Operators
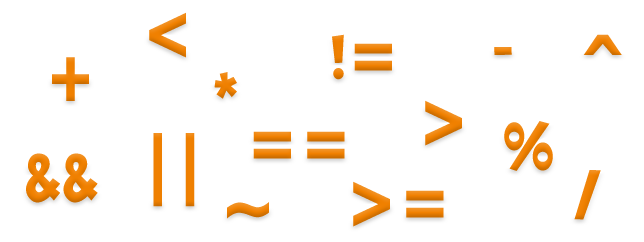
Operators are needed to check the relationship between two values.
Prerequisite
This page assumes you have completed the variables lesson.
Intro
This lesson will bring you closer to understanding coding and how the use of operators really depends on what you need the code to do for your program. Not only that, the use of operators in multiple conditions can really bring a program to life!
Assignment
The = operator is used for assignments. It takes whatever is on the right side and assign its value to the left side.
x = 50
y = 43 + x
Equality
The = operator is also used to check if two sides are equal. In advanced languages, == is used for equality but DUE Script keeps things simple. DUE Script know that in an if statement, you are checking for equality and not assigning a value to a variable
If x = 50 # This is checking for equality and not an assignment
End
To check for not equal, we use != operator.
If x != 50
Print("X is not 50")
End
Greater or Less
The greater-than > and less-than < symbols compare two values to see which one is more or less. In this example, setting x to 50 will not print anything. That is because 50 is not less than 50!
If x > 50
Print("X is more than 50")
End
To check if the value is greater-than or equal-to, use >= operator. Similarly, you can use <= to check if a value is less-than or equal-to. In this example, setting x to 50 will print the message.
If x >= 50
Print("X is more than or equal 50")
End
Math
These work exactly how you would expect. 5+5 will result in 10.
Operator | Name |
---|---|
+ | Add |
– | Subtract |
* | Multiply |
/ | Divide |
% | Modulus, the remainder of a division. |
Logical
Logical operators are useful to check multiple evaluations. For example, you can check if x is more than 10 AND is also less than 40 using &&.
If x > 50 && x < 90
Print("between 50 and 90")
End
We recommend using parathesis to make the code clearer.
If (x > 50) && (x < 90)
Print("between 50 and 90")
End
The opposite of and operator is the or operator. In DUE Script the logical or operator is || (double pipe symbol) and in Python it is or. Can you guess how to make the || symbol using your keyboard?
Do not confuse the pipe symbol | with lower case L and upper case I. The key we need is found all the way to the right above to the Enter key, and it includes the the backslash \ symbol. Just like any other key containing two characters where the one you want is the top character, use shift and hit this key twice to get the double pipe.
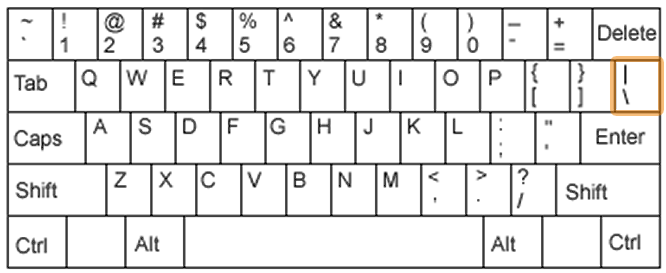
If (x < 50) || (x > 90)
Print("less than 50 or more than 90")
End
Bitwise
These operators handle values on bit level. This is beyond what we need in these lessons and we will not cover them.
Operator | Name |
---|---|
& | AND |
| | OR |
^ | XOR |
~ | NOT |
>> | Right shift |
<< | Left shift |
What’s Next?
You should have a nearly complete idea of the core features of coding. The only thing left is to start creating functions to encapsulate code blocks.
BrainStorm
You can use nested if statements to check multiple conditions. If we can “nest” an if statement inside another if statement, why use the and operator? There are multiple ways of writing the same code. While the BrainPad/computer may behave the same, there are ways to write code that is easier to read and understand.