Smart house lighting
Smart house lighting with IoT enables seamless control and efficiency, allowing users to manage house lights remotely for enhanced energy conservation and convenience.
In this lesson, we will manage the external lighting of our home, controlling its activation and deactivation under daily sunrise and sunset times. This will be achieved through the utilization of an API and prototyping our project on the BrainSense kit.

Prerequisite
You must have a basic understanding of coding with Python, have completed the Neo lesson, and completed BrainSense modules (focus on RGB LEDs section), and have familiarity with using APIs in IoT, you can look at previous IoT lessons like Weather Forecast.
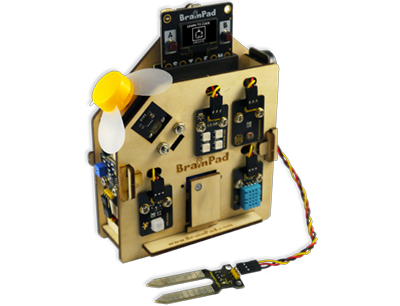
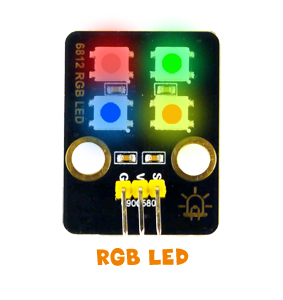
The API
Our demo program uses FREE SunriseSunset.io API, perfect for displaying sunset and sunrise times in our applications or websites using JSON. Currently, they serve millions of requests a month to developers and their apps.
The returned data is in JSON format. In our example, referring to the API docs, we can 3 types of requests based on our requirements. We will use the standard one and specify the location by providing latitude and longitude, In this example, we choose the location of Madison Heights, MI, USA or you can choose any location by Google Maps and get the latitude and longitude of it.
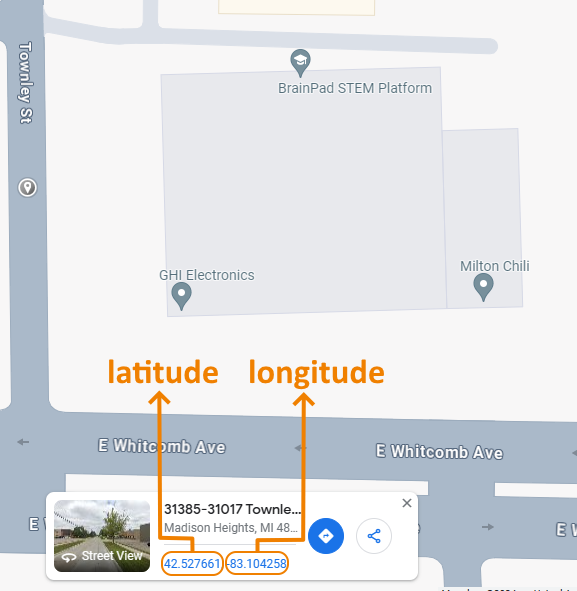
Here is the final JSON return object.
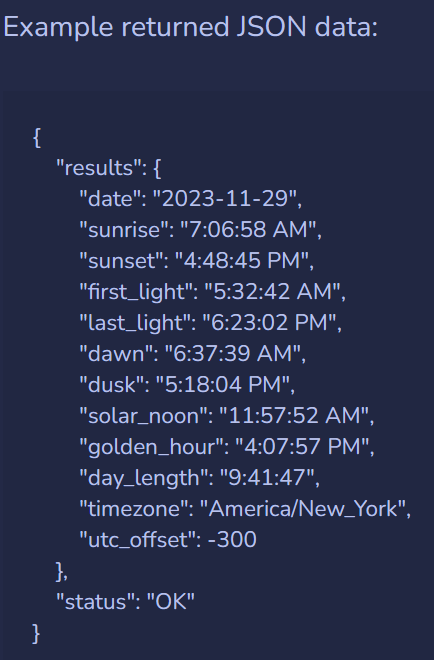
The Implementation
Ensure you have Python 3.10 installed on your computer. Additionally, install the required libraries using the following commands:
pip install urllib3
pip install DUELink
The following code showcases the right use of the API with Python language and prints the results.
# Import required libraries
import urllib3
import json
from datetime import datetime
from DUELink.DUELinkController import DUELinkController
# Get the available port for DUELink controller and initialize the controller
availablePort = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(availablePort)
# Set the latitude and longitude for the location
# In this example we chose Madison Heights, MI, USA
latitude = 42.527661
longitude = -83.104258
# Define the pin for the LED
led_pin = 12
# Create a connection pool for HTTP requests
http = urllib3.PoolManager()
# Fetch sunrise and sunset times from the SunRiseSunSet API based on the given latitude and longitude
response_sunrise_today = http.request('GET', f"https://api.sunrisesunset.io/json?lat={latitude}&lng={longitude}")
sun_time_today = json.loads(response_sunrise_today.data)
# Print the fetched sunrise and sunset times
for key, value in sun_time_today.items():
if isinstance(value, dict):
print(f"\n{key}:")
for sub_key, sub_value in value.items():
print(f" {sub_key}: {sub_value}")
else:
print(f"{key}: {value}")
print(f"______________________________________")
Following this step, it is imperative to store both the sunrise and sunset times along with the date, and subsequently, convert their data types into DateTime objects for seamless comparison between the respective time values.
# Extract relevant data from the API response
today_date = sun_time_today["results"]["date"]
sunrise_value_today = sun_time_today["results"]["sunrise"]
sunset_value_today = sun_time_today["results"]["sunset"]
# Convert sunrise and sunset times to datetime objects
sunrise_value_today = datetime.strptime(today_date + " " + sunrise_value_today, "%Y-%m-%d %I:%M:%S %p")
sunset_value_today = datetime.strptime(today_date + " " + sunset_value_today, "%Y-%m-%d %I:%M:%S %p")
Finally, we will get the current computer time using the DateTime library and do a comparison with the sunrise and sunset times. This will enable the automated control of lights, ensuring they are turned off during this specified timeframe.
# Run an infinite loop to continuously monitor and control the lighting
while True:
# Clear the display and LED strip on the BrainPad
BrainPad.Display.Clear(0)
BrainPad.Neo.Clear()
# Get the current datetime
current_datetime = datetime.now()
# Print the current time, sunrise time, and sunset time for debugging
print(f"Current Time ={current_datetime}")
print(f"Sunrise Time ={sunrise_value_today}")
print(f"Sunset Time ={sunset_value_today}")
print(f"______________________________________")
# Check if the current time is within the sunrise and sunset window
if sunrise_value_today <= current_datetime <= sunset_value_today:
# If within the window, set LED colors to off (black)
colors = [0x000000] * 4
BrainPad.Neo.SetMultiple(led_pin, colors)
else:
# If outside the window, set LED colors to blue
colors = [0x0000FF] * 4
BrainPad.Neo.SetMultiple(led_pin, colors)
# Wait for 1 second before the next iteration
BrainPad.System.Wait(1000)
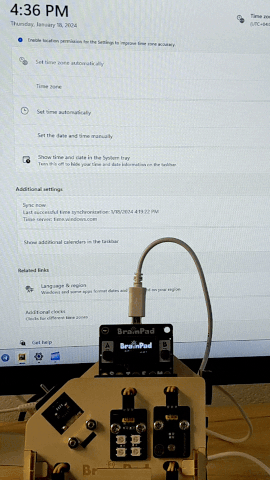
Complete Code
# Import required libraries
import urllib3
import json
from datetime import datetime
from DUELink.DUELinkController import DUELinkController
# Get the available port for DUELink controller and initialize the controller
availablePort = DUELinkController.GetConnectionPort()
BrainPad = DUELinkController(availablePort)
# Set the latitude and longitude for the location
# In this example we chose Madison Heights, MI, USA
latitude = 42.527661
longitude = -83.104258
# Define the pin for the LED
led_pin = 12
# Create a connection pool for HTTP requests
http = urllib3.PoolManager()
# Fetch sunrise and sunset times from the SunRiseSunSet API based on the given latitude and longitude
response_sunrise_today = http.request('GET', f"https://api.sunrisesunset.io/json?lat={latitude}&lng={longitude}")
sun_time_today = json.loads(response_sunrise_today.data)
# Print the fetched sunrise and sunset times
for key, value in sun_time_today.items():
if isinstance(value, dict):
print(f"\n{key}:")
for sub_key, sub_value in value.items():
print(f" {sub_key}: {sub_value}")
else:
print(f"{key}: {value}")
print(f"______________________________________")
# Extract relevant data from the API response
today_date = sun_time_today["results"]["date"]
sunrise_value_today = sun_time_today["results"]["sunrise"]
sunset_value_today = sun_time_today["results"]["sunset"]
# Convert sunrise and sunset times to datetime objects
sunrise_value_today = datetime.strptime(today_date + " " + sunrise_value_today, "%Y-%m-%d %I:%M:%S %p")
sunset_value_today = datetime.strptime(today_date + " " + sunset_value_today, "%Y-%m-%d %I:%M:%S %p")
# Run an infinite loop to continuously monitor and control the lighting
while True:
# Clear the display and LED strip on the BrainPad
BrainPad.Display.Clear(0)
BrainPad.Neo.Clear()
# Get the current datetime
current_datetime = datetime.now()
# Print the current time, sunrise time, and sunset time for debugging
print(f"Current Time ={current_datetime}")
print(f"Sunrise Time ={sunrise_value_today}")
print(f"Sunset Time ={sunset_value_today}")
print(f"______________________________________")
# Check if the current time is within the sunrise and sunset window
if sunrise_value_today <= current_datetime <= sunset_value_today:
# If within the window, set LED colors to off (black)
colors = [0x000000] * 4
BrainPad.Neo.SetMultiple(led_pin, colors)
else:
# If outside the window, set LED colors to blue
colors = [0xF0F0F0] * 4
BrainPad.Neo.SetMultiple(led_pin, colors)
# Wait for 1 second before the next iteration
BrainPad.System.Wait(1000)
What’s Next?
Can you figure out other IoT applications we can do with Python and the BrainSense kit? Jump back to see all BrainSense kit modules.
BrainStorm
The integration of hardware and IoT not only amplifies smart home capabilities but also paves the way for sustainable, eco-friendly solutions, revolutionizing living spaces and promoting environmental well-being, can you imagine more interesting sustainable IoT applications?