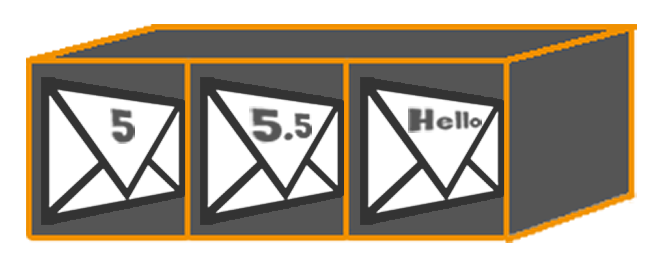
A variable is like a mailbox for computers. It is used to hold a value. That value can be a number (an integer or double) or a word (a string).
Prerequisite
This page assumes you have completed the Coding Intro lesson.
Intro
We can create a variable within C# and JS by using the keyword var, or let in JS. For Python, we don’t need to add a keyword; simply assign a name and that name is now a variable. In this example, we assigned the name myVariable.
Python
myVariable = 5
C#
var myVariable = 5;
JS
let myVariable = 5;
Variable names should be meaningful. You should know what a variable is holding based on the name we give it. This makes the code much easier to read and understand what’s happening inside. We can give the variable any name, but the name we create can’t contain spaces, can’t start with a number, and can’t contain a symbol.
Variable Types
We’re going to discuss 3 different basic types of variables. There are others that you will learn as you get more advanced.
- Integer (int) – An integer is a whole number, like 1, 42, -53, 4834, etc. It can be a positive or negative number but can’t have a decimal point.
- Double (double) – A number with a decimal point, like 0.1, 3.5, 55, -10.2, etc. It can be a positive or negative number and can have a decimal point.
- String (string) – A group of characters. It can be a single word or a long sentence. String variables are always defined by using quotation marks. For example in C#, “This is a string”. Python language also allows for single quotation marks, ‘This is also a string in Python’.
The languages know what kind of variable we’re creating based on value we’re assigning to it.
Python
# creates an integer
number = 10
# creates a double
fraction = 3.5
# creates a string
name = "John"
C#
// creates an integer
var number = 10;
//creates a double
var fraction = 3.5;
//creates a string
var name = "John";
JS
// creates a bool
let number = true;
//creates a number
let fraction = 3.5;
//creates a string
let name = "John";
Some languages, like C#, have a fixed variable type. Once we create the variable it must contain the same type of value for the rest of the program.
// create an integer variable, named x
var x = 10;
// change the value of the variable
x = 83;
// this is wrong and will be an error.
x = "hello";
In fact, C# requires a type for any variable, but using var will let C# pick the most appropriate type for us.
These two lines are technically identical.
var x = 10;
int x = 10;
Python and JS are dynamic and allow to switch types. In the following example, the first line will create a variable named x, since this is the first time we assign a value to x. The variable type will be an integer. The second line will change the variable type to a string and assign “Hello” to it.
Python
x = 10
x = "Hello"
JS
x = 10
x = "Hello"
Arrays
Arrays are a way to store a list of variables that are the same type into one variable name. Let’s create an array of string variables called flowers, and initialize it with values.
Python
flowers = ["Roses", "Violets", "Daisies", "Mums", "Orchids", "Lilies"]
C#
var flowers = {"Roses", "Violets", "Daisies", "Mums", "Orchids", "Lilies"};
JS
let flowers = ["Roses", "Violets", "Daisies", "Mums", "Orchids", "Lilies"];
We can access and assign variables to specific spots in an array. We do this by including a number in brackets after the name, as shown below.
Python
BrainPad.System.Print(flowers[1])
C#
BrainPad.System.Print(flowers[1]);
JS
await BrainPad.System.Print(flowers[1]);
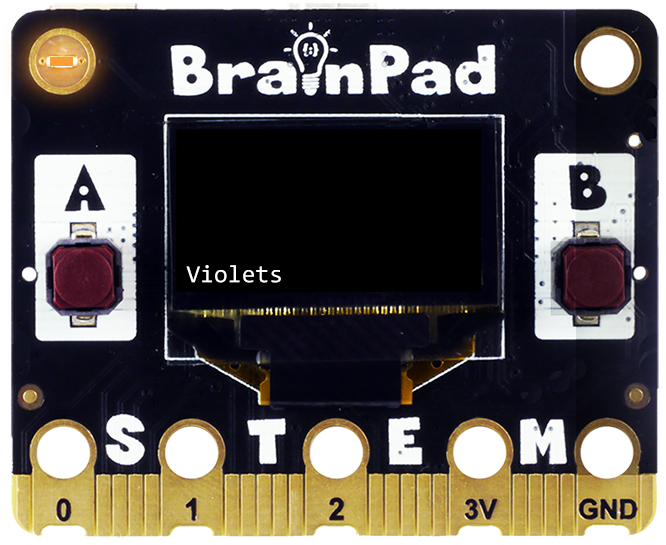
You may wonder why ‘Violets’ appeared instead of ‘Roses’. This is because arrays start counting from zero. To print ‘Roses’, we would have to use flowers[0].
We can easily set a value to a specific spot in an array just like we would set any other variable. It just has to be the same variable type as the array we created.
Python
flowers[3] = "Marigolds"
C#
flowers[3] = "Marigolds";
JS
flowers[3] = "Marigolds";
Now the flowers[3] slot has replaced ‘Mums’ with ‘Marigolds’.
What’s Next?
Learn about Loops and how they are used to repeat tasks.
BrainStorm
We know that anything between double quotes is a string. (Python and JS allow single quotes as well). With that in mind, what is the difference between 53 and “53”? Can you guess what this code will print? Try it!
By the way, the last line differs slightly as C# and Python handle adding to a string differently. When adding a string to a string (“5″+”5”), the result is one string that combines both strings (“55”). However, adding a numerical value to a string (“5” + 4) does not work in the same way between these languages. C# automatically converts the numerical value to a string, such as a 4 becomes “4”. But Python does not do this and will issue an error. We will need to tell it to change the value to a string by adding str before the (numerical value), JavaScript is a dynamically typed language and is not strict about the datatypes of the operand. When the operands are of different types it will convert them into suitable types instead of showing errors
Python
BrainPad.System.Println(53)
BrainPad.System.Println("53")
BrainPad.System.Println(53 + 1)
BrainPad.System.Println("53" + "1")
BrainPad.System.Println("53" + str(1))
C#
BrainPad.System.Print(53);
BrainPad.System.Print("53");
BrainPad.System.Print(53 + 1);
BrainPad.System.Print("53" + "1");
BrainPad.System.Print("53" + 1);
JS
await BrainPad.System.Println(53);
await BrainPad.System.Println("53");
await BrainPad.System.Println(53 + 1);
await BrainPad.System.Println("53" + "1");
await BrainPad.System.Println("53" + 1);