Weather Forecast with BrainSense
Weather forecasting employs advanced technologies to predict future atmospheric conditions. Scientists use data from satellites, radars, and weather stations to analyze temperature, humidity, wind speed, and pressure. Computer models then simulate the complex interactions of these factors, providing us with accurate and timely forecasts, and guiding our day-to-day plans.
In this lesson, we will learn how to compare our room’s temperature and humidity using the BrainSense kit sensor and the city weather using weather API.
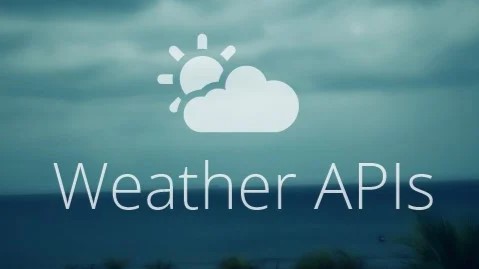
Prerequisite
Basic Python coding, completion of BrainSense modules (focus on temperature and humidity sensor section), and familiarity with using APIs in IoT, you can look at previous IoT lessons like stock tracker.
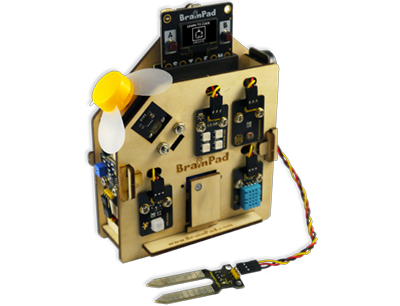
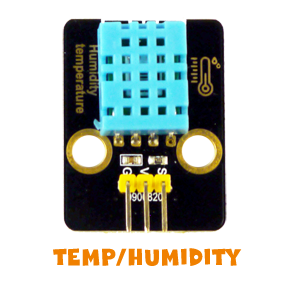
The API
Our demo program uses FREE weather forecasting API from open-meteo.com. The free service allows up to daily requests below 10,000 for non-commercial use.
The returned data is in JSON format. In our example, referring to the API docs, we can customize our request based on our requirements. We can specify the time and location by providing latitude and longitude or by typing the city name in the search bar, in this example we choose the location of Detroit, MI, USA.
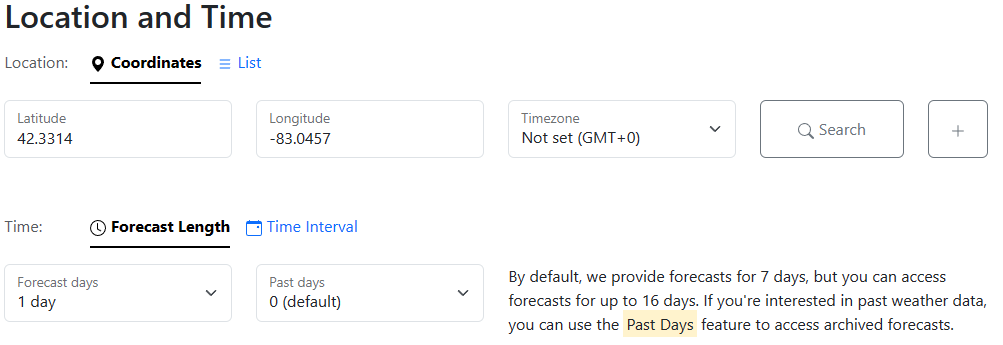
Next, we select whether we want hourly, daily, or current weather variables, and we must define our preferred units by accessing the settings section.
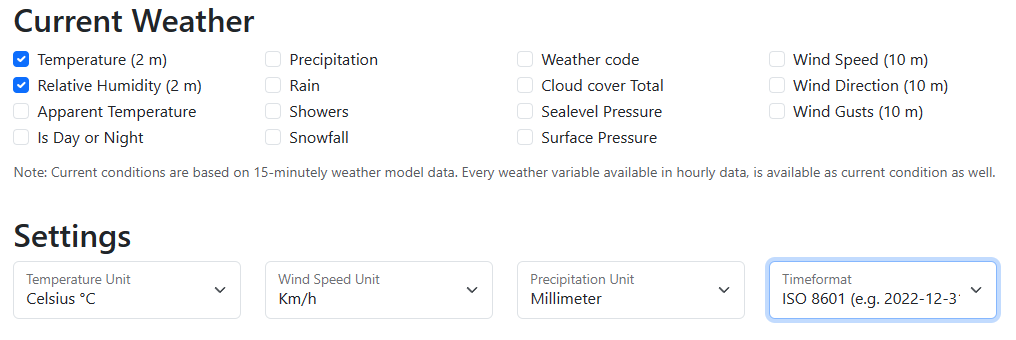
Lastly, in the API Response section, we can utilize our preferred programming language (in our case, Python) and copy the generated code to correctly implement the API, here is the final JSON return object.
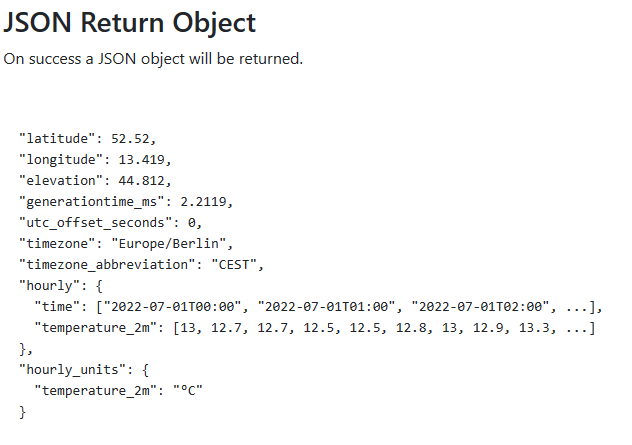
The Implementation
Ensure you have Python 3.10 installed on your computer. Additionally, install the required libraries using the following commands:
pip install openmeteo-requests
pip install requests-cache retry-requests numpy pandas
pip install DUELink
The following code showcases the right use of the API with Python language.
import time
from datetime import datetime
import openmeteo_requests
import requests_cache
from retry_requests import retry
# Make sure all required weather variables are listed here
# The order of variables in hourly or daily is important to assign them correctly below
url = "https://api.open-meteo.com/v1/forecast"
params = {
"latitude": 42.3314,
"longitude": -83.0457,
"current": ["temperature_2m", "relative_humidity_2m"],
"timezone": "auto",
"forecast_days": 1
}
while True:
# Set up the Open-Meteo API client with cache and retry on error
cache_session = requests_cache.CachedSession('.cache', expire_after=3600)
retry_session = retry(cache_session, retries=5, backoff_factor=0.2)
openmeteo = openmeteo_requests.Client(session=retry_session)
responses = openmeteo.weather_api(url, params=params)
# Process first location. Add a for-loop for multiple locations or weather models
response = responses[0]
print("_______________________________________________________________________")
print(f"Coordinates: {response.Latitude()}°E {response.Longitude()}°N")
print(f"Elevation: {response.Elevation()} m asl")
print(f"Timezone: {response.Timezone()} {response.TimezoneAbbreviation()}")
print(f"Timezone difference to GMT+0: {response.UtcOffsetSeconds()} s")
# Current values. The order of variables needs to be the same as requested.
current = response.Current()
current_temperature_2m = current.Variables(0).Value()
# Round float numbers to two decimal places.
current_temperature_2m = float("{:.2f}".format(current_temperature_2m))
current_relative_humidity_2m = current.Variables(1).Value()
# Convert Unix timestamp to datetime
formatted_time = datetime.utcfromtimestamp(current.Time()).strftime('%Y-%m-%d %H:%M:%S UTC')
print(f"Current time: {formatted_time}")
print(f"Current tempera ture_2m= {current_temperature_2m}")
print(f"Current relative_humidity_2m= {current_relative_humidity_2m}")
time.sleep(3)
The following code is to get the temperature and humidity value from the sensor and a method to display all the data of room and city temperature and humidity on the BrainPad Pulse LCD screen.
import time
from DUELink.DUELinkController import DUELinkController
available_port = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(available_port)
temp_humid_pin = 6
def pulse_lcd(sensor_temp, sensor_humidity, current_temperature, current_relative_humidity):
BrainPad.Display.Clear(0)
# BrainPad.Display.DrawTextScale("BrainSense", 1, 3, 0, 2, 1)
y = 0
x = 5
BrainPad.Display.DrawText("Room", 1, 55, y)
BrainPad.Display.DrawText(f"Temperature= {sensor_temp}", 1, x, y + 10)
BrainPad.Display.DrawText(f"Humidity= {sensor_humidity}", 1, x, y + 20)
BrainPad.Display.DrawText("City", 1, 55, y + 30)
BrainPad.Display.DrawText(f"Temperature= {current_temperature}", 1, x, y + 40)
BrainPad.Display.DrawText(f"Humidity= {current_relative_humidity}", 1, x, y + 50)
BrainPad.Display.Show()
while True:
# Get the temperature and humidity value from assigned sensor
temperature_sensor_value = BrainPad.Temperature.Read(temp_humid_pin, 11)
humidity_sensor_value = BrainPad.Humidity.Read(temp_humid_pin, 11)
print("__________________________________")
print(f"############ Room ############\nTemp= {temperature_sensor_value} | Humidity= {humidity_sensor_value}")
# Call the method responsible for printing all data on the BrainPad Pulse screen
#pulse_lcd(temperature_sensor_value, humidity_sensor_value, current_temperature_2m, current_relative_humidity_2m)
time.sleep(3)
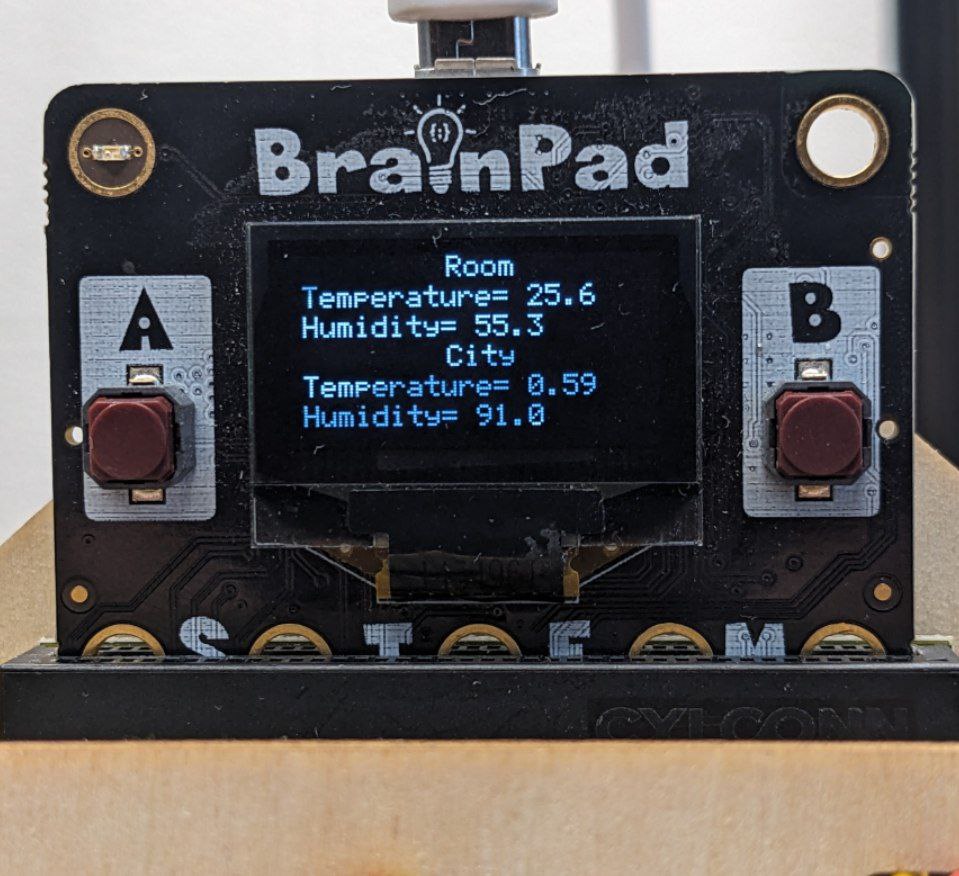
Complete Code
import time
from datetime import datetime
import openmeteo_requests
import requests_cache
from retry_requests import retry
from DUELink.DUELinkController import DUELinkController
available_port = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(available_port)
temp_humid_pin = 6
def pulse_lcd(sensor_temp, sensor_humidity, current_temperature, current_relative_humidity):
BrainPad.Display.Clear(0)
# BrainPad.Display.DrawTextScale("BrainSense", 1, 3, 0, 2, 1)
y = 0
x = 5
BrainPad.Display.DrawText("Room", 1, 55, y)
BrainPad.Display.DrawText(f"Temperature= {sensor_temp}", 1, x, y + 10)
BrainPad.Display.DrawText(f"Humidity= {sensor_humidity}", 1, x, y + 20)
BrainPad.Display.DrawText("City", 1, 55, y + 30)
BrainPad.Display.DrawText(f"Temperature= {current_temperature}", 1, x, y + 40)
BrainPad.Display.DrawText(f"Humidity= {current_relative_humidity}", 1, x, y + 50)
BrainPad.Display.Show()
# Make sure all required weather variables are listed here
# The order of variables in hourly or daily is important to assign them correctly below
url = "https://api.open-meteo.com/v1/forecast"
params = {
"latitude": 42.3314,
"longitude": -83.0457,
"current": ["temperature_2m", "relative_humidity_2m"],
"timezone": "auto",
"forecast_days": 1
}
while True:
# Set up the Open-Meteo API client with cache and retry on error
cache_session = requests_cache.CachedSession('.cache', expire_after=3600)
retry_session = retry(cache_session, retries=5, backoff_factor=0.2)
openmeteo = openmeteo_requests.Client(session=retry_session)
responses = openmeteo.weather_api(url, params=params)
# Process first location. Add a for-loop for multiple locations or weather models
response = responses[0]
print("_______________________________________________________________________")
print(f"Coordinates: {response.Latitude()}°E {response.Longitude()}°N")
print(f"Elevation: {response.Elevation()} m asl")
print(f"Timezone: {response.Timezone()} {response.TimezoneAbbreviation()}")
print(f"Timezone difference to GMT+0: {response.UtcOffsetSeconds()} s")
# Current values. The order of variables needs to be the same as requested.
current = response.Current()
current_temperature_2m = current.Variables(0).Value()
# Round float numbers to two decimal places.
current_temperature_2m = float("{:.2f}".format(current_temperature_2m))
current_relative_humidity_2m = current.Variables(1).Value()
# Convert Unix timestamp to datetime
formatted_time = datetime.utcfromtimestamp(current.Time()).strftime('%Y-%m-%d %H:%M:%S UTC')
print(f"Current time: {formatted_time}")
print(f"Current tempera ture_2m= {current_temperature_2m}°C")
print(f"Current relative_humidity_2m= {current_relative_humidity_2m}")
# Get the temperature and humidity value from assigned sensor
temperature_sensor_value = BrainPad.Temperature.Read(temp_humid_pin, 11)
humidity_sensor_value = BrainPad.Humidity.Read(temp_humid_pin, 11)
print("__________________________________")
print(f"############ Room ############\nTemp= {temperature_sensor_value}°C | Humidity= {humidity_sensor_value}")
print(f"############ City ############\nTemp= {current_temperature_2m}°C | Humidity= {current_relative_humidity_2m}")
# Call the method responsible for printing all data on the BrainPad Pulse screen
pulse_lcd(temperature_sensor_value, humidity_sensor_value, current_temperature_2m, current_relative_humidity_2m)
time.sleep(3)
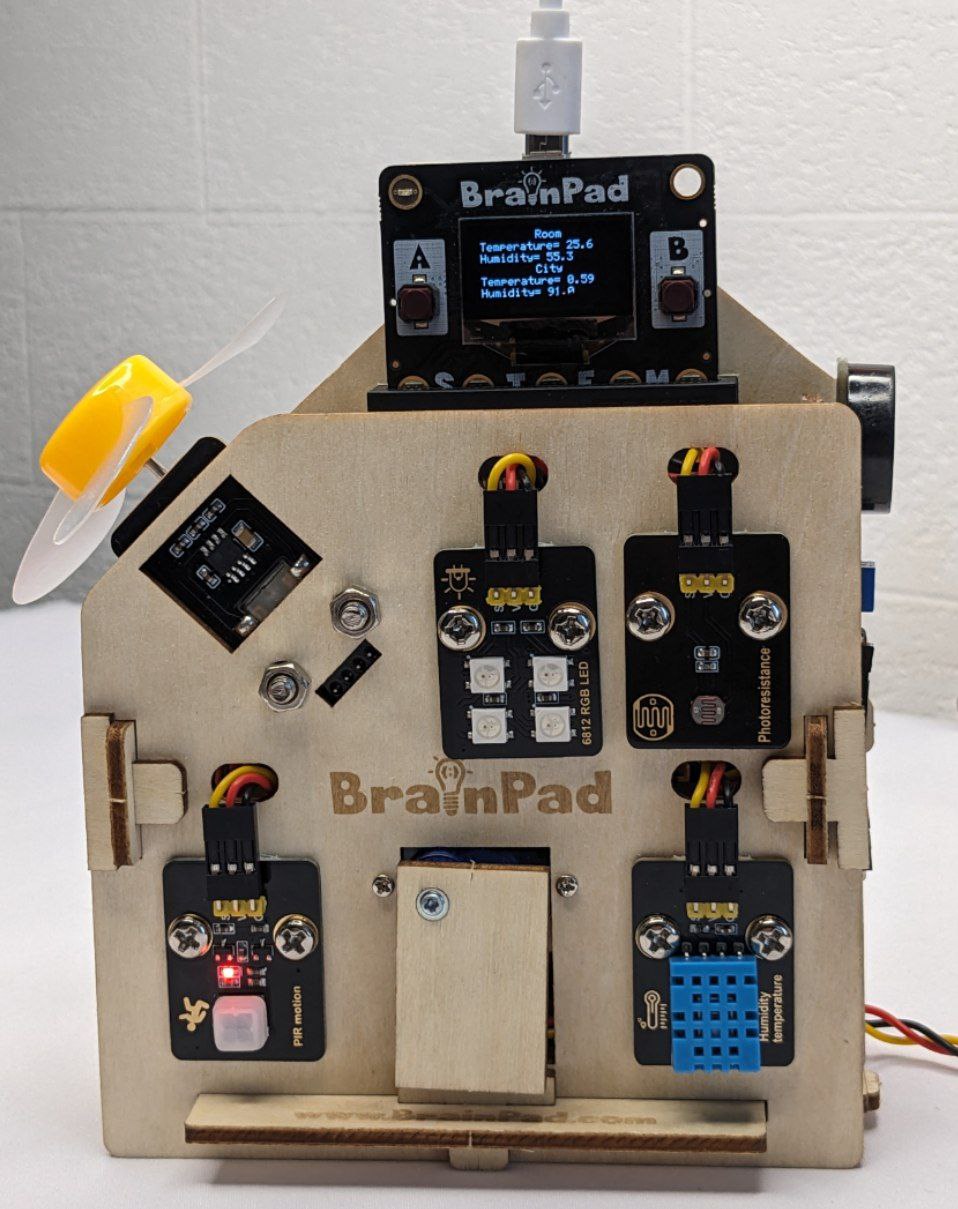
What’s Next?
Can you figure out other IoT applications we can do with Python and the BrainSense kit? Jump back to see all BrainSense kit modules.
BrainStorm
The integration of hardware and IoT not only amplifies smart home capabilities but also paves the way for sustainable, eco-friendly solutions, revolutionizing living spaces and promoting environmental well-being, can you imagine more interesting sustainable IoT applications?